1. Overview
A pattern that delegates the part that creates an object to a subclass.
Provides an interface for object creation and allows subclasses to determine which class instances to create.
(객체를 만들어내는 부분을 서브 클래스(Sub-Class)에 위임하는 패턴.
객체를 생성하기 위한 인터페이스를 제공하고 어떤 클래스의 인스턴스를 만들지는 서브클래스에서 결정하도록 합니다.)
2. UML diagram
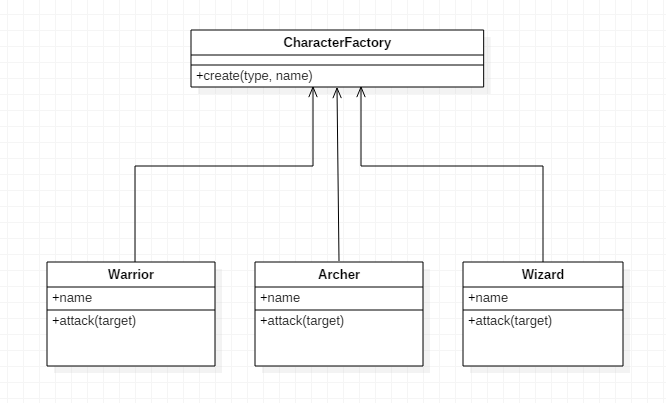
3. Example code
The example program below implements a factory for creating character based on the Diablo game. It does not generate any character on the client, but is a program that sends information to the factory to generate the character and generates the character in the factory.
(아래의 예제 프로그램은 디아블로 게임을 소재로 캐릭터를 만드는 공장을 구현 하였습니다. 클라이언트 에서 캐릭터를 생성 하지 않고, 캐릭터 생성을 위한 정보를 공장에 전달하고 공장에서 캐릭터를 생성하도록 구현한 프로그램 입니다.)
var Warrior =function(options) {
this.name = options.name;
};
Warrior.prototype.attack = function(target) {
console.log('the ' +this.name + ' attacks the ' + target.name);
};
var Archer = function(options) {
this.name = options.name;
};
Archer.prototype.attack = function(target) {
console.log('the ' +this.name + ' attacks the ' + target.name);
};
var Wizard = function(options) {
this.name = options.name;
};
Wizard.prototype.attack = function(target) {
console.log('the ' +this.name + ' attacks the ' + target.name);
};
var characterFactory = (function() {
return {
create: function(type,name) {
var character = null;
if(type == "warrior"){
character = new Warrior({"name":name});
}
else if(type == "archer"){
character = new Archer({"name":name});
}
else if(type == "wizard"){
character = new Wizard({"name":name});
}
return character;
}
}
})();
var barbarian = characterFactory.create("warrior","baba");
var amazon = characterFactory.create("archer","ama");
var sorceress = characterFactory.create("wizard","soso");
barbarian.attack(amazon);
amazon.attack(sorceress);
sorceress.attack(barbarian);
4. Postscript
The Factory pattern makes it easier to access interface code than the implementation, removes code that implements the instance from the client class, makes the code more powerful, and lowers the connection.
(Factory pattern은 구현체 보다는 인터페이스 코드 접근에 좀더 용의하게 해주며, 클라이언트 클래스로부터 인스턴스를 구현하는 코드를 떼어내서, 코드를 더욱 탄탄하게 하고 결합도를 나춥니다.)
Cheer Up!
@lsh1117 got you a $1.67 @minnowbooster upgoat, nice! (Image: pixabay.com)
Want a boost? Click here to read more!
Congratulations @lsh1117! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
This post received a 55% upvote from @krwhale thanks to @lsh1117! For more information, click here!
이 글은 @lsh1117님의 소중한 스팀/스팀달러를 지원 받아 55% 보팅 후 작성한 글입니다. 이 글에 대한 자세한 정보를 원하시면, click here!
뉴비는 언제나 환영!이에요.
팁! : 전자화폐 관련글은 cryptocurrency태그를 이용하면 좋아요.
1.00% 보팅
현재 보상량 : [ 평균 - 1.65 / 5개 / 합계 : 8.23 ]