In the realm of advanced .NET development, as developers embrace asynchronous programming for improved performance, a new set of challenges emerges. Among these challenges, the notorious deadlock stands out as a formidable foe. This blog post delves into the intricacies of async/await in .NET and offers insights on identifying, preventing, and resolving deadlocks in your advanced applications.
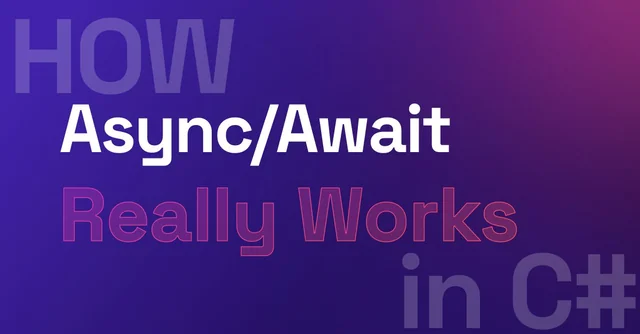
Understanding Async/Await and Deadlocks:
Async/await is a powerful feature in modern .NET, allowing developers to write non-blocking code and enhance application responsiveness. However, the asynchronous nature of these operations introduces the potential for deadlocks – situations where threads are stuck, waiting for each other indefinitely, bringing your application to a grinding halt.
Common Causes of Deadlocks in Async Code:
- Synchronous Calls Inside Async Methods:
- When calling synchronous methods within an async context, deadlocks can occur as the async method waits for the synchronous call to complete, creating a deadlock.
// Example of synchronous call inside async method
public async Task<int> MyAsyncMethod()
{
// Synchronous call causing potential deadlock
int result = SomeSyncMethod();
// Rest of the async code
return result;
}
- Nested Async Calls with Incorrect Usage:
- Incorrect usage of async/await within nested calls can lead to deadlocks if not handled carefully.
// Example of incorrect nested async calls
public async Task<int> OuterAsyncMethod()
{
// Incorrect usage leading to deadlock
int result = await InnerAsyncMethod();
// Rest of the async code
return result;
}
Identifying and Diagnosing Deadlocks:
Debugger Tools:
- Leverage debugging tools such as Visual Studio's debugger to identify deadlock situations. Analyze thread states and execution paths to pinpoint the root cause.
Profiling Tools:
- Use profiling tools like JetBrains dotTrace or Microsoft Performance Profiler to gain deeper insights into the asynchronous behavior of your application.
Preventing and Resolving Deadlocks:
- Avoid Synchronous Calls in Async Methods:
- Refrain from making synchronous calls within async methods. If necessary, use asynchronous alternatives to keep the flow non-blocking.
// Corrected example using async alternatives
public async Task<int> MyAsyncMethod()
{
int result = await Task.Run(() => SomeSyncMethod());
// Rest of the async code
return result;
}
- ConfigureAwait(false):
- Use
ConfigureAwait(false)
when awaiting tasks to prevent deadlock situations by allowing the continuation to run on any available thread.
- Use
// Example of using ConfigureAwait(false)
public async Task<int> MyAsyncMethod()
{
int result = await SomeAsyncMethod().ConfigureAwait(false);
// Rest of the async code
return result;
}
- Async Locks and Semaphores:
- Implement asynchronous locks or semaphores when dealing with shared resources to ensure thread safety without risking deadlocks.
// Example of using SemaphoreSlim
private static readonly SemaphoreSlim semaphore = new SemaphoreSlim(1, 1);
public async Task<int> MyAsyncMethod()
{
await semaphore.WaitAsync();
try
{
// Critical section of code
return await SomeAsyncMethod();
}
finally
{
semaphore.Release();
}
}
Conclusion:
Deadlocks in advanced .NET applications are formidable challenges, but armed with the knowledge of async/await intricacies and the right preventive measures, developers can navigate these complexities. By avoiding common pitfalls, utilizing debugging tools, and implementing async best practices, you can ensure your asynchronous code runs smoothly, delivering high-performance and responsive applications. Happy coding!
Congratulations @dotnetguru! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 20 posts.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Check out our last posts: