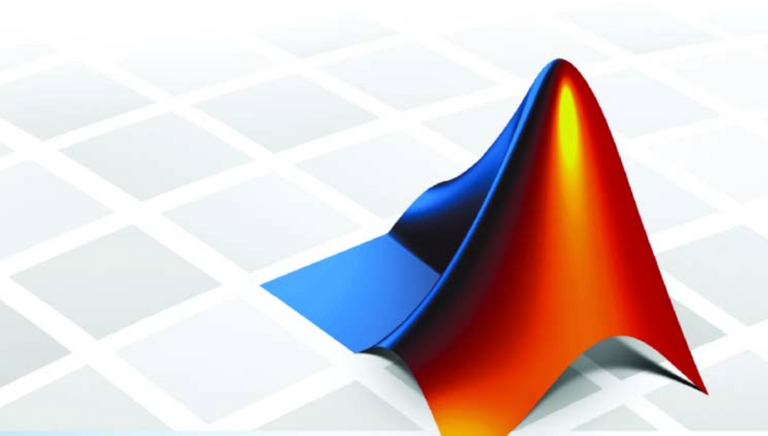
MATLAB is one of my favourite programming languages. It has incredibly simple syntax (notation), yet remains exceedingly powerful. It is also great at visualising work, and a very solid entry into the field of programming if you have never learnt another language before due to its intuitive nature. Further, the glass power ceiling in MATLAB is very high, meaning it can just as easily retrieve and plot the prices of cryptocurrency over time as it can model incredibly complex systems or graphics.
I was first introduced to MATLAB during my undergraduate studies in mechanical engineering, and have now spent a significant fraction of my life programming in it. In fact, the PhD in biomedical engineering I am currently pursuing uses MATLAB as its primary tool for solving complex models of brain function. I generate the mathematical models for whatever system I am investigating, and MATLAB is used to simulate the behaviour of this system over time. I understand that this may seem like an overly complex application for a beginner, so here is an example that takes about 10 minutes to program of some particles trapped in a box:
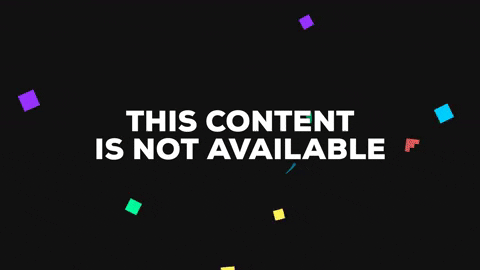
You can also do things like model an entire galaxy. Every one of the dots below is a star, all orbiting a black hole at the centre of the galaxy:
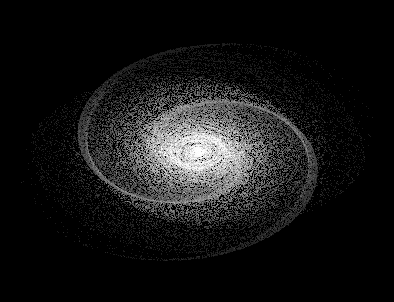
Before we get started with MATLAB, you will need to get yourself a copy of the software. Most research institutions offer free licence access to their students, and depending on what country you are in MATLAB offers free licences for you to practice the language in, provided you do not do any research or make money from it. I will assume you are able to get your hands on a copy of the software. It does not matter what edition you are using, as the syntax is 99.99% the same, but I will be running version 2014b.
When you load MATLAB, you will be greeted with a window like this:

The left hand pane is the command window, where we execute code live. The central pane is the editor, where we can write code to be saved and executed later. To begin, we will focus on the command window.
Basic Arithmetic
You can enter commands to perform basic arithmetical calculations straight into the Command Window in much the same way as you would on a calculator. For example, at the command prompt type the following and press Enter (note that the >> is shown for completeness and should not be typed):
>> 27 + 54
Matlab will calculate the result and show the answer in the Command Window:
>> ans =
81
You use the following arithmetical operators for addition, subtraction, multiplication and division:
- Use + for addition
- Use - for subtraction
- Use * (the asterisk) for multiplication
- Use / (forward slash) for division
Try the following in the command window:
>> 27 - 54
>> 27 * 54
>> 27/54
You can repeat commands by using the up arrow (↑) to take you back through the previous commands you have typed. Note that it does not matter if there are spaces between the numbers and arithmatical operators, as MATLAB will not incorporate these when interpreting your command.
