Hello Steemit,
have you ever asked from where other Steemians with a high Vote-Weight got their SteemPower delegated?
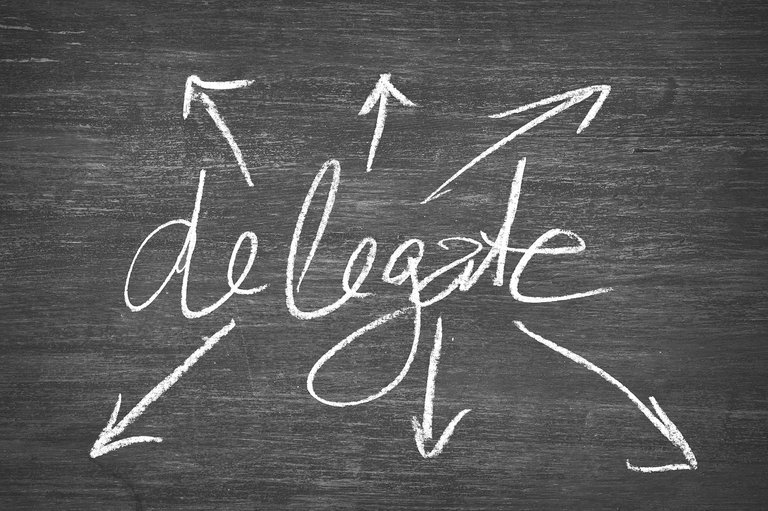
SteemData
I developed 2 python scripts for SteemData by @furion to find answers to this question. All you need is to install @furions steemdata python libarys, for details check https://steemdata.com/guide.
delegator.py - To which Accounts were SteemPower delegated?
In case you want to know, to which accounts a certain account has delegated SteemPower, just use the delegator.py script:
from steemdata import SteemData
from collections import Counter
import sys
s = SteemData()
delegations = s.Operations.find( {
'$and': [
{'type' : 'delegate_vesting_shares'},
{'delegator': sys.argv[1]}
]
} )
delegation_map = dict()
for delegation in delegations:
delegatee = str(delegation['delegatee'])
vestings = delegation['vesting_shares']
amount = vestings['amount']
delegation_map[delegatee] = amount
i=1
for key in sorted(delegation_map):
if delegation_map[key] > 0:
print('|' + str(i) + '|' + key + '|' + str(delegation_map[key])+ '|')
i += 1
Copy the script into a new text file called delegator.py and save it. You can run the script from the command line and provide an account name.
# python3 delegator.py twinner
The script will output a list of all accounts that got a SteemPower delegation from the given account name. In this example it is the current list of my SP-delegations I did 4 weeks ago to support new german users.
Number | Account | Delegated Vests |
---|---|---|
1 | acid78 | 941666.666667 |
2 | adamicelli | 1040622.916667 |
3 | addictedtophotos | 1010416.666667 |
4 | adelheid | 1040625.0 |
5 | ahinga | 1027817.805383 |
6 | alexmicrocontrol | 1040618.75 |
7 | alexvan | 3093750.0 |
8 | angrezi | 1040625.0 |
9 | arbeitssuchend | 1033171.84265 |
10 | argekunst | 1005977.083333 |
11 | artemisia | 1019887.5 |
12 | artpoet | 66302.277433 |
13 | austrobot | 999597.916667 |
14 | beatminister | 890755.693582 |
15 | beers | 200000.0 |
16 | besteulz | 834285.416667 |
17 | biggi | 910343.6853 |
18 | bluchr | 1040625.0 |
19 | bluepeaches | 1040625.0 |
20 | blueperegrina | 985416.666667 |
21 | brickster | 1027883.333333 |
22 | brotagnist | 1040595.833333 |
23 | bussdee | 1040625.0 |
24 | chancenbuffet | 1040625.0 |
25 | cobalus | 2939129.166667 |
26 | cornflakes | 1034161.490683 |
27 | creative-life | 1030493.75 |
28 | dehenne | 1019712.5 |
29 | denniskoray | 871910.973085 |
30 | derboersennerd | 997766.666667 |
31 | detlev | 538820.833333 |
32 | dswigle | 1024675.0 |
33 | earnfast | 1040625.0 |
34 | eisenbart | 1033708.074534 |
35 | elainetheinsane | 921950.310559 |
