This is the first post of a new series that will explain specific functionalities of the Steem-API and explain how to use them with SteemJ.
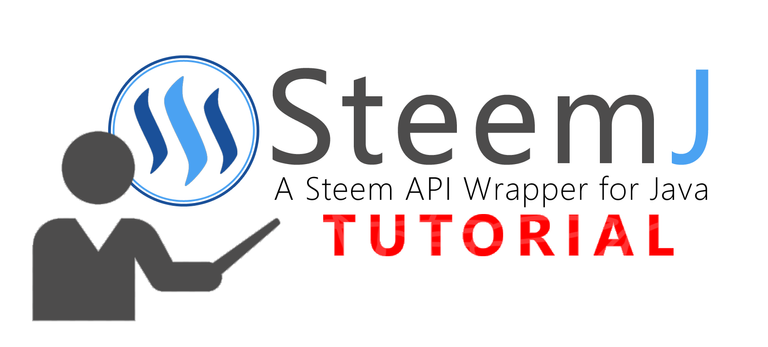
Source of used Icons: Iconfinder.com
Steem API / SteemJ Tutorial #1 - The follow_api
Hello Steemians!
for those of you who already use SteemJ, it is not a big surprise that there is quite a huge lack of documentation. At the beginning I thought that this is not a big deal if I keep using the original fields and methods as provided by Steem itself, but it also turned out that the original code has not that much comments and the Steem Dev Documentation is also not providing detailed information.
To make your life easier, to solve the lack of SteemJs documentation and to motivate myself for writing all this stuff, I've decided to create a Steem Tutorial series where I explain a specific part of the Steem API and show how to use/call those things with SteemJ.
One of the most requested things here on Steemit and also at GitHub was related to the Steem follow_api - So let's start with this.
Follow API
The follow_api covers all api calls related to blogs, feeds, and followers.
TOC
- getFollowers
- getFollowing
- getFollowCount
- getBlogEntries
- getBlog
- getFeedEntries
- getFeed
- getAccountReputations
- getRebloggedBy
- getBlogAuthors
getFollowers
Use this method to get a list of account names which the following
account is followed by.
Steem offers some filter creteria for this method. As usual, Steem allows to limit the number of results using the limit
parameter. Beside that it is also possible to differ between mutes and follows using the type
parameter. The last filter option is the startFollower
parameter which can be used to only receive accounts that the following
account followed or muted after he or she followed or muted the startFollower
account.
List<FollowApiObject> followers = steemApiWrapper.getFollowers(new AccountName("dez1337"),
new AccountName("good-karma"), FollowType.BLOG, (short) 10);
The sample above will return up to 10 account names dez1337 is followed by (FollowType.Blog
) since good-karma has followed dez1337.
List<FollowApiObject> followers = steemApiWrapper.getFollowers(new AccountName("dez1337"),
new AccountName(""), FollowType.BLOG, (short) 10);
The sample above will return up to 10 account names dez1337 is followed by.
getFollowing
Use this method to get a list of account names which the follower
account follows.
Steem offers some filter creteria for this method. As usual, Steem allows to limit the number of results using the limit
parameter. Beside that it is also possible to differ between mutes and follows using the type
parameter. The last filter option is the startFollowing
parameter which can be used to only receive accounts that the follower
account followed or muted after he or she followed or muted the startFollowing
account.
List<FollowApiObject> following = steemApiWrapper.getFollowing(new AccountName("dez1337"),
new AccountName("good-karma"), FollowType.BLOG, (short) 10);
The sample above will return up to 10 account names dez1337 has followed (FollowType.Blog
) after he followed good-karma.
List<FollowApiObject> following = steemApiWrapper.getFollowing(new AccountName("dez1337"),
new AccountName(""), FollowType.BLOG, (short) 10);
The sample above will return up to 10 account names dez1337 is following.
getFollowCount
Use this method to get the amount of accounts following the given account
and the number of accounts this account
follows.
FollowCountApiObject followCount = steemApiWrapper.getFollowCount(new AccountName("dez1337"));
The sample call above will return the number of accounts following dez1337 and the number of accounts dez1337 is following.
getBlogEntries
Use this method to get the blog entries of the given author
based on the given coniditions.
Each blog entry of an author
(resteemed or posted on his/her own) has an entryId
, while the entryId
starts with 0 for the first blog entry and is increment by 1 for each resteem or post of the author
.
Steem allows to use the entryId
as a search criteria: The first entry of the returned list is the blog entry with the given entryId
. Beside that, the limit
can be used to limit the number of results.
List<BlogEntry> blogEntries = steemApiWrapper.getBlogEntries(new AccountName("dez1337"), 5, (short) 2);
So if the method is called with entryId
set to 5 and the limit
is set to 2, the returned list will contain 2 entries: The first one is the blog entry with entryId
of 5, the second one has the entryId
4.
