Repository
https://github.com/holgern/beem
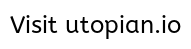
beem is a python library for steem. beem has now 526 unit tests and a coverage of 76 %. The current version is 0.19.39.
I created a discord channel for answering a question or discussing beem: https://discord.gg/4HM592V
New features
ImageUploader
Thanks to @embrebeyler and his python library imagehoster-python-client I was able to add a ImageUploader class to beem. I could simplify the original code to:
from beemgraphenebase.ecdsasig import sign_message
message = py23_bytes(self.challange, "ascii") + image_data
signature = sign_message(message, posting_wif)
signature_in_hex = hexlify(signature).decode("ascii")
It is now possible to upload images in bytes representation directly to steemitimages.com:
from beem.imageuploader import ImageUploader
from beem import Steem
import io
from matplotlib.backends.backend_agg import FigureCanvasAgg as FigureCanvas
import matplotlib.figure
from matplotlib.figure import Figure
fig = Figure(figsize=(10, 10), dpi=100)
canvas = FigureCanvas(fig)
ax = fig.add_axes([.1, .1, .8, .8])
ax.plot(range(15))
ax.set_xlabel('x')
ax.set_ylabel('y')
buf = io.BytesIO()
fig.savefig(buf, format='png')
buf.seek(0)
stm = Steem(keys=["xxx"])
io = ImageUploader(steem_instance=stm)
io.upload(buf.read(), "holger80")
with the result:
{'url': 'https://cdn.steemitimages.com/DQmXvmNs3UxXRYqwA7ieP3WMcTLJeRyN3UdvsejD46UqaMv/image'}
It's then possible to embed this link into a post:

update_nodes()
from NodeList
improved
update_nodes
calculates now the score for each node by:
score = (n - rank) / (n- 1) * 100
weigthed_score = score * weights_dict[benchmark]
sum_score += weigthed_score
where rank
is the rank of the node at the specific benchmark. n
is the number of nodes and weights_dict[benchmark]
the the weight for this benchmark. The sum of weights_dict
is one.
At the moment 5 benchmarks are calculated every hour are written into the metadata field of @fullnodeupdate.
Available benchmarks:
- block
- history
- apicall
- block_diff
- config
Api Definitions
Api Definitions are created with examples for all available API calls defined here.
Missing API calls were added
get_expiring_vesting_delegations
from beem.account import Account
acc = Account("emrebeyler")
print(acc.get_expiring_vesting_delegations())
[{'id': 2341553, 'delegator': 'emrebeyler', 'vesting_shares': '2439949.303020 VESTS', 'expiration': '2018-06-22T15:10:00'}, {'id': 2212414, 'delegator': 'emrebeyler', 'vesting_shares': '20338.649992 VESTS', 'expiration': '2018-06-25T23:48:45'}]
get_feed_entries
from beem.account import Account
acc = Account("gtg")
for f in acc.get_feed_entries():
print(f)
get_blog_authors
from beem.account import Account
acc = Account("gtg")
for h in acc.get_blog_authors():
print(h)
get_savings_withdrawals
from beem.account import Account
acc = Account("gtg")
print(acc.get_savings_withdrawals(direction="from"))
print(acc.get_savings_withdrawals(direction="to"))
get_escrow
from beem.account import Account
acc = Account("gtg")
print(acc.get_escrow())
get_account_reputations
from beem.blockchain import Blockchain
b = Blockchain()
for h in b.get_account_reputations():
print(h)
Discussions_by_author_before_date
from beem.discussions import Query, Discussions_by_author_before_date
for h in Discussions_by_author_before_date(limit=10, author="gtg"):
print(h)
get_transaction_hex
from beem.blockchain import Blockchain
b = Blockchain()
block = b.get_current_block()
trx = block.json()["transactions"][0]
print(b.get_transaction_hex(trx))
get_tags_used_by_author
from beem.account import Account
acc = Account("gtg")
print(acc.get_tags_used_by_author())
Replies_by_last_update
from beem.discussions import Query, Replies_by_last_update
q = Query(limit=10, start_author="steemit", start_permlink="firstpost")
for h in Replies_by_last_update(q):
print(h)
Trending_tags
from beem.discussions import Query, Trending_tags
q = Query(limit=10, start_tag="steemit")
for h in Trending_tags(q):
print(h)
get_vesting_delegations
from beem.account import Account
acc = Account("gtg")
for v in acc.get_vesting_delegations():
print(v)
Commit history
Try to improve blocks with threading and some more error caption
get_expiring_vesting_delegations
added to account
Account
get_expiring_vesting_delegations
added
Blockchain
- thread_limit parameter removed
- batch_mode set to True for threaded blocks()
- block sanity check added for threaded blocks()
Add missing api functions
Account
get_feed_entries
,get_blog_authors
,get_savings_withdrawals
,get_escrow
- Documentation improved
- Account parameter handling improved
Blockchain
get_account_reputations
added
ImageUploader added
- commit 169dc2b
- ImageUploader can be used to upload an image file or an image in bytes
Improved score calculated for update_nodes
Improved blocks with threads and improved unit test
Add Discussions_by_author_before_date and improve some other discussions class
Discussions
Discussions_by_author_before_date
added
beemapi/Nodes
- add an option to freeze a node, in order to prevent switching to the next node
get_vesting_delegations added and block date conversion improved
Account
get_vesting_delegations
added
More functions added and a complete list of API definitions added to docs
Discussions
Replies_by_last_update
andTrending_tags
added
Blockchain
get_transaction_hex
added
Account
get_tags_used_by_author
added
Docs
- apidifinitions added
Block and Blockchain improved and Readme adapted to changes
Block identifier handling improved and test_blockchain_treading improved
GitHub Account
If you like what I'm doing, please consider @holger80 as one of your witnesses (steemconnect).
Thank you for your contribution. Again a great update to a project.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @holger80
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @holger80 , took a break for a while, but big kudos from me on Beem! Keep it up, if you need a hand at something let me know.
@scipio