The ^ symbol (called the carat) is the exponentiation (power) operator. For example:
>> 3^4
would yield:
>> ans =
81
Order of Operations
You may recall from high school that there is an order of operations when using the arithmetic operators (+, -, ×, ÷, etc). You will have encountered this when you do calculations on your calculator too. Matlab has an equivalent order of precedence for its arithmetical operators.
- Brackets
- Exponentiation
- Division and Multiplication
- Addition and Subtraction
The acronym BEDMAS is sometimes used to help to remember this ordering. Anything inside brackets happens first, then exponents (powers), then division and multiplication, finally addition and subtraction. As a result, the Matlab calculation
>> 2 x 3 + 10/2
returns
>> ans =
11
If two or more operations with the same precedence level follow each other, Matlab works through that bit of the calculation from left to right. Try the following and confirm what Matlab is doing for yourself:
>> 30/3*5
>> 2^3^4
Useful Functions
A very common operation is finding the square root of a number. You could use the power operator (for example, 4^0.5 would be 4½ which is 4–√4, but Matlab also provides a built-in sqrt function. In programming a function is similar to, but not quite the same as, a mathematical function. A mathematical function takes one or more inputs and returns a single output. Matlab functions can take zero or more inputs and return zero or more outputs. Matlab provides many built-in functions to help to achieve common programming tasks.
The sqrt function needs only one input, the number that you want to obtain the square root of. Try the following command in Matlab and you will see the answer returned is 13.
>> sqrt(169)
- The number 169 is the value input to the sqrt function
- The syntax for using ('calling') a function is to have the function name followed by the input value(s) enclosed in a pair of round brackets. The round brackets tell Matlab what to use as the input to the function.
One of the best things about Matlab is the extremely good documentation. The documentation includes:
- How to use the built-in functions and what they do
- Ways to search to find if there is a built-in function that does what you need
- Coding examples
- Helpful hints
- Suggestions about similar or alternative functions
- ... and much more
If you know the name of the function that you want you can get help in the Command Window. For example, try typing the following to bring up the help documentation on the sqrt function:
>> help sqrt
Floating Point Numbers
Knowing a little about how numbers are represented within Matlab will help you to understand some of important aspects of Matlab programming. The most important point to note is that not all numbers can be represented accurately. Matlab (like any computer-based calculation mechanism) only has a limited amount of memory in which store its representation of a number. By default, numeric data will be stored in what is called "double precision" format, using 8 bytes of memory (and, internally, Matlab uses a binary representation, which also determines what can and cannot be accurately represented).
The good news is that even though most numbers cannot be represented accurately in Matlab, most of the time you will not be aware of the issue unless your code depends on the result of a calculation being exactly equal to something else. This is relevant when using logical operators, which are a more advanced topic.
Actual Programming
So far you have been treating Matlab like a calculator. You have been entering expressions into the Command Window and Matlab has been calculating and displaying the result. This helps you to learn some of the basics about how Matlab works, but it is not programming. To learn to program with Matlab we will start with the idea of a variable. In programming, a variable has a name (an identifier) and a value. A variable is assigned a value using the = operator (aka the assignment operator, for obvious reasons). For example, the following Matlab statement assigns the value 3 to a variable named myFirstVariable:
>> myFirstVariable = 3
Type this statement into the Command Window and press Enter. You should see the following echoed in the Command Window:
myFirstVariable =
3
Look at your Matlab Workspace (usually, on the right of your Command Window). You will see that (in addition, possibly, to ans), your Workspace contains the variable myFirstVariable, with value 3. You have actually been interacting with variables already this tutorial. Whenever you entered a calculation into the Command Window, the result was assigned, invisibly, to the variable ans. That is why the variable name ans, with the result (answer) for the last calculation you did, may appear in your Workspace.
