We POST 23 kinds of GOF design patterns implemented in javascript. Today, we will look at Abstract Factory Pattern for the second time.
(javascript로 구현하는 GOF 디자인 패턴 23가지를 POST 하고 있습니다. 오늘은 두번째로 추상 팩토리 패턴에 대하여 알아 보겠습니다.)
1. Overview
Provides an interface for creating interrelated or dependent objects, and creates concrete classes as subclasses without generating them directly.
If the class that makes up the object is the same in the authoring process and the implementation is different, the methods are abstracted by grouping them into specific groups.
(서로 연관된, 또는 의존하는 객체를 생성하기 위한 인터페이스를 제공하고, 구상 클래스를 직접 생성하지 않고 서브클래스로 생성합니다.
객체를 구성하는 클래스가 생성 공정이 동일하고 구현 내용이 다를경우 특정 그룹으로 묶어 메소드를 추상화 하여 사용합니다.)
2. UML Diagram
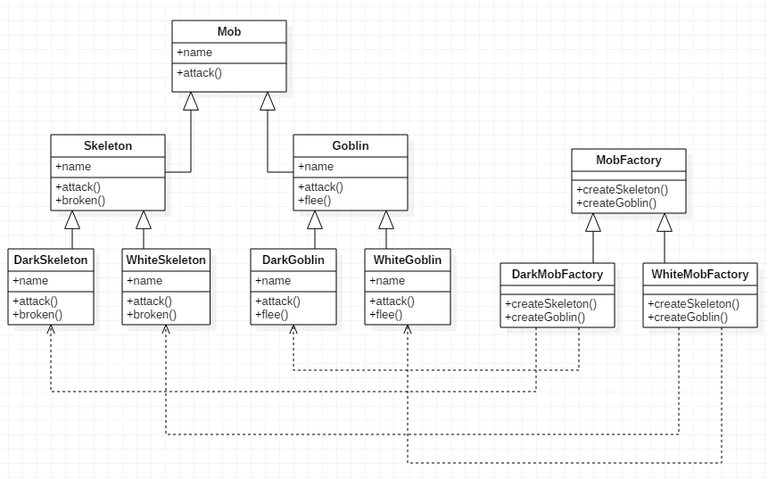
3. Example Code
The following code creates a concrete class at creation by binding Skeleton and Goblin to a specific group (Dark, White), abstracting the generation methods, and calling the same generation method for each group.
(다음 코드는 Skeleton 과 Goblin을 생성시 특정 그룹(Dark, White)으로 묶고, 생성 메소드를 추상화 하여 각 그룹별 동일한 생성 메소드를 호출 하여 구상 클래스를 생성 하는 예제 입니다.)
var Mob = function(){
this.name = "Mob";
};
Mob.prototype.attack = function(target) {
console.log('the ' + this.name + ' attacks the ' + target.name);
};
// Skeleton ////////////////////////////////////////////////////////
var Skeleton = function(){};
Skeleton.prototype = new Mob();
Skeleton.prototype.broken = function(){
console.log('the ' +this.name + ' is broken');
};
var DarkSkeleton = function(){};
DarkSkeleton.prototype = new Skeleton();
var WhiteSkeleton = function(){};
WhiteSkeleton.prototype = new Skeleton();
// Goblin ////////////////////////////////////////////////////////
var Goblin = function(){};
Goblin.prototype = new Mob();
Goblin.prototype.flee = function(){
console.log('the ' +this.name + ' flee');
};
var DarkGoblin = function(){};
DarkGoblin.prototype = new Goblin();
var WhiteGoblin = function(){};
WhiteGoblin.prototype = new Goblin();
// Factory ////////////////////////////////////////////////////////
var MobFactory = function(){
this.name = "MobFactory";
};
MobFactory.prototype.createSkeleton = function() {
console.log('Skeleton Creation');
};
MobFactory.prototype.createGoblin = function() {
console.log('Goblin Creation');
};
var DarkMobFactory = function(){
this.createSkeleton = function(){
return new DarkSkeleton();
};
this.createGoblin = function(){
return new DarkGoblin();
};
};
DarkMobFactory.prototype = new MobFactory();
var WhiteMobFactory = function(){
