To improve your witness latencies, it's important to have the best seed pings in your config.ini.
Usually, you can go to https://status.steemnodes.com/, ping all the online servers, select the ones with the lowest latencies, and add them to the witness config. It's tedious if you want to do that manually!
I wrote a little bash script to automate the pinging process. Here are the steps:
Go to https://status.steemnodes.com/ and click the "config.ini format" tab, this will give you the list of the online seeds.
Copy all the lines into a text file called seeds.txt, for example:
seed-node = node.mahdiyari.info:2001
seed-node = seed.roelandp.nl:2001
seed-node = 104.199.118.92:2001
seed-node = seed.followbtcnews.com:2001
seed-node = seed.thecryptodrive.com:2001
seed-node = steem-seed1.abit-more.com:2001
seed-node = seed.steemnodes.com:2001
Create a new file called "pings.sh" and paste the following into it:
#!/usr/bin/env bash
if [ -f seeds.txt ]; then
let offlinecount=0
let onlinecount=0
rm results.txt
while read seed; do
host=`echo $seed | awk '{print $3}' | cut -d ':' -f 1`
pinged=`ping -c 1 -W 1 $host | tail -1 | awk '{print $4}' | cut -d '/' -f 2`
echo "$seed $pinged"
if [ ! -z $pinged ]; then
echo "$pinged $seed">> results.txt
let "onlinecount++"
else
let "offlinecount++"
fi
done <seeds.txt
echo "$onlinecount seeds are online"
echo "$offlinecount seeds are offline"
sort -n results.txt > pings-sorted.txt
cut -d " " -f2- pings-sorted.txt > pings-finalresults.txt
else
echo "Missing seeds.txt file"
fi
Give the pings.sh execute permissions:
chmod +x pings.sh
Run the script.
./pings.sh
Check the pings-sorted.txt
for the lowest pings (e.g. <100ms), and copy/paste the corresponding lines from pings-finalresults.txt
into your witness config.ini
.
Note: Some of the seeds may be listed as being Online, but they can be down; @wackou can you check that please? Those seeds will be discarded by the script in the final results.
Restart your steemd or dockerized image.
ADDENDUM
paping, a cross-platform TCP port testing tool. It emulates the functionality of ping (port ping).
https://code.google.com/archive/p/paping/As pointed out by @wackou, some servers may have their ICMP firewalled, so the pinging script would yield false negatives. I found
Download paping
wget https://storage.googleapis.com/google-code-archive-downloads/v2/code.google.com/paping/paping_1.5.5_x86-64_linux.tar.gz
Decompress it
tar xzvf paping_1.5.5_x86-64_linux.tar.gz
And use this script instead of the previous one:
#!/usr/bin/env bash
if [ -f seeds.txt ]; then
let offlinecount=0
let onlinecount=0
rm results.txt
while read seed; do
host=`echo $seed | awk '{print $3}' | cut -d ':' -f 1`
port=`echo $seed | awk '{print $3}' | cut -d ':' -f 2`
pinged=`./paping $host -p $port -c 1 --nocolor| grep 'Average' | cut -d '=' -f 4 | cut -d ' ' -f 2`
echo "$host $pinged"
if [ $pinged != '0.00ms' ]; then
echo "$pinged $seed">> results.txt
let "onlinecount++"
else
let "offlinecount++"
fi
done <seeds.txt
echo "$onlinecount seeds are online"
echo "$offlinecount seeds are offline"
sort -n results.txt > pings-sorted.txt
cut -d " " -f2- pings-sorted.txt > pings-finalresults.txt
else
echo "Missing seeds.txt file"
fi
Proud member and delegator of the @minnowsupport project. Join us on https://discord.gg/GpHEEhV
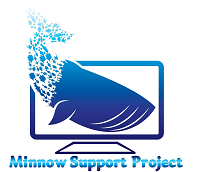
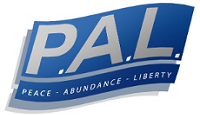

π³ Please check my Steem witness application and consider voting for me. π³
Thank you for your support.
Alternatively, you can issue this command in cli_wallet
(after unlocking it)
vote_for_witness "YOURACCOUNT" "drakos" true true
great job @drakos..Thanks buddy!!!
For those who are already using the script, check the ADDENDUM for an improved version. Thanks to @wackou for pointing out the ICMP issue.
Good work @drakos must be resteemed
You are one of my goto blogs as a new witness. Thanks for giving back.
Thanks for the kind words. I try to give back as much as I can. There's a lot I would like to write about, just not enough time! So many projects on Steemit π
hey @drakos, nice script ;)
I checked what you mentioned, and if you take abit's seed for example, the ping doesn't come back however you can actually connect to the seed node (verify with
$ telnet steem-seed1.abit-more.com 2001
). So I guess abit must've closed his firewall to not answer icmp requests, but the seed is online.On a related note the seed nodes status is now more resilient to random network errors that some people have been reporting. Although not an real fix for this issue, the page should now properly show those affected nodes as online. (see here)
Ah indeed, I overlooked the possibility of blocked icmp. This is problematic for the purpose of this script, which is to determine the best pings. Let me see if I can find a solution for that.