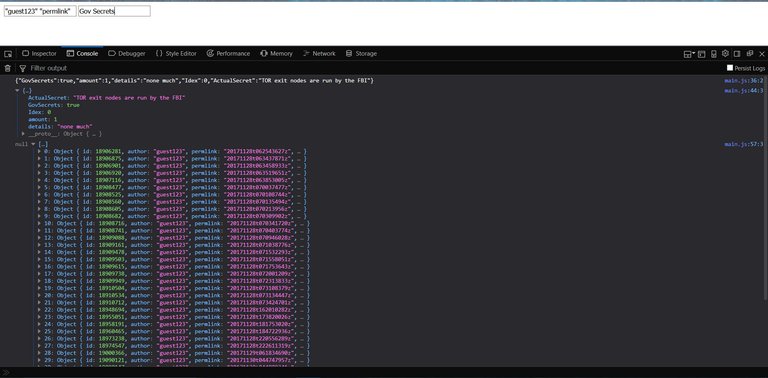
Have you ever wanted to make your own API? Well now’s the time to do it, make any info available in a web developer or programmer friendly way. What I’m talking about here is the ability to give someone a couple of numbers and letters that they can plug into some code or a website and get a feed of info, the info you’re putting out there. Setting up a way to host information can get real tricky real quick, especially considering the facts that you don’t want to mess with no personal server, don’t trust no central authority, and want it as easy as humanly possible. What I’m about to elaborate on is just a little thing I’ve been messing around with right here on Steemit and the Steem blockchain. Now don’t get me wrong, I am not trying to bloat the network or anything, this idea works just fine with the backing principles of Steemit. I mean, what is a social media site truly about here, the sharing of information, socially I guess but whatever, you could make this social too, anything really.
What if I wanted to stream out some government secrets I just got, but I’m a nice guy, I’ll use Steemit to post them both as an article and as a JSON string. Now those friendly folks at wikileaks can just scan my link, parse the JSON, and boom, easy access to my socially shared, totally transparent, and hosted on a decentralized semi-virtual server where it’ll be difficult to take down, tasty secrets. It’s a win for everybody, except whoever’s the bad guy. Want to throw some passwords on here cause screw that server company that hosts your little shops website, no problem, just SHA256 that shite and a cookie to anyone who can crack em. Oh and of course, the social aspect can get pretty interesting too; make a post that contains what you eat every day, then if you become famous enough one day, various websites will literally stream your API and make analyses on what you eat, at least that’s the idea. I’m not even joking, as funny as it might seem I can see it happening, I can see myself doing it. Anyway, enough of this looong intro, lets see how to actually do it.
Super Simple Way.
Step 1: Make a post that will be designated as your database post. It could simply be an article detailing those juicy government secrets you have.
Step 2: Make sure any information you want to pass through the API request is in the comment section, not the actual post. This is to make it easy to scan through info and avoid the 7 day stop on post editing if you want to keep adding info.
Step 3 -very important syntax here: Write all your API comments in the JSON format which is:
- {"a":"b"}
- "a" is the identifier and must always have double quotes around it and preferably no spaces. For example, "a" could equal "AmountOfSecrets"
- "b" is the actual information and does not need double quotes if it's a number or true/false.
- Examples: {"AmountOfSecrets":7} or {"AreThereSecrets":true} or {"TheActualSecret":"Tor exit nodes are all run by the FBI"}
Step 4: Provide the following javascript code to whoever wants to access your info and tell them to get the steemjs library at https://www.npmjs.com/package/@steemit/steem-js
function getInfoFromSteem() {
steem.api.getContentReplies('account name', 'permlink', function(err, result) {
console.log(err, result);
});
}
They ought to know what to do with that but you'll have to fill in the space for your account name in the quotes, and the permlink is found after your name in the browser search box.
An example for this post is:
function getInfoFromSteem() {
steem.api.getContentReplies('loshcat', 'api-s-for-everyone-making-your-own-code-friendly-api-for-info-right-here-on-steemit', function(err, result) {
console.log(err, result);
});
}
This returns all the comments under the database post they can then scan through, find JSON bodies, and use the information inside easily.
Getting Slightly Fancy.
If you want to automate the addition of information based on when it's gained, you could set up something in node.js such as running this every time you get some new secrets:
function updateGovSecrets() {
let Pbody = JsonParser();
steem.broadcast.comment(
postingWif, // Your Steemit Posting Key.
AuthPerm, // Parent Author ie. Author of content you are commenting to.
Mtag, // Parent Permlink or Main Tag; if a comment to content, Permlink of said content.
username, // Author ie. Your account name.
permLink, // Permlink ie. dynamically created permlink for your comment or content.
Ptitle, // Title; if comment to content - leave empty.
Pbody, // Body ie. actual stuff that is posted.
{
tags: ['tag2', 'tag3'],
app: `mine`,
anyIdentifier: 'anything'
}, // Json Metadata, tags start at 2 because of main tag.
function (err, result) {
if (err) {
alert(err, result);
} else {
console.log('Broadcast Successful');
}
}
);
}
and
function toJson(jsonKey, keyContent) {
let JSoned;
if (!isNaN(keyContent) || typeof keyContent === 'boolean') {
JSoned = String('"' + jsonKey + '"' + ':' + keyContent);
return JSoned;
} else {
JSoned = String('"' + jsonKey + '"' + ':' + '"' + keyContent + '"');
return JSoned;
}
}
function constructJson() {
let comma = ',';
let Jstringed = String('{'
+ toJson('GovSecrets', true) + comma
+ toJson('amount', 1) + comma
+ toJson('details', 'none much') + comma
+ toJson('Idex', addIndex) + comma
+ toJson('ActualSecret', newInfo)
+ '}');
console.log(Jstringed);
return Jstringed;
}
function JsonParser() {
try {
let parseFin = JSON.parse(constructJson());
console.log(parseFin);
return constructJson();
} catch(er) {
console.log(er);
}
}
newInfo could be a global variable reset to new gov secrets or newInfo(), a function to evaluate it somehow.
Not sure if 100% accurate since I whipped it up kinda quick right now but it should show the general idea of creating JSON, formatting it into a string so you can post it as a comment, parsing it to check JSON validity, and making the post using steemjs. I think you could also add whatever info you want to the metadata so that's an option as well if you wanna seem sneaky.
This is just an interesting look into decentralized server space and how to kinda use it as a makeshift store for info. Is this necessary? I don't know. Is this maybe better suited for other things like IPFS? No clue. Is this fun and transparent? Definitely.
Byeeeeeee
U2FsdGVkX1/xUAr/N0YynGEwTIPuwN0CxlnicSlwjlXV6ZvfH4V4LGUSuDK5Vxb1EY8tq1Fz5La5UeKAQsDptzuxIciCUD3SvPDdVWHkI0++HBmefnvWp2FazNPT0u1Y
very funny ;)