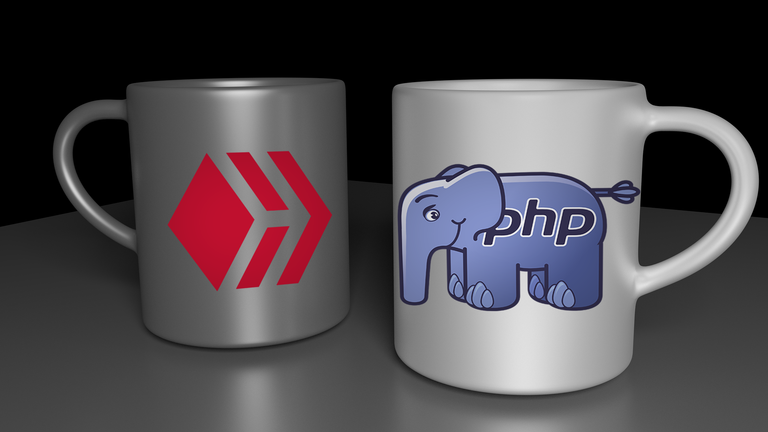
Hive-PHP
This one took a while despite having some demand from PHP developers. But it is finally here.
The serialization and signing are done natively on PHP. Every line of code has been written by me.
I did not test all the operations but all the operations should work. Please do test if you can and let me know if there are any problems.
The documentation is available on both gitlab and packagist. But for the sake of keeping them on blockchain will put them here too.
Installation
composer require mahdiyari/hive-php
Initialization
$hive = new Hive($options?);
Example:
include 'vendor/autoload.php';
use Hive\Hive;
$hive = new Hive();
// or
// default options - these are already configured
$options = array(
'rpcNodes'=> [
'https://api.hive.blog',
'https://rpc.ausbit.dev',
'https://rpc.ecency.com',
'https://api.pharesim.me',
'https://api.deathwing.me'
],
'chainId'=> 'beeab0de00000000000000000000000000000000000000000000000000000000',
'timeout'=> 7
);
// Will try the next node after 7 seconds of waiting for response
// Or on a network failure
$hive = new Hive($options);
Usage
API calls:
$hive->call($method, $params);
Example:
$result = $hive->call('condenser_api.get_accounts', '[["mahdiyari"]]');
// returns the result as an array
echo $result[0]['name']; // "mahdiyari"
echo $result[0]['hbd_balance']; // "123456.000 HBD"
Private Key:
$hive->privateKeyFrom($string);
$hive->privateKeyFromLogin($username, $password, $role);
Example:
$privateKey = $hive->privateKeyFrom('5JRaypasxMx1L97ZUX7YuC5Psb5EAbF821kkAGtBj7xCJFQcbLg');
// or
$privateKey = $hive->privateKeyFromLogin('username', 'hive password', 'role');
// role: "posting" or "active" or etc
echo $privateKey->stringKey; // 5JRaypasxMx1L97ZUX7YuC5Psb5EAbF821kkAGtBj7xCJFQcbLg
Public Key:
$hive->publicKeyFrom($string);
$privateKey->createPublic()
Example:
$publicKey = $hive->publicKeyFrom('STM6aGPtxMUGnTPfKLSxdwCHbximSJxzrRjeQmwRW9BRCdrFotKLs');
// or
$publicKey = $privateKey->createPublic();
echo $publicKey->toString(); // STM6aGPtxMUGnTPfKLSxdwCHbximSJxzrRjeQmwRW9BRCdrFotKLs
Signing
$privateKey->sign($hash256);
Example:
$message = hash('sha256', 'My super cool message to be signed');
$signature = $privateKey->sign($message);
echo $signature;
// 1f8e46aa5cbc215f82119e172e3dd73396ad0d2231619d3d71688eff73f2b83474084eb970955d1f1f9c2a7281681d138ca49fe90ac58bf069549afe961685d932
Verifying
$publicKey->verify($hash256, $signature);
Example:
$verified = $publicKey->verify($message, $signature);
var_dump($verified); // bool(true)
Transactions
There are two ways to broadcast a transaction.