36 | elbiasto | 1040625.0 |
37 | enidan | 1040625.0 |
38 | ergeko | 982062.111801 |
39 | ethnoly | 265050.0 |
40 | felix.herrmann | 759575.0 |
41 | flauschi | 1040393.75 |
42 | flauwy | 952561.076605 |
43 | fraenk | 536456.25 |
44 | germancook | 1022083.333333 |
45 | geschichten | 313027.083333 |
46 | hausbau | 1040625.0 |
47 | hintenberg | 485052.083333 |
48 | ilsignore | 1040625.0 |
49 | irrer-ivan | 1034097.308489 |
50 | jamjamfood | 942710.416667 |
51 | jan-bensky | 1040625.0 |
52 | jeanpi1908 | 937025.0 |
53 | jensm85 | 1005110.416667 |
54 | jogi | 1040622.916667 |
55 | kochmaster | 728620.833333 |
56 | konsumkind | 1040625.0 |
57 | kranoras | 4129366.666667 |
58 | laloelectrix | 891697.916667 |
59 | lcshaft | 881287.5 |
60 | lexcited | 1040625.0 |
61 | liboriotv | 737500.0 |
62 | ligrev | 429704.166667 |
63 | lillliputt | 1038077.083333 |
64 | liyopa | 1040625.0 |
65 | louisa | 989583.333333 |
66 | mabre | 1040625.0 |
67 | maikegrell | 586079.166667 |
68 | maltombo | 1040625.0 |
69 | manonlescaut | 1037500.0 |
70 | marcelloblanco | 872045.833333 |
71 | markdaniel | 1040625.0 |
72 | martin.the.chef | 565656.25 |
73 | matzep | 1040625.0 |
74 | me-do | 625000.0 |
75 | melaniemd | 1040625.0 |
76 | miketr | 1041666.666667 |
77 | mkt | 1040625.0 |
78 | moneyrace | 4050070.320579 |
79 | nacktepoesie | 1040625.0 |
80 | nexanymo | 1035262.5 |
81 | nicoletta | 4075979.166667 |
82 | nuoviso | 320645.833333 |
83 | nutschiii | 983333.333333 |
84 | olli0103 | 1040625.0 |
85 | ophelion | 957725.0 |
86 | patriciarok | 988160.416667 |
87 | pawos | 3566675.0 |
88 | pipurilla | 3606543.950362 |
89 | pluemacher | 1040625.0 |
90 | psywalker | 3761991.726991 |
91 | quocvietle | 983333.333333 |
92 | redtravels | 3475243.75 |
93 | ridi20 | 720254.658385 |
94 | rizatr | 1040625.0 |
95 | rootingrobert | 1040625.0 |
96 | roused | 1018189.583333 |
97 | rushpictures | 800045.833333 |
98 | sabine-reichert | 929166.666667 |
99 | skrzypietz | 920308.333333 |
100 | speedygonzales | 983208.333333 |
101 | spirits4you | 1039618.75 |
102 | stateless | 1040625.0 |
103 | steemchiller | 514583.333333 |
104 | steemigonzales | 1040379.166667 |
105 | steemornot | 1039727.083333 |
106 | steinzeit | 990758.333333 |
107 | talaslegraps | 1040625.0 |
108 | talaxy | 609483.333333 |
109 | tamy | 3798081.25 |
110 | tatjana.lackner | 724470.833333 |
111 | thecoach | 932469.979296 |
112 | themediumone | 1040622.916667 |
113 | thepe | 789675.0 |
114 | tryto | 1037412.5 |
115 | twinkleberry | 4050070.320579 |
116 | tzap90 | 1040625.0 |
117 | vandaze | 1040339.583333 |
118 | wolfoftrading | 1032056.25 |
delegatee.py - From where got an Account SteemPower delegated?
