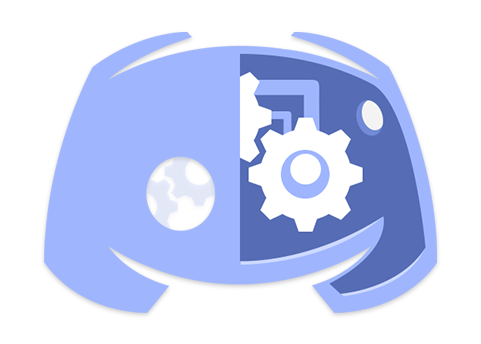
For a couple of days now i'v been active in discord channels, generally steem topics.
Then it hit me, why not make a serie about bot development in NodeJS.
I'm good with nodejs so that was my choice, there's plenty of APIs out there in other languages.
So what is an API?
- An API (application programming interface) are ready to use services that return data when used or let us developers push data to another application.
in example "steem.api.getAccounts([arrayOfUsers]) this steem function will return data about an array of users.
So what will you be learning in this cours?
- The basics of discord bot developement.
- How to get data from steem api.
- Create a Discord bot & give it a command that returns a msg in the channel.
- Get informations about steem accounts and return them.
Requirements:
-Nodejs & NPM.
-Discord.
-basic javascript.
Let's get started!
First of all start by installing Nodejs.
it's fearly easy you just go to officiel nodeJs website and download and install it locally from here
Then You open a terminal, navigate to the chosen repository and init(make a new project) with
npm init
leave all of the following as default like in this screenshot:
Now that You created your project open it in your preferred editor(i prefer Visuel studio Code).
What now?
- install the needed packages: in your CLI(command line interface)
npm install --save steem discord.js dotenv
- Now create a new file and call it server.js
This file will serve as our server code that will be our backend of our Discord bot. - Create also a .env & .gitignore files.
The .env file will have all our secrets and our confidential keys, tokens...
The .gitignore is a file where you put what files you don't want to include when pushing to git.
Now the boring part is over :D let's get to the code.
- Open server.js
paste in this code:
const Discord = require('discord.js') const steem = require('steem') const dotenv = require('dotenv') dotenv.config() const client = new Discord.Client() const prefix = process.env.PREFIX client.login(process.env.DISCORD_TOKEN) client.on('ready', () => { console.log(logged in as ${client.user.tag}!) }) client.on('message', (msg) => { if(!msg.author.bot){ msg.channel.send("hello " + msg.author.username) } })
Now go to Discord developer section and create a new application
Now go to bot in the left menu.
Click on Add bot
Button
Congrats the Discord Application is now a bot activated!
Now Click on copy to copy the bot access token
- A token is a secret key that gives permission to access to a certain application, there's many types of access tokens.
Now in the .env file paste in this code
CLIENT_TOKEN = Your access token OWNER = Your application Client ID PREFIX = $
Note: The OWNER(client ID) you can find it in the application, General Information Tab You just copy that.
Now if You run your code from the terminal using node server.js
and go to your server your bot will not be there even if the code has no errors.
Don't worry we got that covered ;)
https://discordapp.com/oauth2/authorize?&client_id=ClientId&scope=bot
In client_id="here you paste in your Client ID" (the same in your .env file)
And you open that URL in your browser. If your nodejs server is running you'll see that your bot will join your server.
If you write any message in your channel you'll see that your bot responds with "Hello username
"
Now let's go back to the server.js file we'r going to modify our function
client.on('message', (msg) => {
if(!msg.author.bot){
msg.channel.send("hello " + msg.author.username)
}
})
To:
client.on('message', (msg) => {
if(!msg.author.bot){
const args = msg.content.slice(prefix.length).split(/ +/)
const command = args.shift().toLowerCase()
if(msg.content.startsWith("$") && command == "info"){
if(args.length){
for(i=0;i<=args.length-1;i++){
let account = args[i]
console.log(account)
steem.api.getAccounts([account], function(err, steemian_info) {
console.log(err, steemian_info);
if (!err) {
for (let i = 0; i < steemian_info.length; ++ i) {
let profile = JSON.parse(steemian_info[0].json_metadata).profile
let reputation = steemian_info[i].reputation
let name = profile.name
var profile_image = profile.profile_image
var about = profile.about
let formatted_rep = steem.formatter.reputation(reputation);
//console.log(steemian_info[i].name + "'s Reputation is " + formatted_rep);
const embed = new Discord.RichEmbed()
.setAuthor(msg.author.username + " looked for " + name, msg.author.displayAvatarURL)
.setDescription("https://steemit.com/@" + steemian_info[0].name)
.setColor([77, 238, 22])
.setTitle("profile")
.addField("reputation", formatted_rep)
.addField("Description", about)
.setDescription("https://steemit.com/@" + steemian_info[0].name)
.setImage(profile_image)
msg.channel.send(embed)
}
} else {
log("Steem API Error: ", err);
}
});
}
}else{
msg.channel.send('enter a valid username')
}
}
}
})
ps:In your editor the code will be clearer.
Now run your server again (if you want to shut down the server just ctrl+c in the terminal alraidy running)
Reminder: node server.js
Now go test your bot in Discord, type $info mohamedsahbi zenkly
You'll see a display like this:
Like you see here you can even look for multiple profiles.
I'll leave some references you can check for more in depth reading and test as much as you like.
STEEMY
Steem.js
More on building Discord Bots:
https://gist.github.com/y21/a599ef74c8746341dbcbd32093a69eb8
Lastly i like to thank @zenkly for his amazing posts about discord bots that i tested and gave me the oppertunity to write something similar(updated version).
More to come in the next posts.
Spread the STEEM Love 😍 😍 😍
thank you man very instructive
Posted using Partiko Android
Thanks for the mention. I'm glad that my tutorial has been useful to you. Regards!
yep i hope you put in the good effort in many to come articles and i hope we can help developers on this great platform make great apps