Today, I bring to you a solution to the second EulerProject problem. Without further ado, let us examine my solution.
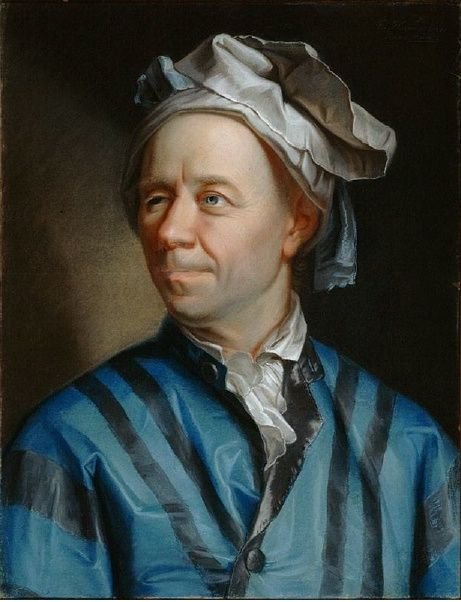
Let us first enjoy this marvellous painting of the man himself, Leonhard Euler
The Problem
Each new term in the Fibonacci sequence is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be:
1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
By considering the terms in the Fibonacci sequence whose values do not exceed four million, find the sum of the even-valued terms.
The Solution
#include <stdio.h>
#define MAX_FIB 4000000
int main(void) {
int a = 1, b = 1, c = 1, sum = 0;
while (c <= MAX_FIB) {
if (c % 2 == 0)
sum += c;
c = a + b;
a = b;
b = c;
}
printf("\nSum: %d\n", sum);
return 0;
}
You may have heard of the Fibonacci sequence and for good reason. Fibonacci was a very prominent mathematician whose theories have fuelled many modern mathematical theories. For example, the golden ratio: if you take two successive numbers from the Fibonacci sequence and look at the ratio between them, you will find that it is very close to the golden ratio.
But enough about math history, let's continue with the problem. What this code does is firstly define a constant (to improve readability) that is the number that should not be passed, as stated in the problem.
Secondly, it defines four variables, one for storing the total sum of the numbers, two variables for storing two fibonacci numbers and a final variable for storing the result of the two previous numbers - the newest Fibonacci number.
Thirdly, the program uses the modulus operator to check whether the newest Fibonacci number is even, as stated in the problem. It does this by returning the remainder of a division. Thus, if the remainder of the number divided by two is 0, this means the number divides perfectly by two which means that it is as even number.
Finally, it updates the variables to move up a step within the Fibonacci sequence and adds the current number to the sum
variable. It then outputs the sum
variable.
I ran this program and surprisingly, it worked first time and output 4613732
. I then put this number into the EulerProject website and it showed this:
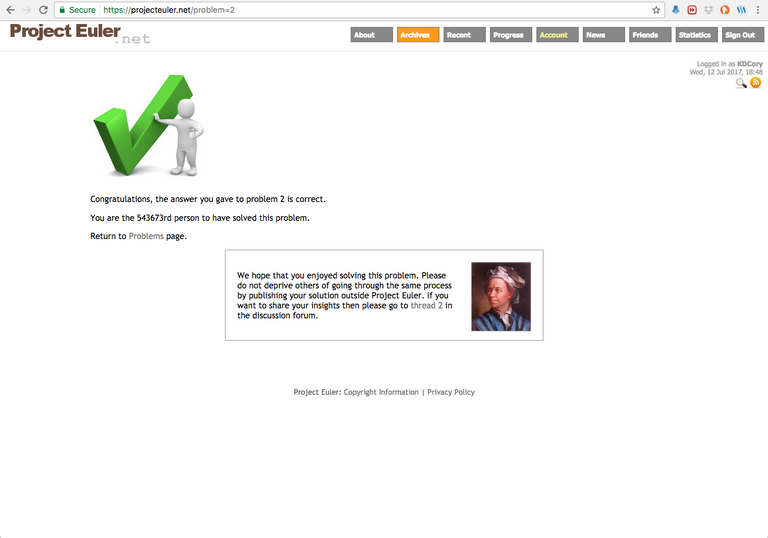
As you can see, the program output the correct answer
That is all for today, folks, I hope you have found this somewhat useful. Expect the next solution within the next few days; and until the, have a good one :)
Very nice, I'd like to see you try a little bit harder problem. I challenge you to do #14 next. I love doing the problems on that website, they're quite a bit of fun and good practice.
Thanks :) I know these are the small-fry problems but it'd bug me if I didn't do them in order. I'm being worked to the bone at an internship right now, but that ends tomorrow and then I shall be posting a problem daily. I completed about 200 of them a year or so ago but I'm a bit rusty on the good ol' mathematics so I'm going through 'em again aha. Thanks for your comment though!
Congratulations! This post has been upvoted from the communal account, @minnowsupport, by Mormegil from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, theprophet0, and someguy123. The goal is to help Steemit grow by supporting Minnows and creating a social network. Please find us in the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you like what we're doing please upvote this comment so we can continue to build the community account that's supporting all members.