In case you want to know, from where an account got SteemPower delegated, just use the delegatee.py script:
from steemdata import SteemData
from collections import Counter
import sys
s = SteemData()
delegations = s.Operations.find( {
'$and': [
{'type' : 'delegate_vesting_shares'},
{'delegatee': sys.argv[1]}
]
} )
delegation_map = dict()
for delegation in delegations:
delegator = str(delegation['delegator'])
vestings = delegation['vesting_shares']
amount = vestings['amount']
delegation_map[delegator] = amount
i=1
for key in sorted(delegation_map):
if delegation_map[key] > 0:
print('|' + str(i) + '|' + key + '|' + str(delegation_map[key])+ '|')
i += 1
Copy the script into a new text file called delegatee.py and save it. You can run the script from the command line and provide an account name.
# python3 delegatee.py minnowsupport
In this example the script will output a list of all accounts that delegated SteemPower to @minnowsupport project by @aggroed.
Number | Account | Delegated Vests |
---|---|---|
1 | adrianobalan | 206917.617596 |
2 | agr8buzz | 24820.824672 |
3 | alexvan | 1039918.355446 |
4 | alphacore | 4135.383314 |
5 | ariane | 10332.674856 |
6 | ausbitbank | 2070650.598418 |
7 | benjojo | 186348907.260027 |
8 | buzzbeergeek | 206940.7942 |
9 | captain-nemo | 206940.7942 |
10 | chrissymchavez | 22415.0 |
11 | cosimo | 28933.102466 |
12 | crazybgadventure | 20679.029737 |
13 | crimsonclad | 26949.0 |
14 | cryptobeard | 10347.510802 |
15 | cryptofixer | 62056.810942 |
16 | cryptopie | 20665.477667 |
17 | danielsaori | 426754.0 |
18 | dber | 1033307.837437 |
19 | diebaasman | 103379.867576 |
20 | digitalking | 10340.348026 |
21 | discordiant | 103385.208857 |
22 | dogeking | 20663.208582 |
23 | drwom | 206734.831158 |
24 | dswigle | 41351.235236 |
25 | ejemai | 20688.898957 |
26 | eliel | 20680.915714 |
27 | errymil | 103470.399999 |
28 | ethical-ai | 1050000.0 |
29 | eturnerx | 1033175.881365 |
30 | everybitmatters | 103470.3971 |
31 | exavier | 104949.0 |
32 | fingersik | 63106.0 |
33 | gnocdepatat | 155087.294461 |
34 | gringalicious | 206801.353693 |
35 | grocko | 20680.663536 |
36 | groundcontrol | 20680.592689 |
37 | gyanibilli | 207998.115079 |
38 | haushinka | 20691.167768 |
39 | hitmeasap | 206949.787772 |
40 | hypexals-spiral | 209618.0 |
41 | iamjustincscott | 10300.0 |
42 | isaac.rodebush | 2067953.346083 |
43 | jaredcwillis | 206831.415654 |
44 | jassennessaj | 31021.086522 |
45 | jedau | 1033128.741758 |
46 | jeffjagoe | 208877.211049 |
47 | jesse2you | 51723.959573 |
48 | jocra | 1034414.986604 |
49 | joeyrocketfilms | 413680.411198 |
50 | jraysteem | 20668.229739 |
51 | jrhughes | 60949.0 |
52 | justcallmemyth | 103837.0 |
53 | kid4life | 20665.673503 |
54 | kryptokayden | 20685.732016 |
55 | krystle | 212746.0 |
56 | lances | 1034596.92104 |
57 | leyargoz | 10335.388685 |
58 | livingwaters | 20677.393856 |
59 | luke-skywalker | 41366.23758 |
60 | manishmike10 | 41382.506787 |
61 | matthewtiii | 207998.115079 |
62 | michelios | 2066658.529673 |
63 | moataz | 20689.626635 |
64 | morph | 20678.623159 |
