This week block.one’s blockchain c++ development team has picked up 4 new members and has been interviewing a few more. The new recruits have already begun making contributions to the code.
Removing Registered Message Schemas
With the move to WebAssembly (WASM) we have had to re-evaluate some of our earlier design decisions based on the assumption of using Wren. Because WASM is language independent, every application would have to provide different WASM for parsing the messages passed to the application. This means the ABI (application binary interface) is technically defined by the WASM parsing and not our prior builtin registered message types and formats.
Since the blockchain is unable to enforce the message schemas and every contract would have to independently parse and validate the incoming binary data, we opted to remove the blockchain defined message schemas. This gives more power to application developers.
Changing Account Name Structure
One of the bigger changes to the code this week was changing account names from 256 bit strings to 60 bit integers that convert to human-readable names via base32 encoding. This limits the account names to 12 lowercase letters and a few numbers. The average Twitter account is only 12 characters long.
This change was made primarily out of bandwidth, memory, and performance considerations. From an applications logic perspective an account name is simply a unique identifier and they are used everywhere. The old ABI would serialize these account names as length-prefixed strings and require a computationally intensive unpacking process.
By moving to a fixed-width integer representation for accounts we got performance, but the developer experience was less friendly. To resolve this we simply print the integers as base32 and this gives human-readable names.
Longer names can still be supported by registering them with a name service contract.
A typical transfer message is 75% smaller after this change, which makes a big difference when you are processing 10's of thousands of them per second.
Filtering Floating point from WASM
We updated the WASM processing to detect and reject code that uses floating point operations which can generate non-deterministic behavior.
Sandboxing WASM Runtime
We made significant progress toward injecting checkpoints into WASM to allow us to check the runtime and abort if too much time has passed. This is a critical component of running untrusted code.
New C++ Simplecoin (80,000 TPS)
In one of my prior updates I showed a proof-of-concept currency smart contract written in C and achieving 50K transactions per second. I mentioned that I thought we could clean up the syntax and today I am happy to show a new implementation of the simplecoin in C++ that gets compiled to WASM and then executed. Using a tight loop of generating and pushing transactions to the blockchain we achieved 80K TPS transferring funds from one account to another in a single thread.
Real world performance may be higher or lower based upon the evolving architecture, it is still too early to tell. All measurements were made on a 4Ghz Intel Core i7 in a 2014 iMac.
struct Transfer {
uint64_t from;
uint64_t to;
uint64_t amount;
char memo[];
};
static_assert( sizeof(Transfer) == 3*sizeof(uint64_t), "unexpected padding" );
struct Balance { uint64_t balance; };
void on_init() {
static Balance initial = { 1000*1000 };
static AccountName simplecoin;
simplecoin = name_to_int64( "simplecoin" );
store( simplecoin, initial );
}
void apply_simplecoin_transfer() {
static Transfer message;
static Balance from_balance;
static Balance to_balance;
to_balance.balance = 0;
readMessage( message );
load( message.from, from_balance );
load( message.to, to_balance );
assert( from_balance.balance >= message.amount, "insufficient funds" );
from_balance.balance -= message.amount;
to_balance.balance += message.amount;
if( from_balance.balance )
store( message.from, from_balance );
else
remove( message.from );
store( message.to, to_balance );
}
Not only is the code cleaner and easier to read, the generated WASM code is much smaller too. Most of this time and space savings is made possible by changing the account name and ABI for this contract. In this case the ABI uses a zero-parse copy from the database to the c++ struct and all account names are fixed length.
You can experiment with this code on WasmFiddle with this shared link.
Conclusion
The EOS development team is growing and the technology is progressing with all signs pointing toward a very high-performance platform even running in a single thread. Stay tuned for more updates!
Thanks for using my image. : )
Nice to see these notes and a EOS POC.
Good job.
Nice post! What will happening, if someone resteem your post?
Report from bytemaster himself, just like good old days :) I would be great if payout wasn't declined.
My understanding of how EOS achieves massive transaction speed / scalability is that it delegates block production to a single actor for each block. How is block forgery NOT possibly a problem? I've read the explanations and it's somehow 'the longest chain wins' like Bitcoin ... but I don't see how that actually applies in a 'one actor per block' scheme? One actor just forges, block is hashed, boom, done: nobody the wiser. Hope my question makes sense :) and I'd love to know the answer! Big fan of EOS in all other ways than this lingering doubt.
Bitcoin works same way, one actor per block. Bitcoin uses hash and pow for signature which can be forged by anyone willing to spend as much pow, DPOS uses private key signatures which cannot be forged without hacking an account.
That's great informative comment.
So with all these layers of security it is still not safe from the threat of a hacker ? Now I totally feel safe with the $20,000 I just put into this project :(
Well, I guess you best leave it in your bank account or bitcoin wallet which are both also vulnerable to having their accounts "hacked".
Username checks out.
