He's a little application I wrote today. It's a price ticker you can use on your website. You can see it live at https://steemian.info/ticker. Maybe I'll embed it on all the pages later. It would be nice to have something similar added in Condenser (Steemit's web interface).
I was writing it at first in PHP, but then I figured why not make it non-PHP, for the fun of it.
The code relies on API calls to Coinmarketcap and Steemit's new API node. The prices update every 60 seconds; you can change that by editing the setInterval()
function.
Cryptocurrency prices are queries from Coinmarketcap. The SBD price feed and the MVest value are taken and calculated from Steemit. Enjoy!
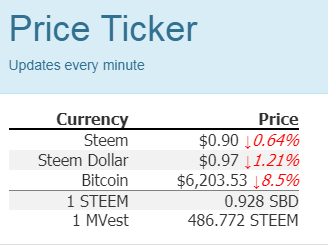
Specifications: HTML5, JQuery, CSS.
Code available on
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<style>
tr td {text-align: right;}
table {padding: 5px;}
table.striped tr:nth-child(even){background-color: #f2f2f2;}
thead {border-bottom: 2px solid black; font-weight: bold;}
</style>
</head>
<body>
<div class="container">
<div class="panel panel-info">
<div class="panel-heading"><h1>Price Ticker</h1><h5>Updates every minute</h5></div>
<div class="panel-body">
<table class="striped">
<thead>
<tr>
<td width="120">Currency</td>
<td width="170">Price</td>
</tr>
</thead>
<tbody>
<tr>
<td >Steem</td>
<td class="blink" id="steem"></td>
</tr>
<tr>
<td>Steem Dollar</td>
<td class="blink" id="sbd"></td>
</tr>
<tr style="border-bottom: 1px solid grey;">
<td>Bitcoin</td>
<td class="blink" id="btc"></td>
</tr>
<tr>
<td>1 STEEM</td>
<td class="blink" id="feed_price"></td>
</tr>
<tr>
<td>1 MVest</td>
<td class="blink" id="steem_per_mvests"></td>
</tr>
</tbody>
</table>
<script>
$.ajaxSetup({async: false}); // must set to synchronous to be able to set the global variables sbd, steem, etc...
function getPrices() {
url = 'https://api.coinmarketcap.com/v1/ticker/steem/?convert=USD';
$.getJSON(url, function (data) {
steem = parseFloat(data[0].price_usd).toFixed(2); // convert the string to number and round to 2 decimals
steemchange = parseFloat(data[0].percent_change_24h).toFixed(2);
});
url = 'https://api.coinmarketcap.com/v1/ticker/steem-dollars/?convert=USD';
$.getJSON(url, function (data) {
sbd = parseFloat(data[0].price_usd).toFixed(2);
sbdchange = parseFloat(data[0].percent_change_24h).toFixed(2);
});
url = 'https://api.coinmarketcap.com/v1/ticker/bitcoin/?convert=USD';
$.getJSON(url, function (data) {
btc = parseFloat(data[0].price_usd).toFixed(2);
btcchange = parseFloat(data[0].percent_change_24h).toFixed(2);
});
url = "https://api.steemit.com";
$.ajax({
type: "POST",
url: url,
dataType: "json",
data: JSON.stringify({"id": "0", "jsonrpc": "2.0", "method": "get_state", "params": ["total_vesting_shares"]}),
success: function (response) {
if (response) {
total_vesting_shares = response.result.props.total_vesting_shares;
total_vesting_fund_steem = response.result.props.total_vesting_fund_steem;
feed_price = response.result.feed_price.base;
ret = total_vesting_shares.split(" ");
total_vesting_shares = ret[0];
ret = total_vesting_fund_steem.split(" ");
total_vesting_fund_steem = ret[0];
steem_per_mvests = (total_vesting_fund_steem / total_vesting_shares * 1000000).toFixed(3); // round to 3 decimals
}
}
});
$("#btc").html("$" + btc.replace(/\d(?=(?:\d{3})+\.)/g, '$&,') + " " + (btcchange > 0 ? '<i style="color:green;">\u2191' + Math.abs(btcchange) + '%</i>' : '<i style="color:red;">\u2193' + Math.abs(btcchange) + '%</i>'));
$("#sbd").html("$" + sbd.replace(/\d(?=(?:\d{3})+\.)/g, '$&,') + " " + (sbdchange > 0 ? '<i style="color:green;">\u2191' + Math.abs(sbdchange) + '%</i>' : '<i style="color:red;">\u2193' + Math.abs(sbdchange) + '%</i>'));
$("#steem").html("$" + steem.replace(/\d(?=(?:\d{3})+\.)/g, '$&,') + " " + (steemchange > 0 ? '<i style="color:green;">\u2191' + Math.abs(steemchange) + '%</i>' : '<i style="color:red;">\u2193' + Math.abs(steemchange) + '%</i>'));
$("#steem_per_mvests").html(steem_per_mvests + " STEEM");
$("#feed_price").html(feed_price);
}
getPrices(); // get prices
setInterval(function () {
getPrices();
$(".blink").fadeOut("slow").fadeIn();
}, 60000); // then get prices every 60 seconds
</script>
</div>
</div>
</div>
</body>
</html>

Available & Reliable. I am your Witness. I want to represent You.
🗳 If you like what I do, consider voting for me 🗳
If you never voted before, I wrote a detailed guide about Voting for Witnesses.
Go to https://steemit.com/~witnesses. My name is listed in the Top 50. Click the upvote symbol.
Alternatively,
with cli_wallet : vote_for_witness "YOURACCOUNT" "drakos" true true
with steem-python: steempy approvewitness drakos --account YOURACCOUNT
That's a great effort. Keep it up
Thanks :)
thank yhuvery much sir for yhur services
This is sweet! Will use this and add my local currency. Thanks! HOOT!
Cool. Just follow the code, it's pretty easy to add your favorite currencies. Copy/paste here and there, done 🤓
Thanks for sharing, would like to build my own price checker too.
Nice work @drakos!
Yes ajax definitely makes it easier to embed anywhere, and coinmarket guys have a solid API.
The ajax call to steemit's api was a pain though. I was stuck on it, wasn't working with
getJSON
. Then I redid it with good old ajax and setting the type to POST (after recommendation by @netuoso), but didn't work neither. I tinkered with it until I discovered it neededJSON.stringify
! The rest is history.I hear you, that's the fun with programming :D
Yet it is highly rewarding once you finally get things working !