65 | natra | 207998.115079 |
66 | necrophagist | 103470.3971 |
67 | neoxian | 10342075.0 |
68 | netuoso | 208984.686182 |
69 | nicnas | 208402.0 |
70 | nnnhhh | 206944.220255 |
71 | omar-hesham | 6202.834833 |
72 | paulag | 20689.712247 |
73 | pisolutionsmru | 103999.057539 |
74 | pratikchordia | 20680.861517 |
75 | r0nd0n | 207000.0 |
76 | raserrano | 10332.833146 |
77 | raymonjohnstone | 1035641.0 |
78 | realm | 20683.977777 |
79 | revelim | 20676.352879 |
80 | ribalinux | 82701.999676 |
81 | robertdurst10 | 22990.0 |
82 | romantic4 | 4132.896869 |
83 | rufaz | 20800.115079 |
84 | sammosk | 20672.049093 |
85 | sandstorm | 1034731.807862 |
86 | satfit | 62061.432543 |
87 | shawnfishbit | 206915.959014 |
88 | shellyduncan | 206852.185497 |
89 | siddartha | 162152.913824 |
90 | siersod | 103470.3971 |
91 | snubbermike | 2179988.115079 |
92 | somethingsubtle | 206906.540316 |
93 | soundwavesphoton | 20689.583829 |
94 | starke | 51666.726901 |
95 | stay9n0 | 50998.115079 |
96 | steemblake | 41374.801918 |
97 | steemface | 9429.0 |
98 | sulev | 2069407.942 |
99 | surprisebit | 20689.712247 |
100 | swelker101 | 24801.998226 |
101 | tamaralovelace | 103442.140673 |
102 | tech-trends | 20689.797861 |
103 | theblindsquirl | 206949.787772 |
104 | timcliff | 7233186.126111 |
105 | touchman | 2296131.218686 |
106 | tremendospercy | 206833.788368 |
107 | uniwhisp | 1034419.26668 |
108 | v4vapid | 1034703.971 |
109 | vallesleoruther | 20663.588915 |
110 | venuspcs | 2079980.115079 |
111 | vj1309 | 60000.679363 |
Thank you for reading!
In case you are interested in the delagtions of other accounts, just leave a comment and I will post it there.
cool script, great job looking more good stuff from ur side man
This post received a 4.2% upvote from @randowhale thanks to @abbasfxtm! For more information, click here!
Tolle Arbeit. Man muss mal von ganzen Herzen einfach mal Danke sagen. Was du für mich und hunderte andere machst und getan hast, all die Unterstützung !!! Sowas ist nicht selbstverständlich mein Freund. # header
Amen to that!
great script ! Its amazing what people do here to make data transparent ! Thanks for that !
Ich finde es einfach großartig, wie du Neulinge, sei es durch das Delegieren von Steempower, großzügige Upvotes oder sonstige Hilfestellungen unterstützt!
This is a really good tool! Thanks for sharing!
I will try it and explore :D
Upvoted and followed!
Thanks for sharing this scrript
As Always great and useful post
Keep the good work
Have a great and sunny day
Interesting
There is a post to check one's account delegation status:
https://steemit.com/lolitest/@elautomatico/sp-delegation-toolbox-20170725
Hehe, nice.
I used some logic just like this on the https://mspdelegator.herokuapp.com website to get the list of delegators for the @minnowsupport account.
It is always cool to see the different languages of the same type of script
Interesting! Thank you!
Das sind leider böhmische Dörfer für mich, auf jeden Fall ganz toll, wie du die Anfänger hier (wie auch mich) so großartig unter die Arme greifst. Vielen, vielen Dank!