this.createSkeleton = function(){
return new WhiteSkeleton();
};
this.createGoblin = function(){
return new WhiteGoblin();
};
};
WhiteMobFactory.prototype = new MobFactory();
// client //////////////////////////////////////////////////////
// create
var darkMobFactory = new DarkMobFactory();
var darkSkeleton = darkMobFactory.createSkeleton();
darkSkeleton.name = "darkSkeleton1"
var darkGoblin = darkMobFactory.createGoblin();
darkGoblin.name = "darkGoblin1"
var whiteMobFactory = new WhiteMobFactory();
var whiteSkeleton = whiteMobFactory.createSkeleton();
whiteSkeleton.name = "whiteSkeleton1"
var whiteGoblin = whiteMobFactory.createGoblin();
whiteGoblin.name = "whiteGoblin1"
// action
darkSkeleton.attack(darkGoblin);
darkGoblin.flee();
whiteGoblin.attack(whiteSkeleton);
whiteSkeleton.broken();
4. Postscript
The abstract factory pattern is similar to the factory method pattern, but its purpose differs. You can use factory method patterns in abstract factory patterns. If you need to add a RedSkeleton, you will need to modify the factory class if you implement the factory method pattern only. However, if you implement the abstract factory pattern, you can implement it by adding RedMobFactory instead of modifying the class. Thus, using the abstract factory pattern, you can comply with the core principle of open-close principles (open for expansion, closed for modification).
(추상 팩토리 패턴은 팩토리 메소드 패턴과 유사하면서도 용도가 다르다는 것을 알 수 있습니다. 추상 팩토리 패턴에서 팩토리 메소드 패턴을 사용할 수 있습니다. 만약 RedSkeleton을 추가 해야할 상황이라면, 팩토리 메소드 패턴 만으로 구현을 할경우 팩토리 클래스를 수정을 해야 할 것입니다. 그러나 추상 팩토리 패턴으로 구현을 할경우 클래스를 수정 하는것이 아니라 RedMobFactory 를 추가 하여 구현할 수 있습니다. 따라서 추상 팩토리 패턴을 사용하면 핵심 원칙인 개방-폐쇄 원칙(확장에는 열려 있고, 수정에는 닫혀 있다)을 준수 할 수 있습니다.)
Cheer Up!
저는 예전에 자바 파란책으로 패턴 공부햇던게 생각나네요. kr-dev 태그도 이용해보시면 어떨까요?
좋은 의견 감사합니다.~ ^^
나도 나름 퍼블리션데.. 1도 못 알아듣겠어요 엉엉ㅠㅠ
언젠간 이해하는 날이 오길.. 그때까지 열심히 구경할게요ㅋㅋㅋ
내용이 좀 어렵죠 ㅎㅎ. 개발자들도 디자인패턴이란게 있는거만 알지 잘 사용하는사람은 드물어요. 저도 리마인드 할겸 글 올리고 있어요. 우선 패턴에 대해 다 정리 한다음 쉽게 이해할수 있도록 조금씩 다시 올릴게요.^^
This post received a 55% upvote from @krwhale thanks to @lsh1117! For more information, click here!
이 글은 @lsh1117님의 소중한 스팀/스팀달러를 지원 받아 55% 보팅 후 작성한 글입니다. 이 글에 대한 자세한 정보를 원하시면, click here!
뉴비는 언제나 응원!이에요.
팁! : 조사한바에 따르면. 텍스트가 공백제외 1000자 이상이면 지속적으로 사랑받는 포스트가 된다네요.
30.00% 보팅
현재 보상량 : [ 평균 - 2.83 / 3개 / 합계 : 8.48 ]
Congratulations @lsh1117! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
팁유 테스트 tip! 0.3
Hi @lsh1117! You have just received 0.3 SBD tip from @lsh1117!
@tipU - send tips by writing tip! in the comment, get share of the profit :)