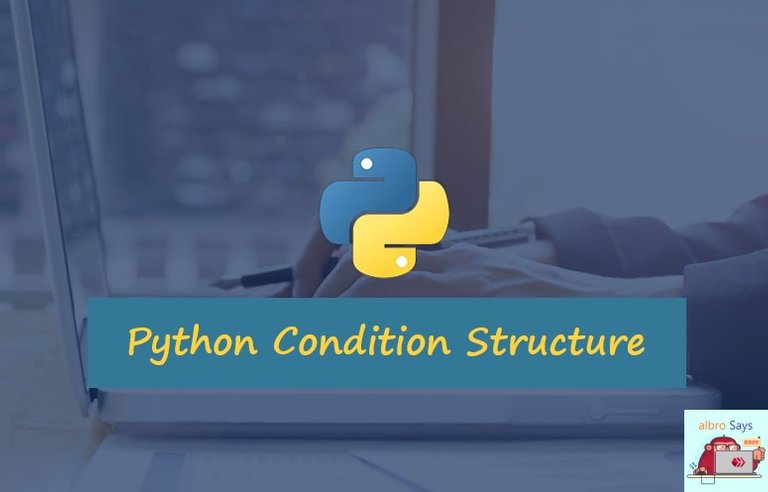
Table of Contents
- Condition statement in Python
- The
if
statement in Python - The
else
statement in Python - Python
elif
conditional statement - Summary: Post condition in Python
We can make decisions with the help of conditions in programming languages. Using the if
statement in Python, you can specify that if one or more conditions are met, to execute certain codes. In this post, I will talk about the condition in Python and the methods of using it.
In programming languages, just like the things we say every day, we have many buts and ifs! for example:
- If the user approves the message, log the information.
- If the entered number is less than 100, double it.
- If the coefficient number is 3 or more than 999, show a thank you message.
- If the number is odd, display it and if it is even, subtract one from it.
These are simple and different conditions that can be implemented using the if
statement and the if else
statement in Python. If you don't know what a statement is, you can see the post on types of programming statements.
Condition statement in Python
As you have noticed so far, with the help of a condition, if a certain situation exists, we will execute certain codes.
The diagram below shows how to execute the simplest type of conditional statement (with one condition).
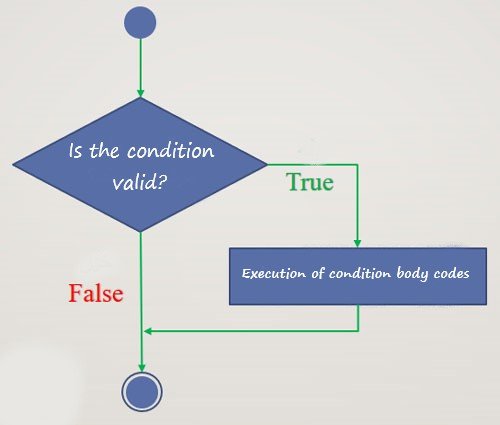
How are conditions defined?
To execute the condition, we must first specify a condition. By condition, I mean what we want other code to execute if it happens.
Conditions are either True
or False
. These types of values are called Boolean values.
To compare numerical values, we must use comparison operators. Comparison operators are:
==
to check if two values are equal!=
to compare two values that are not equal<
and>
for greater and less comparison<=
and>=
to compare greater equal and less equal
Suppose we want to do something special if the value of variable i
is equal to 1. So our condition becomes:
i == 1
The result of this comparison will be a Boolean expression.
If we want to use several conditions at the same time, we will use the keywords and
and or
between the conditions.
and
: All conditions must be met at the same time.or
: At least one of the conditions must be equal.
Now it's time to get familiar with the structure of Python's conditional statements. Let's get acquainted with the 3 main condition structure in Python.
The if
statement in Python
The foundation of all conditionals in Python begins with the if
keyword. The structure of a simple condition in Python is as follows:
if condition:
doSomeThing()
condition
is our condition or conditions.- And
doSomeThing()
is a piece of code or a function call.
As you can see, defining the condition in Python is very simple and similar to conversational language!
Suppose we want to print a Accepted
message if i
is greater than 10
. For this, we do the following:
if i >= 10:
print("Accepted!")
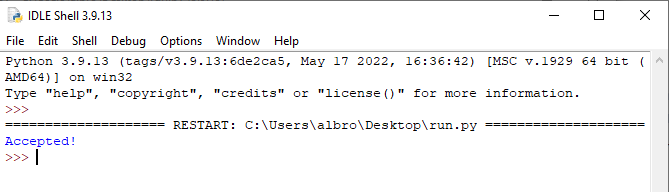
simply!
Now we want to print a Accepted
message if the value of i
is greater than 10
and the value of j
is exactly equal to 25
:
if i >= 10 and j == 25:
print("Accepted!")
