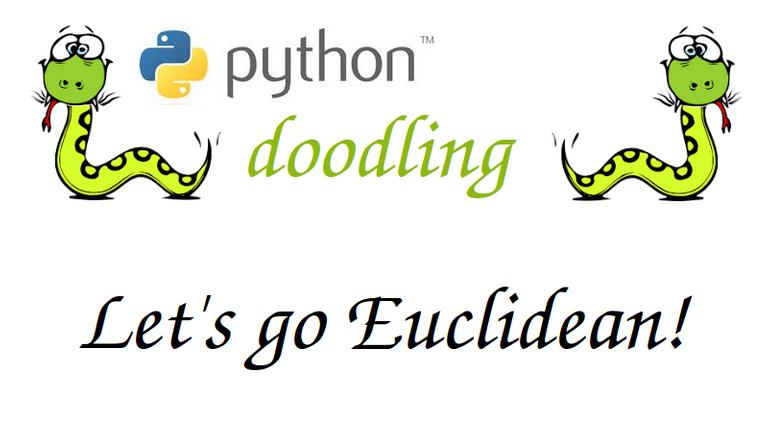
I start to discover Python richness. Python programming language is fine example how open source projects can do great things, when community gathers around some useful field of science. Programming is for sure an important science and knowledge enabling us harvest a power of our computers more efficiently.
There are tons of libraries and open source applications written in Python. I start my first Python doodle with numpy library. It implement linear algebra functions operations on vectors and matrix for faster and easier calculation.
Linear algebra is very important base knowledge to master some more complex concepts as neural networks and deep learning algorithms which are very popular now days.
So start my doodle with numpy.linalg.det() function which calculate a determinant of a matrix. Code speak for it self and is well documented.
import numpy as np
def fLineIntersect2d(line1, line2):
"""Function calculate intersection between lines line1 and
line2 and returns tuple of coordinates (x y) of
intersection point. If there is no intersection (lines
are parallel) then point (0, 0) is returned.
It uses determinants to calculate intersection point,
source: https://en.wikipedia.org/wiki/Line%E2%80%93line_intersection
"""
p1, p2 = line1[0], line1[1] # line1 is a list of two points
p3, p4 = line2[0], line2[1]
x1, y1 = p1[0], p1[1] # point is a list of to float
x2, y2 = p2[0], p2[1] # representing x and y coordinates
# of point in plane
x3, y3 = p3[0], p3[1]
x4, y4 = p4[0], p4[1]
# denominator determinant of four
aDeterminant = np.linalg.det(
[[np.linalg.det([ # points ob both lines.
[x1, 1], # If this value is zero this indicate
[x2, 1]]), # that there is no intersection,
np.linalg.det([ # lines are parallel.
[y1, 1],
[y2, 1]])],
[np.linalg.det([
[x3, 1],
[x4, 1]]),
np.linalg.det([
[y3, 1],
[y4, 1]])]
]
)
print('Determinant: ', aDeterminant)
# initial value for intersection point
intersection = (0, 0)
if aDeterminant > 0:
# calculating line intersection (px, py)
px = np.linalg.det(
[[np.linalg.det([
[x1, y1],
[x2, y2]]),
np.linalg.det([
[x1, 1],
[x2, 1]])],
[np.linalg.det([
[x3, y3],
[x4, y4]]),
np.linalg.det([
[x3, 1],
[x4, 1]])]
]
) / aDeterminant
py = np.linalg.det(
[[np.linalg.det([
[x1, y1],
[x2, y2]]),
np.linalg.det([
[y1, 1],
[y2, 1]])],
[np.linalg.det([
[x3, y3],
[x4, y4]]),
np.linalg.det([
[y3, 1],
[y4, 1]])]
]
) / aDeterminant
intersection = (px, py)
# function return intersection point
return intersection
def fTest():
"""Test function for testing correct implementation of
function fLineIntersect2d().
"""
line1 = np.array([[0.0, 0.0], [3.0, 3.0]])
line2 = np.array([[2.0, 0.0], [0.0, 2.0]])
print('intersection:')
print(fLineIntersect2d(line1, line2))
# intersection must be: (1.0, 1.0)
line1 = np.array([[10.0, 1.0], [-3.0, -4.0]])
line2 = np.array([[1.0, 1.0], [9.0, -3.0]])
print('intersection:')
print(fLineIntersect2d(line1, line2))
# intersecction must be: (4.9130, -0.9565,)
To test code save code into euclidgeom.py file, start python interpreter and load this file as is showed below. I use Python 3.6 Anaconda distribution, because it comes with a ton of very useful libraries installed (installation is trivial, check here @)
>>> import euclidgeom as e
>>> e.fTest()
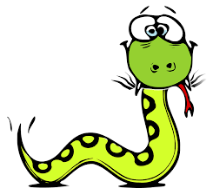
Fellow Steemians!
Hope you enjoy In this Python code snippets!
Hopefully, they brig some new ideas and deliver some useful knowledge. If you like them, join me as my follower and I promise more stuff for sure
Questions, suggestions are welcome in comments and I'm fully open for a discussion.
Dear @tombort
I believe its good people and you've got the high concern of the poor like us. I like your post and it's not easy. I apologize if I have commented differently with your post, I believe you do understand and I believe your kindness.
I need your help, my life was in debt lilit. May I know, where should I ask for help. I don't have a job in the country and to this day I have no costs to get married.
Sorry my impudence, kan has pitted the unfortunate fate of me in your post
If you are willing to pay a visit to my blog lah, with all due respect I thank you.
Save poor countries
Save humanity and the future of the children.
Yes, I really don't like that your comment is out of topic!
But ff you can produce some interesting stuff in your local environment, that could be sold on e-commerce platforms this is a good start to earn some money for survive. Try to sell something on https://www.peerhub.com. People already buy stuff for SBD and Steem there. Check it out and find your opportunities. One day you will remember my advice.
Be good!
p.s. Gather knowledge and be innovative!