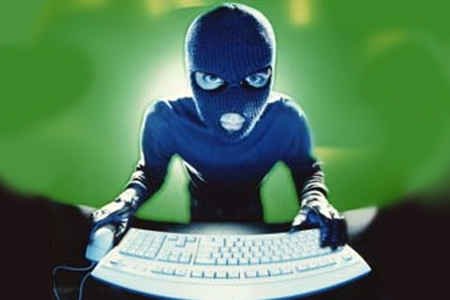
For my 100th post I felt like I had to share something special.
So here's a nifty node package that makes writing your own IRC bot surprisingly easy. Although I'm still in the process of writing my own, I thought that I could share what I've learned and pass on the basics to help other people get started too!
What is IRC?
As IRC is not a mainstream technology anymore, some of you might be wondering what it is. Instant Relay Chat (IRC) is one of the first chat protocols to ever become popular on the internet. It's very light because it emerged at a time where bandwidth and processing power were scarce. But as a result it's very efficient. To chat with other users, first connect to your network of choice and then to any of the available channels. There are dozens of well-populated networks with millions of channels.
I love to use IRC because it's one of the last places on the internet where you can engage in a constructive instant messaging chat with strangers about almost any topic. Just like steemit :)
What's the point of an IRC bot?
IRC bots can be used for all sorts of tasks. You could simulate another user (by writing a simple AI for example). You could make the bot send certain messages into the channel. You could write a bot that administrates your channel and kicks people who are being naughty. You could make your bot scrape a website for you and relay useful information. It's up to your imagination. Really!
What you will need
Minor familiarity with:
- Javascript (or some OOP)
- Node.js
- IRC commands
I wouldn't get too hung up on these requirements though. Many people like to learn as they go and as you'll mostly be copying commands out of the docs anyway.
Note that the rest of this guide will be written in the perspective of a Linux user. The steps should be almost identical if you're using Unix. Windows users will be mostly working from the GUI, so that shouldn't be a problem either.
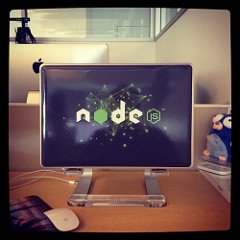
Install node and npm
If you're on Ubuntu, this is as simple as:
sudo apt-get update
sudo apt-get install nodejs
sudo apt-get install npm
If you're on Windows, fetch the Windows installer from the official node website.
Prepare your project folder
Make a new folder for your project:
mkdir project-name
Install npm's irc package
npm install irc
Skeleton
As with most programming projects, it helps to start with a skeleton.
Here's everything you need to make a bot that connects to the #botwar channel on the freenode IRC network. The reason I chose this channel is because they allow you to experiment with your bots (up to an extent). If you want to change the channel the bot sits in and listens, change #botwar to something else. Make sure not to bother anyone else while you're tinkering away!
The bot has one listener that says "¿Que pasa?" into the #botwar channel whenever anyone sends a message to the channel. So basically this bot just annoyingly answers what everyone says with the same message.
// Create the configuration
var config = {
channels: ["#botwar"],
server: "irc.freenode.net",
botName: "your-bot-name"
};
// Get the lib
var irc = require("irc");
// Create the bot name
var bot = new irc.Client(config.server, config.botName, {
channels: config.channels
});
// Listen for any message, say to him/her in the room
bot.addListener("message", function(from, to, text, message) {
bot.say(config.channels[0], "¿Que pasa?");
});
Check out David Walsh's blog for a more detailed description behind the functions in this program.
Run
So to run this thing, save the script above and execute it with
node your-script.js
or
nodejs your-script.js
depending on your distro and whether you have nodejs-legacy installed.

Customize
So at this point the rest is up to your imagination. You can add more listeners, with different event parameters, and different responses.
You should make sure to add an error listener though so you know if the sever is having trouble communicating with your bot.
bot.addListener('error', function(message) {
console.log('error: ', message);
});
To get a better idea of the types of tools that are at your disposal, check out the documentation.
Another thing to note, is that you can add several channels to the config.channels array. I like to just include it in the config variable at the top like so:
var config = {
channels: ["#botwar", "#add", "#more", "#channels", "#here"],
server: "irc.freenode.net",
botName: "your-bot-name"
};
Closing Thoughts
And that's that! Don't hesitate to ask some questions but keep in mind that I'm still learning this too!
Check out my Steemit profile for more tutorials, philosophical essays and prose!
If you want to know what I'm up to, or get in contact with me, check out my portfolio website.