I'm glad to hear that with the increase in technology and performance, the safety is even more important.
dan why this Payout decline of ur post?
Yes
In an era of permissioned blockchains gaining favor with financial institutions over decentralized, freely trading cryptocurrencies like Bitcoin, Ethereum, or Ripple, Safe Cash is one of the first blockchains to be commercially viable that can meet the transaction processing speed and throughput requirements of today's market. Safe Cash employs instant settlement in under five seconds, improved security, and controlled consensus that does not rely on miners or any intermediary coin that must be purchased. It allows banks to wean themselves off the high-priced, inefficient SWIFT network that can take days to transfer money. Banks can have their own "white label" blockchain that they control and manage. Inter-bank settlement can be achieved with multi-currency wallets, a separate bank settlement blockchain, or a combination thereof, depending on bank requirements and legal compliance.
Achieving 80K Transactions Per Second would be truly incredible, i guess EOS will be the fastest among all crypto-currencies. Congratulations and warm welcome to all the new EOS developers .
Bitshares already does 100k transactions per second.
For now enjoy bitshares and steem. Still the fastest in crypto land.
If they can pull it off, it will be the fastest without a doubt, c++ and "c" are machine level coding languages basically, the closer you get to machine level, faster the data transmission because gpu's are hardware so no need for conversion after a hash hack. I truly applaud them on trying.
Is the 100K per second what they are saying they will achieve or has this technology already been put in place ? I heard some people quoting 1 million per second
This could easily become the best crypto currency to be ever made if everything succeeded. 80,000 tx per second is going to be incredible. Looking forward to it's development.
This is going to be interesting. Very interesting - hope you keep up the log over the next 12 months and look forward to reading your updates!
Me too hopefully there is a positive result some where along the lines of bitshares. Just bought 50 ether work and hoping for the best
Slow and steady win the game.
Great update and very good news, what a potent crew in the making. This is awesome! All for one and one for all!!! Namaste :)
Very interesting, thank you!
Hey Dan!!! I came by to learn some more and say have a good weekend.
You must be stoked about the Poloniex news I broke on my page today about 10 mins after they finally released the stranglehold on everyone's BTS wallets for like 100 years slight hyperbole ---- they still cannot find the 500 SBD I transferred to them a week ago and I cannot reach them.
Like with BTS, I am still telling people to check it out, sending people over -- I am still learning.
It is our Canadastan Day long weekend here like your Almost Independence Day there coming up.... I wish you the best always, some rest and fun and stay strong man.
I am always rooting for you!!!
steemit.com/eos/@dan/eos-d…
Disclaimer: I am just a bot trying to be helpful.
This could easily become the best crypto currency to be ever made if everything succeeded. 80,000 tx per second is going to be incredible. Looking forward to it's development.
All Graphene based cryptos have that already (Bitshares, Steem, Peerplays etc...) with a 100.000 or 150.000 transactions...
Oh,that is awesomer then!
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by kryptokayden from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, and someguy123. The goal is to help Steemit grow by supporting Minnows and creating a social network. Please find us in the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you like what we're doing please upvote this comment so we can continue to build the community account that's supporting all members.
Found this article very informative and fascinating and about the possibilities that lie ahead.
That's funny :D
hehe, thanks :)
Very interesting. Will surely check all your blog
nice
First I though there will be 'EOS' not 'steemit' but it made me happier anyway :) Have a nice day :)
That was REALLY nice brother!
Really nice this. I wish to start experimenting with genetic algorithms kind of stuff on EOS. Then I will also like to test simple codes to gather data from multiple sources and concentrate them on a single database. The usage from EOS on this last one is that I need to do some processing on the fly before storing the results, and by using EOS makes it perfect to loose the dependency of having a computer or expensive computational device near the collection source.
Well done post You deserve for getting Upvote from me. I appreciate on it and like it so much . Waiting for your latest post. Keep your good work and steeming on. Let's walk to my blog. I have a latest post. Your upvote is high motivation for me. Almost all Steemians do their best on this site. Keep steeming and earning.
This comment has received a 0.07 % upvote from @booster thanks to: @hamzaoui.
Tried the example. "Build" and then "run", I get:
line 3: Uncaught TypeError: wasmInstance.exports.main is not a function
is supposed to output something?
no, you have to save the WASM and run it on the blockchain.
@dantheman when will the next EOS update happen?
So much great progress on EOS, is there a published roadmap or expected release date for the EOS blockchain? Thanks for your amazing contribution to the decentralised world we are creating ;-)
I bought some EOS a few hours ago at Bitfinex. Went very smooth, Bitfinex is a class exchange. I bought based on Suppoman's youtube video. He's a steemer too. Hope it makes top 3 coins soon. :)
It is nice to see great progress being made on the team building and especially the code. Thanks for the update.
EOS will be big in next 12-24 months!
