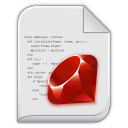
Some people like to create a bunch of accounts. And some people like to preemptively mute them. Here's a way to do that.
This assumes that the same person creates the accounts you want to mute. But this approach can be modified to work with other criteria like reputation instead.
As always, we use Radiator with bundler
. You can get bundler
with this command:
$ gem install bundler
I've tested it on various versions of ruby. The oldest one I got it to work was:
ruby 2.1.0p0 (2013-12-25 revision 44422) [x86_64-darwin14.0]
First, make a project folder:
$ mkdir radiator
$ cd radiator
Create a file named Gemfile
containing:
source 'https://rubygems.org'
gem 'radiator'
gem 'steem_api'
gem 'awesome_print'
Then run the command:
$ bundle install
Create a file named auto_mute_created_by.rb
containing:
require 'rubygems'
require 'bundler/setup'
Bundler.require
if ARGV.size < 1 || ENV['ACCOUNT_NAME'].nil? || ENV['WIF'].nil?
puts "Usage: ACCOUNT_NAME=name WIF=wif ruby #{__FILE__} <creator account> [broadcast]"
exit
end
creator_account_name = ARGV[0]
broadcast = ARGV[1] == 'true'
account_name = ENV['ACCOUNT_NAME']
wif = ENV['WIF']
tx = Radiator::Transaction.new(wif: wif)
api = Radiator::FollowApi.new
ignoring = []
count = -1
until count == ignoring.size
count = ignoring.size
response = api.get_following(account_name, ignoring.last, 'ignore', 100)
ignoring += response.result.map(&:following)
ignoring = ignoring.uniq
end
accounts = SteemApi::Tx::AccountCreate.where(creator: creator_account_name)
accounts = accounts.where.not(new_account_name: ignoring)
puts "Ignoring accounts: #{accounts.count}"
accounts.find_each do |account|
data = [:follow, {
follower: account_name,
following: account.new_account_name,
what: ['ignore']
}
]
tx.operations << {
type: :custom_json,
id: 'follow',
required_auths: [],
required_posting_auths: [account_name],
json: data.to_json
}
end
result = tx.process(broadcast)
if result.class == Radiator::Transaction
puts 'Not broadcasted.'
exit
end
ap result
Then run it (here we're using social
and its wif
, which is public, but you can use your own):
$ ACCOUNT_NAME=social WIF=5JrvPrQeBBvCRdjv29iDvkwn3EQYZ9jqfAHzrCyUvfbEbRkrYFC ruby auto_mute_created_by.rb noganoo true
The expected output will be something like this:
Ignoring accounts: 35
{
"result" => {
"id" => "e567d344d4f0ba2d4419380f1fb618c98c0a1c03",
"block_num" => 19128228,
"trx_num" => 14,
"expired" => false
},
"id" => 1
}
See my previous Ruby How To posts in: /f/ruby
over my head.....but......
wont muting by rep be too inclusive?
I'd only recommend it if you're matching on rep less than 25. Anyway, this script doesn't do that.
well done bro another great bot
really super post
Your post is very nice,
I'm a regular reader, your writing
And you very sincerely,
i upvoted you.
Please help me and vote hope,
Because your vote is very valuable to me.
Like a virtual fly swatter, good to keep the bugs under control. Now if there was a way to create one massive fly swatter to help exterminate the pests before they are hatched
Hello @inertia please let me use banjo in my discord server.
I do not now how people create and manage lot of accounts, I have one account and I gave my 3, 4 hours to steemit , I love to read posts that give me motivation
Another great tool integrated with the STEEM platform using Ruby. And what is good about this is that it comes through having at its core a real problem that sometimes we want to ignore certain accounts and we don't have a solution for that. Well, with this one you can "mute" such undesired accounts.
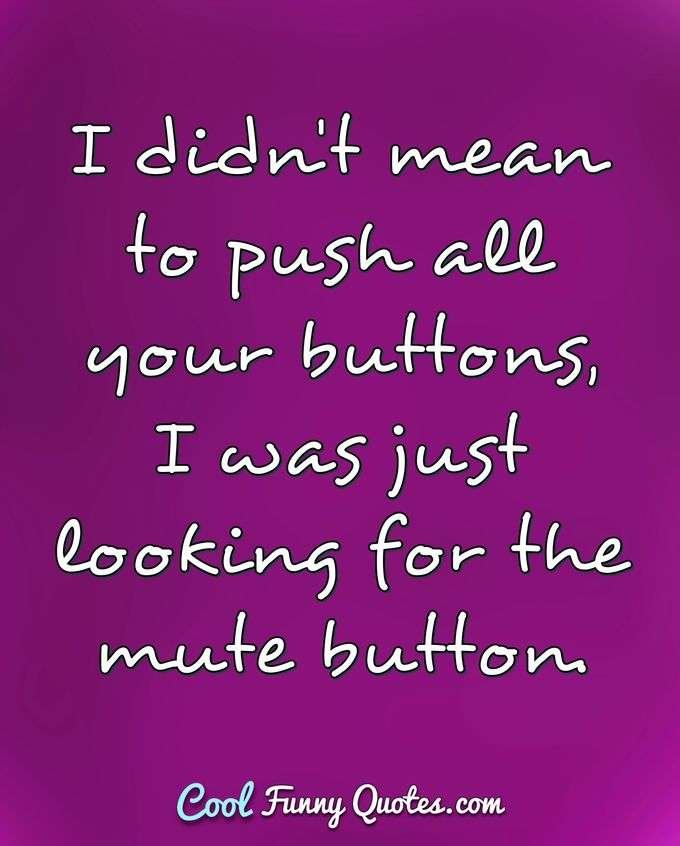
Wow this really helps a lot! Thank You!!!
Wow this really helps a lot! Thank You!!!
This post has received a 0.10 % upvote from @drotto thanks to: @banjo.