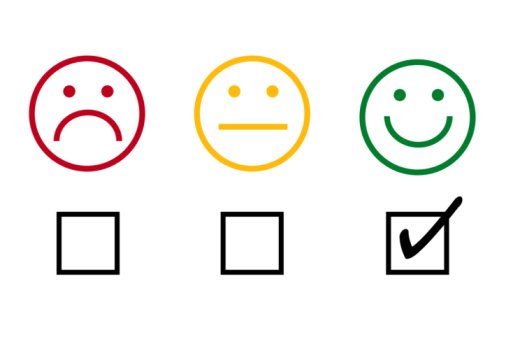
All you wanted to know about reputation
A lot of users found themselves confused about what reputation is, how it is computed, how it changes, which effect it has on what, ...
I have compiled here all the information I found about reputation and tried to make it easier for everyone to understand how it works on Steemit.
What is reputation for?
The reputation has two roles:
- It is an indicator that shows how “trusted or appreciated by the community” you are.
- It is a tool that prevent user with low reputation to harm other users.
How it works
Reputation points are calculated using the mathematical function Log base 10.
Here is a representation of this function. (note for the purists: I know the X-axis scale is not correct for reputation. I made it as is for simplicity)
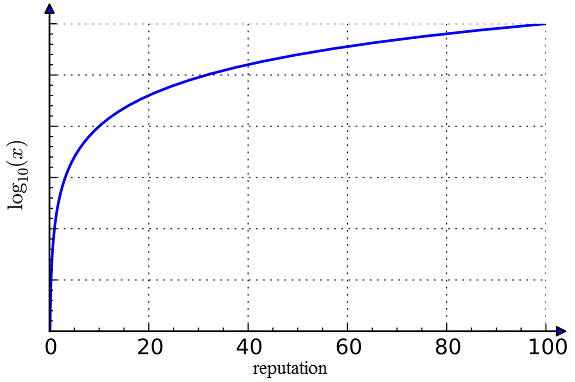
As you can see, is it very easy to raise your reputation at the beginning, but the higher your reputation, the harder it is to increase it. In fact, each time you want to increase your reputation of 1 point, it is ten times harder!
The main effect is that a reputation of 60 is 10 times stronger than the reputation of 59.
It is the same for a negative reputation. A reputation of -8 is 10 times weaker than the reputation of -7.
People with a low reputation cannot harm the reputation of someone with a strong reputation.
This explains why creating a bot that systematically flags other posts is useless, unless the bot has a high reputation, something that will be hard to achieve for a “flag bot”. In no time, the bot’s reputation will be ruined and it will become harmless.
The lower limit of reputation is -8
There is no upper limit to reputation.
About Reward Shares
Before continuing to see how reputation points are “computed”, you need to understand the concept of “Reward Shares“
When you vote for a post or comment, you tell the system to take some money (the reward) from the Global Reward Pool and give 75% of this reward to the author (the author reward) and to share the remaining 25% of the reward between the people that voted for the post (the curation reward).
The more votes for the post from people with high voting power, the higher both rewards will be.
But the curation reward is not distributed equally among all voters.
Depending on the timing of your vote, the voting power you have and how much you allocated to your vote (percentage gauge), you will get a tiny part of the pie or a bigger one.
The size of your part is called the reward share
Here is an example of the distribution of the reward share on a comment:
You see that, despite all users voted with full power (100%), they have different Reward Shares.
OK, now, let's go back to the reputation. All you have to do is to keep in mind this Reward Share value exists.
How reputation is “computed”
Each time some vote for a post or a comment, the vote can have an impact on the author reputation, depending on:
- the reputation of the voter
- the Reward Share of the voter
Let's have a look at the code that is executed each time you vote for something.
You can find it on github here
const auto& cv_idx = db.get_index< comment_vote_index >().indices().get< by_comment_voter >();
auto cv = cv_idx.find( boost::make_tuple( comment.id, db.get_account( op.voter ).id ) );
const auto& rep_idx = db.get_index< reputation_index >().indices().get< by_account >();
auto voter_rep = rep_idx.find( op.voter );
auto author_rep = rep_idx.find( op.author );
// Rules are a plugin, do not effect consensus, and are subject to change.