If the entryId
is set to 0, the first returned item will be the latest blog entry of the given author
.
List<BlogEntry> blogEntries = steemApiWrapper.getBlogEntries(new AccountName("dez1337"), 0, (short) 2);
So if a user has 50 blog entries and this method is called with an entryId
set to 0 and a limit
of 2, the returned list will contain the blog entries with the entryId
s 50 and 49.
getBlog
This method is like the getBlogEntries method, but instead of the author
and the permlink
, it contains the whole content of the post.
List<CommentBlogEntry> blog = steemApiWrapper.getBlog(new AccountName("dez1337"), 0, (short) 10);
The sample above returns the last 10 blog entries of dez1337 blog.
getFeedEntries
This method is like the getBlogEntries method, but instead of returning blog entries, the getFeedEntries
method returns the feed of the given account
.
List<FeedEntry> feedEntries = steemApiWrapper.getFeedEntries(new AccountName("dez1337"), 0, (short) 100);
So that the sample above returns the latest 100 posts of dez1337s feed.
getFeed
This method is like the getBlog method, but instead of returning a blog, the method will return the feed of the given account
.
List<CommentFeedEntry> feed = steemApiWrapper.getFeed(new AccountName("dez1337"), 0, (short) 100);
So that the sample above returns the latest 100 posts of dez1337s feed including the whole content of each post.
getAccountReputations
This next method can be used to get the reputation for one or more accounts.
The method requires an account name pattern and the number of results Steem should return.
List<AccountReputation> accountReputations = steemApiWrapper
.getAccountReputations(new AccountName("dez1337"), 0);
The method returns an account name that matches the most with the given account name pattern and the reputation of this account. The sample above would return the reputation from dez1337, because this account has a 100% match with the given account name pattern "dez1337".
List<AccountReputation> accountReputations = steemApiWrapper
.getAccountReputations(new AccountName("dez1337"), 1);
This sample would return the reputation for dez1337, but also add a second account dez243 to the result, because dez243 is the account that has the second most accordance with the given account name pattern.
getRebloggedBy
Use this method to find out, which user have resteemed a specific post.
The method requires the author of the post and its permanent link, so a method call could look like this:
List<AccountName> accountNames = steemApiWrapper.getRebloggedBy(new AccountName("dez1337"),
"steemj-v0-2-6-has-been-released-update-11");
The result of this call is a list of account names which have resteemed the given post.
getBlogAuthors
Use this method to find out, how many blog entries of which authors have been published by an account.
The method only requires an account name of the blog owner to analzse, so a method call could look like this.
List<PostsPerAuthorPair> blogAuthors = steemApiWrapper.getBlogAuthors(new AccountName("dez1337"));
And would return a list of pairs, while earch pair consists of an author name and the number of blog entries that dez1337 has resteemed from this author.
ausbitbank: 1
good-karma: 3
...
In this case two pairs have been returned, providing the information that dez1337 has currently resteemed one post from ausbitsbank in his blog history, and three posts from good-karma.
General information
What is SteemJ?
SteemJ is a project that allows you to communicate with a Steem node using Java. So far, the project supports most of the API calls and is also able to broadcast most of the common operation types. Further information can be found on GitHub.
How to add it to your project?
SteemJ binaries are pushed into the maven central repository and can be integrated with a bunch of build management tools like Maven. The Wiki provides a lot of examples for the most common build tools. If you do not use a build management tool you can download the binaries as described here.
Contribute
The project became quite big and there is still a lot to do. If you want to support the project simply clone the git repository and submit a pull request. I would really appreciate it =).
git clone https://github.com/marvin-we/steem-java-api-wrapper.git
Get in touch!
Most of my projects are pretty time consuming and I always try to provide some useful stuff to the community. What keeps me going for that is your feedback and your support. For that reason I would love to get some Feedback from you <3. Just contact me here on Steemit or ping me on GitHub.
Follow me | Follow @dez1337 |
---|---|
![]() | ![]() |
Thanks for reading and best regards,
@steemj
Thanks for SteemJ, I just found it and it is really useful! Keep it up with the tutorial series, I'm sure it will be useful to a lot of developers (me included) who can focus more on the UX :-)
Greate!
Wonderful tutorial. It makes it much easier to learn the API.
Hi @steemj,
Good job!
I'm looking for an API that allows me to create content (POSTs). I have been reading the documentation and it seems that we can only retrieve information or just create a comments or up-vote.
I wonder if you could point me to some API that allows creating POST in steemit.com.
Thanks,
@realskilled
You can find an informative article which includes an instructional video that details how to consume the STEEM API to post content to the Blockchain. See https://www.pmncoins.com/index.php?option=com_k2&view=item&id=318. When you finish your project tell us about it so we can publish and share with our users. Cheers. Good luck.
whats the most efficient way to check if I am following certain account?