Scripts
You have seen how you can find and repeat previous commands in the Command Window using the up arrow, but this is clunky and impractical for a longer series of commands. The next level up is a script file. A script file contains a sequence of commands. When the script is run, all the commands in the sequence are executed, in the order that they are given. It is easy to rerun scripts, including changing some or all of the values of the variables and then rerunning the script. It is also easy to build up the script step by step, rerunning as you add each additional part, until you have completed the whole task. Open a new script by clicking the 'New Script' icon in the Matlab toolstrip:

Type the following lines into your new script:
Steemians = 600
Icecreams = 1200
IcecreamsPerSteemian = Steemians/Icecreams
These are just the same lines you just used for a Command Window exercise, but this time the Command Window prompt >> is omitted, to indicate that the lines are not just typed directly into the Command Window. To save the script, click the Save button on the Toolstrip in the Editor window (make sure that you are looking in the Editor window not the main Matlab window).
You will need to pick a name for your script. As with variable names, there are some rules to follow:
- You cannot have spaces in script names.
- You can have numbers in the script names but the name cannot begin with a number.
- The underscore "_" is the only non-alphanumeric character allowed in the script name.
- Use informative names that tell you about the purpose of the script.
Finally, you'll get to run your script. Click on the Run icon in the Editor window:
And you will see that everyone gets 2 icecreams!
I hope you learnt something useful from this very basic introduction into the world of MATLAB. There are many programming languages out there, and it can be tricky to pick the right one for the job you want to do. If you have never learnt a language before though, anywhere is a good start.
Content credit:
Banner, Mathworks
Footer, @me-shell
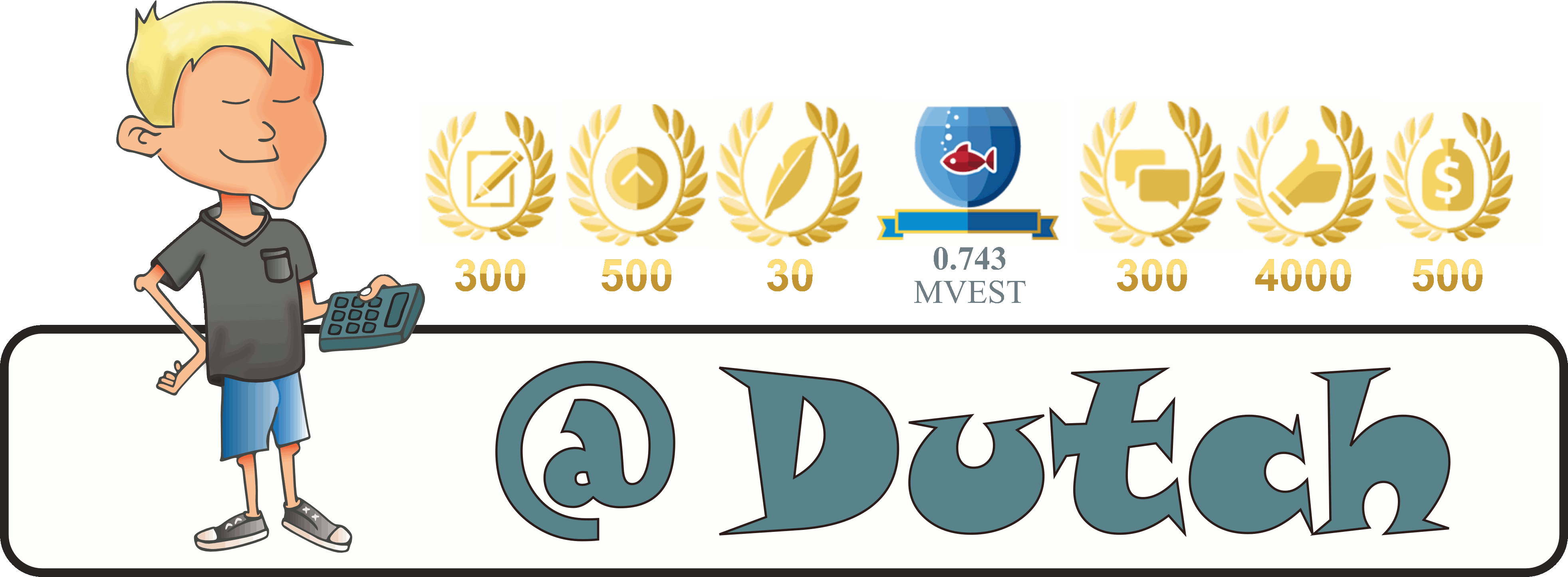
Nice program, but i have a question. I am a very very beginner in programming. What can you actual do with MATLAB, games, scripts, ?
