Spatial Domain
Spatial Domain can be broadly said as the image space. The image can be represented in the form of a 2D matrix where each element of the matrix represents pixel intensity. This state of 2D matrices which depict the intensity is called Spatial Domain. Manipulation of the pixel values are done here and the image is processed pixel by pixel.
Smoothing Filter
Image smoothing is a digital image processing technique that reduces and suppresses image noises. In the spatial domain, neighborhood averaging can generally be used to achieve the purpose of smoothing.
Average Filter
Average filtering is a method of smoothing images by reducing the amount of intensity variation between neighbouring pixels. The average filter works by moving through the image pixel by pixel, replacing each value with the average value of neighbouring pixels, including itself.
Program
Importing python libraries
import cv2
import numpy as np
import matplotlib.pyplot as plt
Reading the image
image = cv2.imread('badges.jpg')
image = cv2.cvtColor(image, cv2.COLOR_RGB2BGR)
plt.title('Original Image')
plt.imshow(image);
Converting the original image to grayscale
img = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
plt.title('Grayscale image')
plt.imshow(img, cmap='gray', vmin=0, vmax=255);
Number of rows and columns of the image
m, n = img.shape
print(f'Rows: {m}, Columns: {n}')
Developing Averaging filter mask of (3,3)
If the image is bigger in size then you can increase the mask to (5,5) or (7,7).
mask = np.ones([3,3], dtype=int)
mask = mask/9
print(mask)
Convolving the 3X3 mask over the image
newImage = np.zeros([m, n])
print(newImage)
Median Filter: Median filtering is a nonlinear process useful in reducing impulsive, or salt-and-pepper noise. In a median filter, a window slides along the image, and the median intensity value of the pixels within the window becomes the output intensity of the pixel being processed.
Traverse the image. For every 3X3 area, The median of the pixels are found and center pixel of the median is replaced by it.
Tools Used: Jupyter NoteBook
Python Libraries: OpenCV, numpy, matplotlib
Explanation
The original image is taken into the imread function of OpenCv and is displayed in the output with the help of matplotlib. The image is converted into grayscale for further process. The number of rows and columns of the image are obtained by the shape function which comes out to be 480 rows and 640 columns. A 3x3 averaging filter is generated containing all elements as 1 and it is divided by 9. The 3x3 mask created are convolved over the image. The blurred image is created through the imwrite() function of OpenCV and is stored in the workspace of jupyter notebook. It is observed that the filtered image is slightly blurred. If we increase the size of the averaging mask, more blurring can
be obtained. For median filtering the image is traversed for every 3x3 area. The median of the pixels is found out and the center is replaced by the median. The median filtered image is considerably enhanced with hardly any salt and pepper noise in it.
Conclusion:
- It is observed that the filtered image is slightly blurred. If we increase the size of the averaging mask, more blurring can be obtained.
- The median filtered image is considerably enhanced with hardly any salt and pepper noise in it.
References: Wikipedia, geeksforgeeks website, personal prepared notes.
Reference 1 Reference 2 Reference 3
Image Source: https://pixabay.com
I tried to make the code simple to understand and gave explanation of the various functions used in the program. Feel free to give your suggestions. Until we meet again in some other post, this is @biggestloser signing off....
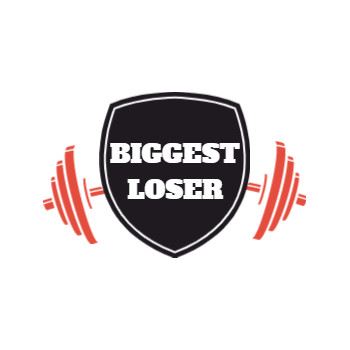
!discovery 15
Congratulations @biggestloser! You have completed the following achievement on the Hive blockchain and have been rewarded with new badge(s) :
Your next target is to reach 30 posts.
Your next target is to reach 500 upvotes.
Your next target is to reach 600 upvotes.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
This post was shared and voted inside the discord by the curators team of discovery-it
Join our community! hive-193212
Discovery-it is also a Witness, vote for us here
Delegate to us for passive income. Check our 80% fee-back Program