Manual broadcast
Example:
$vote = new stdClass;
$vote->voter = 'guest123';
$vote->author = 'blocktrades';
$vote->permlink = '11th-update-of-2022-on-blocktrades-work-on-hive-software';
$vote->weight = 5000;
$op = array("vote", $vote);
// transaction built
$trx = $hive->createTransaction([$op]);
// transaction signed
$hive->signTransaction($trx, $privateKey);
// will return trx_id on success
$result = $hive->broadcastTransaction($trx);
var_dump($result);
// array(1) {
// 'trx_id' =>
// string(40) "2062bb47ed0c4c001843058129470fe5a8211735"
// }
Inline broadcast
Example:
$result = $hive->broadcast($privateKey, 'vote', ['guest123', 'blocktrades', '11th-update-of-2022-on-blocktrades-work-on-hive-software', 5000]);
var_dump($result);
// array(1) {
// 'trx_id' =>
// string(40) "2062bb47ed0c4c001843058129470fe5a8211735"
// }
Notes for Transactions
Operations are in the following format when broadcasting manually:
array('operation_name', object(operation_params))
example:
$vote = new stdClass;
$vote->voter = 'guest123';
$vote->author = 'blocktrades';
$vote->permlink = '11th-update-of-2022-on-blocktrades-work-on-hive-software';
$vote->weight = 5000;
$op = array("vote", $vote);
Any parameter in opreation that is JSON (not to be confused with json strings), should be passed as an object.
Example:
The beneficiaries
field in comment_options
is in JSON format like this:
$beneficiaries = '[0, {"beneficiaries": [{"account": "mahdiyari","weight": 10000}]}]';
We should convert that into an object.
$beneficiaries = json_decode($beneficiaries);
(beneficiaries go inside the extensions)
$result = $hive->broadcast($privateKey, 'comment_options', ['author', 'permlink', '1000000.000 HBD', 10000, true, true, [$beneficiaries]]);
How to know the operation parameters
Easiest way is to find the operation on a block explorer.
e.g. https://hiveblocks.com/tx/2062bb47ed0c4c001843058129470fe5a8211735
You can also search the operation in /lib/Helpers/Serializer.php
to get an idea of what parameters it requires.
Any missing features?
Create an issue or reach out to me.
License
MIT
I hope PHP developers enjoy this and get away from the "hacks" they used so far to interact with Hive blockchain.
Composer package: https://packagist.org/packages/mahdiyari/hive-php
Gitlab repository: https://gitlab.com/mahdiyari/hive-php
Almost forgot the bonus content!
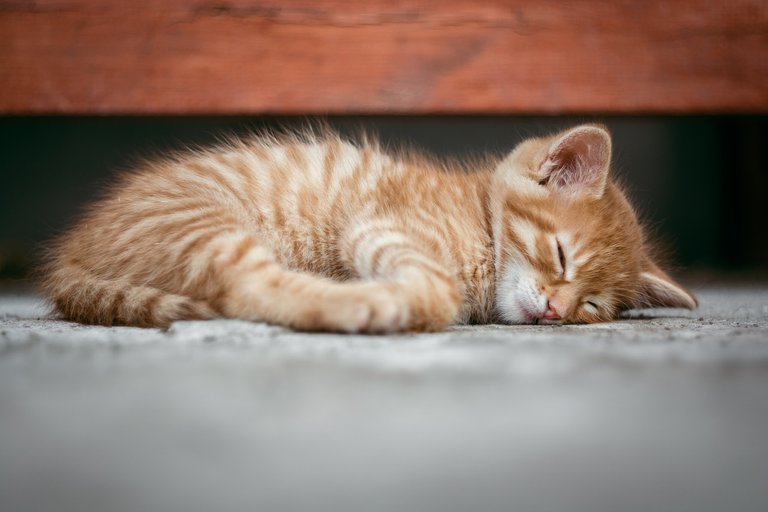
Made with ❤️ by @mahdiyari
Image source: pixabay.com
OMG THANKK YOU THANK YOU!!! This is SO GREAT!!! ❤️💛💚💙💜❣️
I just updated my post about verifying signatures in php to point here to your post!!! This is so much better and cleaner if you can install ext-gmp!!! This is so GREAT!!! Thank you again!!!
https://peakd.com/stem/@innerwebbp/solved-php-native-hive-signature-validation-and-the-hivewordpress-sso-single-sign-on
Oh, wow, now, that was a lot of work, but you can expect devs to storm Hive. There are so many PHP devs
And build what? Everything on hive all the dapps...they come snd they go...its like the steemit dot com effect... we need one large stakeholder who is famous to give out huge upvotes to make this work again
This definitely have a lot of work been put into it which is awesome. Keep up the good work progressing.