Vielen Dank für diesen hilfreichen Post und deine Upvotes! Die Unterstützung bedeutet mir (und wahrscheinlich allen anderen auch) sehr viel. Aller Anfang ist schwer und bei so einer tollen Community, die sich gegenseitig unterstützt, macht es alles so viel mehr Spaß.
interessantes, sehr hildreiches Script ! Ist Python zum access der API besonders geeignet oder laufen solche Scripts ebenso gut mit PHP ? Wie sieht es mit der Datenmenge aus ? Viele Fragen :)
Bin am ueberlegen, ebenfalls Daten aus der API aufzubereiten, da ich das einfach nur klasse finde, solche Daten den Usern auf Steemt zur Verfügung zu stellen. Thumbs up und DANKE !
So wie ich es verstanden habe ist in der Steemdata python API schon ein MongoDB Treiber mit eingebunden, den man bei anderen Programmiersprachen noch manuell installieren müsste. Aber PHP oder Javascript sollten auch kein Problem darstellen.
@twinner you are my hero!!! :D :D
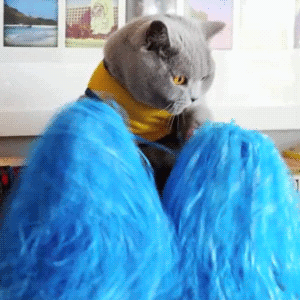
As an totally clueless newbie, one day I noticed you had generously delegated power to me. I was surprised and a bit overwhelmed. I didn't know what to do with it, so my approach was to very carefully look for posts that impressed me and upvote them.
Question: From your perspective as the granter, how would like the grantee to use the delegated power? Vote like crazy, vote cautiously, sit on it, or something else? What would be most beneficial for you?
Vote whatever you want :-)
Ich hatte mich irgendwann gewundert warum ich plötzlich so viel SteemPower hatte. Hab es aber erst vor paar Tagen bemerkt, dass ich dir das zu verdanken habe. Lieben Dank.
Wirklich wieder einmal mehr eine grandiose Arbeit, die du für die Steemit-Community geleistet hast @twinner (:
Vielen Dank dafür! Auch wenn du mir jetzt persönlich die Freude daran genommen hast, mühselig selber herauszufinden, wem ich meine delegierten SP zu verdanken haben (kommst ja eh nur du in Frage) :D
Very helpful. I hope someday soon such kind of data can be pulled by every user via simple frontend-features...
I hope it too.
Very interesting. Resteemed :-)
Thank you very much @lichtblick :-)
This is a good concept @twinner hwoever for those with enough to delegate..Hope to join soon
I have no idea what you are talking about, but you get my upvote, because of your support. My name is on that list! Juhuu! Thank You!!!
Thank you @blueperegrina.
any ideas?
telesti@starship:~/python$ python3 ./delegator.py enki74 Traceback (most recent call last): File "./delegator.py", line 1, in <module> from steemdata import SteemData ImportError: No module named 'steemdata' telesti@starship:~/python$ ./delegator.py enki74 from: can't read /var/mail/steemdata from: can't read /var/mail/collections ^C./delegator.py: line 5: syntax error near unexpected token `(' ./delegator.py: line 5: `s = SteemData()'
Hi enki74,
you need to install steemdata python libs first
See https://steemdata.com/guide
Nice work its really helpful and finger tips data.
Good article full of knowledge.
nice artikel
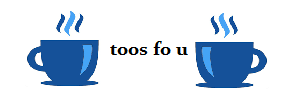
@fahrullah
Wow! You did good post my friend @twinner and interesting article! Look on my post! I posting very interesting cool journey to the pool! It's very cool : https://steemit.com/travel/@bugavi/super-tusa-in-the-pool-journey-to-the-pool-from-bugavi-swim-with-me
upvote and like it
Upvoted and RESTEEMED :)
thanks, exactly what i was looking for!
und danke fuer die delgation nochmal, habe bald schon fast genug eigene SP um den power slider aus eigener Kraft nutzen zu duerfen! Melde mich wenn's soweit ist.
Tolle Post twinner und Vielen Dank für den Ratschlag bei meine Post über Liechtenstein @johnadams
Great work, thank you. And great explanation.
I could just run that script in any computer and get the results? Does it need a specific operating system (linux, maybe)? does it need a direct connection to a specific server?
Nice scripts. Can you also show the date of the delegations?