// Rule #1: Must have non-negative reputation to effect another user's reputation
if( voter_rep != rep_idx.end() && voter_rep->reputation < 0 ) return;
if( author_rep == rep_idx.end() )
{
// Rule #2: If you are down voting another user, you must have more reputation than them to impact their reputation
// User rep is 0, so requires voter having positive rep
if( cv->rshares < 0 && !( voter_rep != rep_idx.end() && voter_rep->reputation > 0 )) return;
db.create< reputation_object >( [&]( reputation_object& r )
{
r.account = op.author;
r.reputation = ( cv->rshares >> 6 ); // Shift away precision from vests. It is noise
});
}
else
{
// Rule #2: If you are down voting another user, you must have more reputation than them to impact their reputation
if( cv->rshares < 0 && !( voter_rep != rep_idx.end() && voter_rep->reputation > author_rep->reputation ) ) return;
db.modify( *author_rep, [&]( reputation_object& r )
{
r.reputation += ( cv->rshares >> 6 ); // Shift away precision from vests. It is noise
});
}
Here you are. Everything you ever wanted to know is defined is those 33 lines of code.
Now that you have read them, isn’t it clear to you?

I you feel like this, don’t worry. I will help you to translate it to a human understandable language.
auto cv = cv_idx.find( boost::make_tuple( comment.id, db.get_account( op.voter ).id ) );
Out of all votes, get the vote info of the voter (you)
auto voter_rep = rep_idx.find( op.voter );
auto author_rep = rep_idx.find( op.author );
Get the reputation of the voter (you)
Get the reputation of the author of the post or comment
// Rule #1: Must have non-negative reputation to effect another user's reputation
if( voter_rep != rep_idx.end() && voter_rep->reputation < 0 ) return;
Self documented, if you have the a negative reputation, the process stops.
You have no influence on other’s reputation.
if( author_rep == rep_idx.end() )
The process check the existing reputation of the author
- Case 1: the author has no reputation yet
// Rule #2: If you are down voting another user, you must have more reputation than them to impact their reputation
// User rep is 0, so requires voter having positive rep
if( cv->rshares < 0 && !( voter_rep != rep_idx.end() && voter_rep->reputation > 0 )) return;
Self-documented, if your vote is negative and your reputation is not positive, the process stop.
db.create< reputation_object >( [&]( reputation_object& r )
The reputation of the author is initialized, then ...
r.reputation = ( cv->rshares >> 6 ); // Shift away precision from vests. It is noise
Your Reward Share becomes the new author reputation.
- Case 2: the author has some reputation, the process is pretty similar ...
// Rule #2: If you are down voting another user, you must have more reputation than them to impact their reputation
if( cv->rshares < 0 && !( voter_rep != rep_idx.end() && voter_rep->reputation > author_rep->reputation ) ) return;
Self-documented, if your vote is negative and your reputation is not greater than the author’s reputation, the process stop.
db.modify( *author_rep, [&]( reputation_object& r )
The process will modify the existing author reputation
r.reputation += ( cv->rshares >> 6 ); // Shift away precision from vests. It is noise
Your Reward Share is added to the author’s reputation.
That’s it. Easy and simple.
Finally, the reputation is simply a VERY BIG number that contains the sum of all the Reward Shares of every votes associated to your posts and comments.
If someone unvote your post, his Reward Shares get deduced and your reputation is lowered.
If your post or comment gets flagged, the Reward Share is deduced and your reputation is diminished.
To display it as a human readable number , you can use the following formula:
max(log10(abs(reputation))-9,0)*((reputation>= 0)?1:-1)*9+25
How to raise your reputation
The best way to increase your reputation to get votes from people with a positive reputation and, even better, with a lot of voting power.
To achieve this goal:
- publish quality posts. Forget quantity, it's about quality!
- take part in the discussions (you can get extra rewards and reputation points for your comments)
- vote carefully (do not vote for crap posts, vote for appropriate content and authors)
- increase the number of followers and build your following list
Conclusion
I hope you now better understand how reputation works and how to build it.
Remember, reputation is a key factor which reflect how you behave and how the members of the community evaluate your work.
As in real life, having a high level of reputation is a hard work and a long run.
And as in real life, ruining it can be very fast. Then it will be even harder to rebuild.
If you aim at top reputation, focus on quality and on a constructive attitude.
Thanks for reading!
Support me and my work as a witness by voting for me here!

You like this post, do not forget to upvote or follow me or resteem
"Everything you ever wanted to know is defined is those 33 lines of code." Free masons have 33 degrees , therefore Illuminati confirmed ! :)
doesn't this formula intents that when you have negative reputation and it gets more and more negative your displayed reputation will only grow due to 'abs' operator?
You're right. I posted with an old formula.
