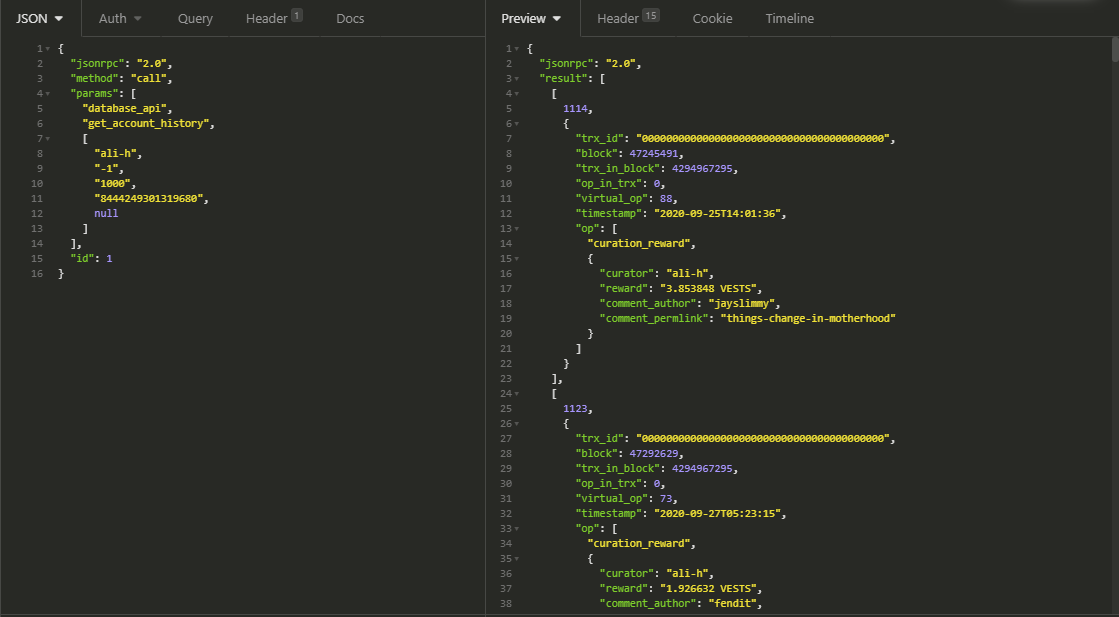
So there's recently been some changes in the account history API. Now it allows us to fetch account history (duh), But we can filter out specific operations making it much faster and customizable.
This is not fully implemented to all nodes yet, so it is experimental. I've used it on several nodes... https://api.hive.blog, https://api.deathwing.me, https://api.pharesim.me/ with direct request... but there is a block of code in hive-js 0.8.9 to pop extra parameters if the node isn't https://api.hive.blog.
@hiveio/hive-js/lib/api/transports/http.js
//SPECIAL CODE - can be removed after all API node operators upgrade to get the updated get_account_history api call
if (this.options.uri !== 'https://api.hive.blog' && data.method === 'get_account_history' && data.params.length >= 4) {
//We are experimenting with a new version of get_account_history that can now take up to 5 params
//but this is only deployed on api.hive.blog nodes, so if this particular request is going to a different
//backend, just strip the extra parameters off the call to avoid breaking it. Once all API nodes have upgraded
//this code can be removed.
while (data.params.length > 3) {
data.params.pop();
}params = [api, data.method, data.params];
}
//END SPECIAL CODE
Guide
This feature requires two more parameters operation_filter_low
and operation_filter_high
in get_account_history
request. we are going to use latest version of hive-js (0.8.9) in the tutorial below.
So firstly, to get things up, along with the normal hive-js library we need to import two more things from the lib's directory named chainTypes
& makeBitMaskFilter
. one of them carries the index of operations as how they are viewed on the blockchain and other being a function to create a BitMask of those indexes hence returning new parameters.
const hive = require("@hiveio/hive-js");
const chainTypes = require("@hiveio/hive-js/lib/auth/serializer/src/ChainTypes");
const makeBitMaskFilter = require("@hiveio/hive-js/lib/auth/serializer/src/makeBitMaskFilter");
Then to retrieve the new parameters, we choose which operations we need to filter from the history. we get the indexes of all operations from operation
property in chainTypes
and calling the makeBitMaskFilter
function like this
const op = chainTypes.operations;
const filter = makeBitMaskFilter([
op.curation_reward,
op.author_reward,
op.comment_reward,
op.liquidity_reward
]);
console.log(filter); // [ '8444249301319680', null ]
The above code will return an array of two elements which can be used as two last parameters of our request.
Continuing this we will now make the final adjustment of setting the rpc node to https://api.hive.blog, otherwise it will remove the new parameters.
hive.api.setOptions({url: "https://api.hive.blog"});
After that, as usual we can make a request with hive.api.getAccountHistory()
function but including new params like this.
hive.api.getAccountHistory(
'ali-h',
-1, 1000,
filter[0],
filter[1],
function(err, result) {
console.log(err, result);
});
Executing above code will return all the operations we used to create the BitMaskFilter in the last 1000 operations by the account ali-h
, i.e. curation, author, comment and liquidity rewards.
axios
to use this feature on them. Maybe in the next updates it will get out of the 'experimental' phase.This method (with hive-js) only works on https://api.hive.blog right now but there are other nodes that have already updated accordingly. so we can use any other library like
FULL CODE:
const hive = require("@hiveio/hive-js");
const chainTypes = require("@hiveio/hive-js/lib/auth/serializer/src/ChainTypes");
const makeBitMaskFilter = require("@hiveio/hive-js/lib/auth/serializer/src/makeBitMaskFilter");
const op = chainTypes.operations;
const filter = makeBitMaskFilter([
op.curation_reward,
op.author_reward,
op.comment_reward,
op.liquidity_reward
]);
hive.api.setOptions({url: "https://api.hive.blog"});
hive.api.getAccountHistory(
'ali-h',
-1, 1000,
filter[0],
filter[1],
function(err, result) {
console.log(err, result);
});
Thank you for sharing this with us, I'm sure this will come in handy in the future unless insert_favourite_hive_library_here does it for me so I can be lazy 😂 and sorry for the late comment!
Thank you for sharing this amazing post on HIVE!
non-profit curation initiative!Your content got selected by our fellow curator @tibfox & you just received a little thank you via an upvote from our
You will be featured in one of our recurring curation compilations and on our pinterest boards! Both are aiming to offer you a stage to widen your audience within and outside of the DIY scene of hive.
Join the official DIYHub community on HIVE and show us more of your amazing work and feel free to connect with us and other DIYers via our discord server: https://discord.io/diyhub!
If you want to support our goal to motivate other DIY/art/music/homesteading/... creators just delegate to us and earn 100% of your curation rewards!
Stay creative & hive on!