Games, certainly. I would not use MATLAB for any intense games, but it is very capable for writing boardgames, text adventures (Zork, if you remember that gem), and other games with low graphics requirements. That beig said, people have made racing games in MATLAB - but only to prove a point, not because it was the best choice of software.
From being an absolute beginner, you could learn to program chess in MATLAB or Python comfortably within a week.
MATLAB is really good for doing cool stuff like neural network learning. This is where an AI essentially educates itself by trail and error. Here is an AI learning to walk:
None of these creatures were taught how to walk. They were essentially given muscles, and developed their gaits on their own. Some actually discovered - entirely autonomously - that hopping is more efficient than walking!
Thats really interesting, i think i will start doing this.
I learned just enough MATLAB back in the 90s to realize that I do not much enjoy programming. Have you used the free version, Octave?
I have not - the only company I worked for before returning to academics to do a doctorate actually paid the insane MATLAB license fee. I have heard Octave is nearly identical though.
The student version of MATLAB is sort of reasonable, $-wise, but yeah, it's almost as costly as Mathematica. Insane is the right word.
Have you read Wolfram's opus?
http://blog.stephenwolfram.com/2017/05/a-new-kind-of-science-a-15-year-view/
super
Though I have been using MATLAB for long, I am new to machine learning- SVM. I am attempting to do Machine Learning in MATLAB, I kind of think its not best choice but my advisor wants me to do it in MATLAB. Would you suggest me to use MATLAB or other platform like R or Python?
Ah awesome, just followed you and I hope that you will post more about MATLAB. I am using MATLAB at work and maybe I can learn something new from your posts.
Thank you - I appreciate the feedback. =)
If you are already using MATLAB I think my target audience experience level is far too low right now. I would be happy to cover some advanced features though.
MATLAB also stands for Matrix Laboratory as it is good for dealing with matrices, other linear algebra topics and math in general.
I knew a PhD Student from a Psychology department who specialized in neuroscience and machine learning. He used MATLAB (I think).
I no longer remember much of MATLAB as I use R and (sometimes) Python.
R is a language designed primarily for statistics right? We have a girl in the office who does statistical analysis of our models, which is important to work out if our results are actually statistically significant. I think she uses R.
Yes, R was primarily designed for statistics, math (linear algebra) and probability. As of late there has been some very good contributors to R that have made very useful packages for data visualization, data wrangling, data cleaning, machine learning tools, text mining, compatability to C++, SQL, etc.
For the person in your office I would think it is very likely she would use R (RStudio). The next choice would be SAS.
Great! I also use Matlab a lot, for my undergraduate physics studies. I have never liked it, because I have always been sort of dazzled with Mathematica. I met Mathematica way before, and still find it´s mathematical and programming capabilities far greater. And it´s graphics, for sure.
But, like I said, it might be just my impression, since I don´t have a good command of neither.
Great post, MATLAB has always seemed interesting to pick up but I've never gotten around to it. Seems like I should start playing with it for a bit.
Woo MATLAB! Many an hour was spent in MATLAB during university. For those who don't have a copy of it and don't want to get it from more questionable sources the best alternative I've used to date is Spyder which is python based and comes with the Anaconda python distribution.
tôi dùng chúng trong việc lập trình ra phần mềm y tế ? bạn có biết lĩnh vực này không