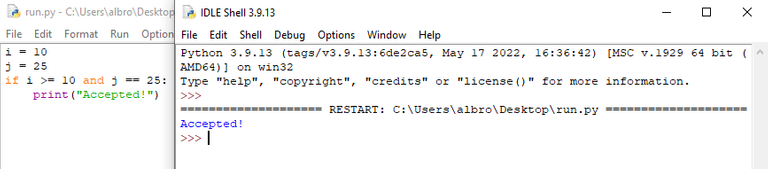
The else
statement in Python
In everyday conversations, we also use more complicated conditions. For example, we say:
If the weather is sunny tomorrow, I will go to the street and otherwise I will stay at home!
Pay attention to the second part of the said phrase. What caused the separation of the second part of the phrase?
You guessed it: "Otherwise."
The equivalent of "Otherwise" in Python conditional structure is known as the else
keyword and its usage is as follows:
if condition:
# code 1
else:
# code 2
If the condition was true (True
), code 1 will be executed, otherwise code 2 will be executed.
Suppose we have a number in the num
variable. We want to check if this number is an whole number or negative? Whole numbers are in the range 0 and greater than that.
So we have to check that if the value of the variable is greater than or equal to 0, then it will be an whole number and otherwise it will be a negative number.
num = 7
if num >= 0:
print("Number is Whole!")
else:
print("Number is Negative!")
After executing the code, the condition is satisfied and the third line is executed:
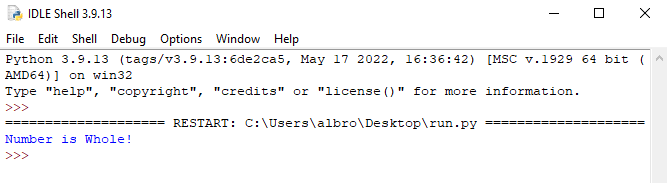
If we set the value of the variable equal to a negative number, line 5 will be executed.
num = -21
if num >= 0:
print("Number is Whole!")
else:
print("Number is Negative!")
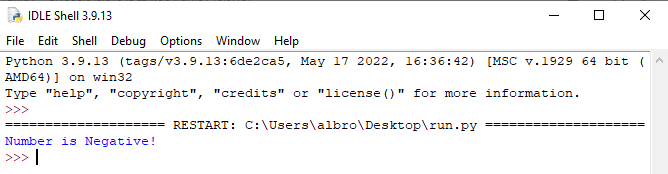
The order of executed lines in the if...else
conditional structure above is as follows: 1 -> 2 -> 4 -> 5
Python elif
conditional statement
So far, we have learned about the basic structure of condition in Python. Another keyword is for conditional structures where we want to check several times and several different conditions.
The word elif
is an abbreviation of the else if
statement. Consider the following colloquial sentence:
If it's sunny tomorrow, I'll go outside, if it's rainy I'll go to the park, otherwise I'll stay at home.
The above statement is divided into three parts: if [part 1] else if [part 2] otherwise [part 3]
Depending on the situation, the second part can be repeated over and over again. But in each conditional structure, the first part and the third part exist only once.
The if...elif...else
structure in Python is defined as follows:
if condition1:
# code 1
elif condition2:
# code 2
else:
# code 3
By repeating the second part, we can check different conditions over and over again; Similar to the following:
if condition1:
# code 1
elif condition2:
# code 2
elif condition3:
# code 3
elif condition4:
# code 4
else:
# code 5
Consider the same numerical example as before, except that if the number is positive, it will display one message, if it is zero, another message, and otherwise (if it is negative) an associated message.
Let's get the number from the user:
num = int(input())
if num > 0:
print("Number is Positive!")
elif num == 0:
print("Number is Zero!")
else:
print("Number is Negative!")
We run this code and give it some different numbers as input. You can see the result in the image below.
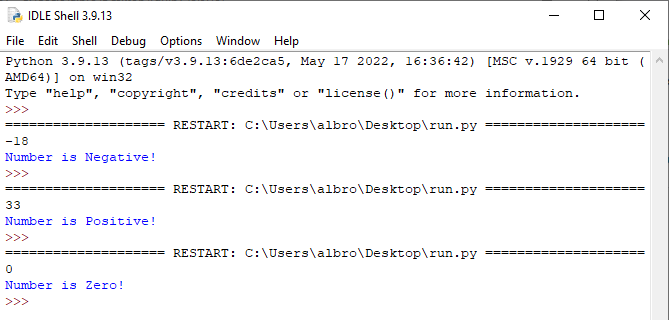
Summary: Post condition in Python
In this post, we got acquainted with the structure of terms in Python. Conditions are used when we want a specific piece of code to be executed if a certain condition is met. The condition structure in Python uses three main keywords:
if
: to start the conditional structure and check the conditionelif
: checks another condition if the main condition is not met. (optional)else
: will be executed if the condition (or conditions) is not met. (optional)
Conditionals are used a lot in any programming language. So, in addition to reviewing the examples in this post, try to code some examples for yourself.
https://leofinance.io/threads/@albro/re-leothreads-7k9f6r
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🏅 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 3250 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
Check out our last posts:
Support the HiveBuzz project. Vote for our proposal!