Here the correct one:
[display reputation] = max( log10(abs(reputation)) - 9, 0) * ((reputation >= 0) ? 1 : -1) * 9 + 25
I updated the post
The abs() is because a logarithm from a negative number is not possible, so they have to make it positive before the logarithm. No need to worry :)
This post has been ranked within the top 80 most undervalued posts in the second half of Jan 07. We estimate that this post is undervalued by $6.58 as compared to a scenario in which every voter had an equal say.
See the full rankings and details in The Daily Tribune: Jan 07 - Part II. You can also read about some of our methodology, data analysis and technical details in our initial post.
If you are the author and would prefer not to receive these comments, simply reply "Stop" to this comment.
I am new here and really confused. There have too many things things to learn 😢
Same
This has been incredibly helpful for a new user!
Thank you very much for sharing this information.
Glad you found it interesting
That's a great post. I think it is great content and definitely undervalued.
Well done.
Thanks! Your comment is really appreciated.
My full Movie review- just out if you are looking for something good to watch/read etc BankingOnBitcoin -- Movie just released Friday!
https://steemit.com/bitcoin/@barrydutton/movie-review-banking-on-bitcoin-brand-new-movie-out-showcasing-all-the-names-and-issues-we-are-familiar-with-in-the-liberty
Please, avoid promoting unrelated posts!
I was going thru comments and replying to people and in cases where someone provided me value, I try and do that back - in this case with a brand new movie out, tied to the crypto space I thought you might like.
Followed.
Thanks =)
Thanks so much. I just signed up and I'm trying to figure it all out still. This really helped.
Follow you.
I 'm sorry,, I decreased my reputation ,, and I don't know how it was decreased :(
i joined here just a few days and I've shocked now!!
I think It because of my votes!! I just know that we can vote 10 times in a day... and I didn't know that before!!
Is it can be a reason?? and now.. could you do me a favor and help me to increase it :(
I'm very sad
That was an awesome post.... truely thank you for sharing , i have been wondering about it for long.
It took me two weeks of searching to find this post to answer my questions about how reputation is calculated. Thanks so much. I feel a lot better now.
It is too late for rewards on this post, but I did give you my witness vote.
Glad you finally found it =)
Many thanks for your witness support!
Looks very helpful. I was kind of like that toddler staring at that computer screen. Ha! This would be good for resteeming. It looks looks like it's still valid for general understanding purposes.
Wow, I've learned from this post. Thanks for your time.
Thank you, well explained.
Great post, didn't expected for somebody to actually analyze the code from the repository:)) Now I understend why it's getting so hard to increase my reputation.
Thank you @arcange ! What do you think about this idea?
https://steemit.com/blockchain/@aigents/proof-of-reputation-as-liquid-democracy-for-blockchain
This is a very interesting post. I missed it when it was published.
Such a pity it didn't generated more comments and discussion.
I know my comment is really old here, but I want to say thanks. This is the only post I have ever seen that explained how the raw reputation score was computed. (And I have read a lot of them, looking for this information.) It makes sense that it would be the sum of all the reward shared ever voted on. That explains it all!
Thanks..for this information....
thank you very much for the great post and helpfull information
Thank you for the post. Greatly help newbie like me.
awesome post,thanks a lot
I can't believe this post only got $2 at time of payout. By far the best explanation of rep out there. I upvoted your most recent one since this one's time has passed.
I recently wrote a follow on post about rep with a few more bullets laid out. It also includes some findings that I found interesting. I hope you don't mind me posting the link here. I think it will be a good follow on resource for folks looking into rep in the future. It will be passed payout in three days as well, so I'm not really looking for upvotes. Let me know if you would rather I take it down. https://steemit.com/reputation/@moeknows/some-interesting-facts-about-reputation-on-steemit
Very informative. Thank you very much for this.
Thanks a lot. Now i understand what it is?
This is very curious for me to see this post having 2$ only.
great post
Great post about reputation! The resteem button is no longer available, but i did @follow you. Maybe you should make an update.
Will reputation go down with inactivity?
Thank you for your comment @buckfumbler
I will think of an update of the post.
No, activity or inactivity has no effecf on reputation by itself, except that when you are active, you create interaction with other users, wich might influence your reputation.
I read your post all long but still have some confusions. Can you please let me know?
what is the impact on my reputation upon commenting to any post or upvote any post.
Is there any increase in reputation if any positive reputation person comment me back or upvote my comment?
what a valuable post to us new steemians. Really...thank you. I had no idea how to protect let along grow my reputation number. Thanks for this post.
Now I will focus on increasing my reputation
View or trade
BEER
.Hey @arcange, here is a little bit of
BEER
from @pixresteemer for you. Enjoy it!Learn how to earn FREE BEER each day by staking your
BEER
.Better start with a new account!