That is nice.
Currently, I am working on @hiveland.dapp that uses PHP. In this project we don't need to interact with Hive but we need to interact with Hive Engine and in the beginning it was hard because I needed to implement all the interactions with a Hive Engine node that we need.
It is nice to see some "libraries" be implemented on PHP and other langaunge to help developers to interact with our ecosystem.
Wow! This is a great work. Thanks for sharing it here.
as a beginner doing activities in hive, i have to study this more deeply because it is very important to develop writing performance in hive. Growth in the hive is my goal and the unknown ways will always be learned. thank you friend this is very useful for me.
~~~ embed:1552036131616821249 twitter metadata:WWFuUGF0cmlja198fGh0dHBzOi8vdHdpdHRlci5jb20vWWFuUGF0cmlja18vc3RhdHVzLzE1NTIwMzYxMzE2MTY4MjEyNDl8 ~~~
The rewards earned on this comment will go directly to the people( @mahdiyari, @shiftrox ) sharing the post on Twitter as long as they are registered with @poshtoken. Sign up at https://hiveposh.com.
Your content has been voted as a part of Encouragement program. Keep up the good work!
Use Ecency daily to boost your growth on platform!
Support Ecency
Vote for new Proposal
Delegate HP and earn more
Congratulations @mahdiyari! Your post has been a top performer on the Hive blockchain and you have been rewarded with the following badge:
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Support the HiveBuzz project. Vote for our proposal!
LOVED IT!
I didn’t understand a thing but the cat deserve a lovelly Up 💓
Wooooooow, this is very wonderful buddy. I love you work and the cat
I don't know how much time you put into this work, but it is certainly very valuable.
Thanks for sharing.Hello @mahdiyari
Amazing work, thank you! This will help a lot! 👍
This is very beautiful, I love your work and too be honest, I wish you could teach me this beautiful hand work of yours it will be a Honor to receive teachings from you.
Awesome!!
Lol is the php elephant related to India?
https://docs.php.earth/php/community/elephpant/
Congratulations @mahdiyari! Your post has been a top performer on the Hive blockchain and you have been rewarded with the following badge:
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out the last post from @hivebuzz:
Support the HiveBuzz project. Vote for our proposal!
Hello good time. Dear friend, is it possible to talk to you on WhatsApp or Telegram? Or should I have a contact number?
telegram @mahdi_Edw
Wow. This is so much effort put into one piece. Well done @mahdiyari
Hello.
I am using your library in my project. Does my project need delegation. I tried $hive->broadcast($privateKey ...) for delegation and it didn't work when Amount delegation > 10000 VEST. The error I get is: missing required active authority:Missing Active Authority sora-haraTransaction failed to validate using both new (hf26) and legacy serialization. The key I use is Active Key private. Can you share with me why? Thank you.
That error can be because of wrong parameters of the transaction. Send me the full code.
Thanks for your answer. This is my code.
$ops = [
"sora-hara",
"tuanbh",
"5000.000000 VESTS"
];
$hive = new Hive();
// create Obj privateKey
$key = $hive->privateKeyFrom(HIVE_DELEGATION_KEY);
// call API
$request = $hive->broadcast($key, 'delegate_vesting_shares', $ops);
It succeeds when VEST = 2000. AND >= 5000 then error.
I checked with the js library that the sign function to create a signature is giving a different value than your library when putting in the same Transaction. But I can't tell where it's coming from because the encryption libraries of the 2 are different. You code you are correct. Can you give me more information about the sign signature? Version of the library that is associated with it.
When you create a transaction, its parameters are dynamic so the signature will be different every time you make the same exact transaction. Also, for the same exact transaction (assuming the dynamic parts are fixed), it is completely fine to have multiple unique signatures.
Make sure the account you are delegating has enough VESTS.
The code seems fine but I will do some testing later to confirm it is fine.
Can you tell me your test results with delegation > 5000 VESTS? If it's completely fine. Can you share with me the versions of the libraries involved in the sign key? thank you so much.
About testing. I got a transaction and set them directly to the param of the JS and PHP sign functions. I then used the generated signature of JS for PHP and the transaction was successful. When I pass specific param the JS signature result always returns a value so I don't think there will be any other interference resulting in the 2 different results.