I put 0.5 ETH into the ICO on day 1. Just checked the contract address on Etherscan and with 14 hours left of the initial 5 day period 455,000 Ether have been received. Say another 45,000 ETH is received before the end of the period and the total ETH received is 500,000 I will end up with 400 EOS tokens based on my 0.5 ETH contribution. Is that right? When can they be claimed? At the end of the 5 days or at the end of the entire 300+ day period in which coins are being offered?
https://etherscan.io/address/0xd0a6e6c54dbc68db5db3a091b171a77407ff7ccf
You will get 200 eos because you participated with 0.5 eth. (200000000 eos / 500000 eth) * 0.5 eth
You can claim them any time after the end of the 5 days period.
The way I understand it is the amount of tokens you received is fixed. The value of a token will of course fluctuate.
I don't think you need to 'claim' them since etherscan.io already shows them being transfered to you.
https://etherscan.io/address/YOURADDRESS#tokentxns
Replace YOURADDRESS with the address you sent from.
I hope someone will comment if I have this wrong.
Definitely, you will need to claim your tokens as supposed to be transferred to your Ether Address
Instructions to follow are posted in the EOS Token Distribution https://www.eos.io/instructions
Great stuff @dan 👍
The project is super interesting and can't get enough of it.
Just out of curiosity, where does all that money go if you decline a payout?
it is as if I never made the post.
I mean like does it circulate to the curators of the post , does it help boost the steem economy in other ways, aside from a well written post of course? I was just curious.
There is no payout. Neither for the curators.
no payout for anyone, just author reputation increases (which is fine, obviously :-)
Other posts each get a bit more.
I am very optimistic EOS will be the dominate worlds Blockchain
Me too, I was optimistic enough to put a fair share of my portfolio into it. After all the time I have spent trading crypto the biggest issues I have found is lack of bandwidth. Hopefully EOS can address all these issues moving forward.
This is a good development, thanks a lot to you and your team. Following you and thus will certainly stay tuned for more updates. Team is great beacuse of you and your team working behind the scene to add value to the platform.
I wish you and your team more success.
Dont forget to check out my post for a link to collect free crypto..a once in a life time opportunity
80K TPS is incredible! Well done! And I thought I was all cool today having made a simple little He-Man finding Skeletor game. Now I feel like a complete programming novice, lol.
awesome stuff,
I wish to thank you personally for voting for me this past year. Steem was very fun in the beginning, when most people recognized each other. And now it is a little easier to get followers. The middle time had my best unrewarded work, however.
dan, its actually dantheman the upvoted me a while back, but whatever. Hoping you see this before everything comes in.
Real world performance will definitely be lower due to network lag and such. For testing I'd try running over the Internet and posting those results.
Hi @dan
You really are genius! I'm new to all this but trying to understand it, thanks for these informative reads. My mind is boggled but for less than a month into this - I think i'm okay... :D
I just hope Dan will stay with the project -
That would make it a 100% success as we all wish it to be
Congratulations @dan!
Your post was mentioned in my hit parade in the following category:
I love how this blog started with a stardate!
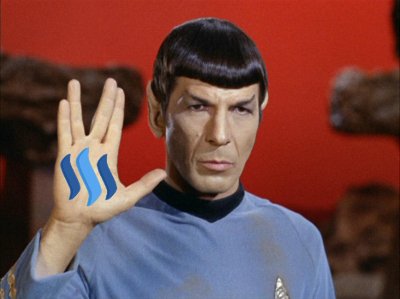
Live long and prosper, Mtherfcker!
Amazing !!future is here ,only we need wait a little bit, great to know that team is collecting and growing , I know here is huge potential . Thank.y ou for the article ,good news.
Great update @dan! I look foward to the future of EOS!
My understanding of how EOS achieves massive transaction speed / scalability is that it delegates block production to a single actor for each block. How is block forgery NOT possibly a problem? I've read the explanations and it's somehow 'the longest chain wins' like Bitcoin ... but I don't see how that actually applies in a 'one actor per block' scheme? One actor just forges, block is hashed, boom, done: nobody the wiser. Hope my question makes sense :) and I'd love to know the answer! Big fan of EOS in all other ways than this lingering doubt.
Excellent news! Nice to be a frontrow sitter following the program. Good work!
Please upvote me im a poor dev
great info
WOW,expect!
Very interesting, thank you!
That code is really easy to understand c:
The most precious coin here is the mind of this man, and the most fast blockchain is his mind.
i really admire this guy @dan
Hello! I just upvoted you! I help new Steemit members! Upvote this comment and follow me! i will upvote your future posts! To any other visitor, upvote this post also to receive free UpVotes from me! Happy SteemIt!
code
Great work man on future development of codes.
I really like your projects Dan.
it's july 1, but still no signs of trade on bitfinex. any info, when will be the gates open?))
What do you guys think? After we like 4-7x our profits sell it and buy back after a year when it goes back down instead of holding for a whole year?>