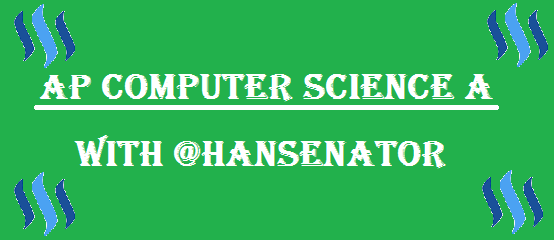
An Armstrong number is defined to be an integer where the sum each digit raised to the power of the number of digits in the number equals the number itself. Haha. Sound confusing? Well here is are two examples:
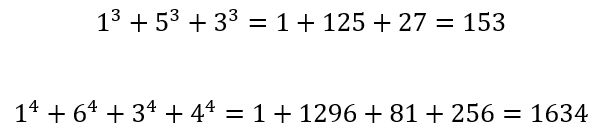
My program to find these numbers:
Surprisingly this only took 53 lines of code... It can be done in less.
The output:
Here are all Armstrong numbers from 1 to 100,000
1, 2, 3, 4, 5, 6, 7, 8, 9, 153, 370, 371, 407, 1634, 8208, 9474, 54748, 92727, 93084
If you want to try out the raw code yourself you can go to browxy.com or codiva.io and copy/paste this:
class Armstrong {
// This is the main class. It runs the program.
public static void main(String[] args) {
AllArmstrongNumbers(1,100000);
}
// This prints out all Armstrong numbers from 1st to 2nd argument.
public static void AllArmstrongNumbers(int min, int max) {
boolean ANum;
for (int i = min; i <= max; i++) {
ANum = isArmstrong(i);
if (ANum == true) {System.out.print(i + " ");}
}
}
// This counts the number of digits in a number.
public static int digitCount(int n) {
int count = 0;
while(n != 0) {
n = n / 10;
count++;
}
return count;
}
// This is an exponent calculator.
public static int power(int base, int exponent) {
int summ = base;
for(int i = 1; i < exponent; i++){
summ = summ*base;
}
return summ;
}
// This finds out if a number is Armstrong.
public static boolean isArmstrong(int num) {
int remainder;
int exp = digitCount(num);
int originalNumber = num;
boolean ArmstrongNumber;
int summ = 0;
for (int i = 1; i <= exp; i++) {
remainder = num % 10;
summ = summ + power(remainder,exp);
num = num / 10;
}
if (summ == originalNumber) {ArmstrongNumber = true;}
else {ArmstrongNumber = false;}
return ArmstrongNumber;
}
}

All SteemitEducation Posts
Physics
Kinematics Video, Circular Motion Video, Forces in 1 & 2 Dimensions Videos, Kinematics Full Lesson, Circular Motion and Gravitation Full Lesson, Kinematics and Forces Quiz, Forces in 1-Dimension, Forces in 2-Dimensions, Basic Physics, Kinematics, and Forces Review, AP Physics Midterm Exam, AP Physics Midterm Exam Solutions , Kinematics and Forces Quiz
Computer Science
1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17
SAT
Asymptotes, Composite Functions, Contest 1 - Area, Contest 1 – Winners and Solution, PEMDAS, Systems of Equations, Functions, Exponents, Contest 2 - More Functions, Contest 2 – Winners and Solution, Percents, The Interest Equation, All About Division, Contest 3 - Factoring, Contest 3 – Winners and Solution, Fractional and Negative Exponents, Basic Trig, Contest 4 - Math Vocab, Contest - 5, Contest 5 – Winners and Solution, Contest 6, Contest 6 – Winners and Solution
General Math
Basics of Algebra, Trigonometry Video, Proof of Quadratic Formula, Factoring Video, Unions and Intersections, Surface Area of a Cylinder, Substitution Video, Combinatorics Basics
Graphing from Algebra to Calculus
Algebra 1, Algebra 2, Pre-Calculus, Calculus
Piloting Airplanes
Introduction, Preflight, Requirements, Instruments, Take-Offs and Landings, Why a Plane Can Fly, Reading the Runway, Maneuvers
Other
Engineering (Isometric) Drawing, Pressure & SCUBA Diving

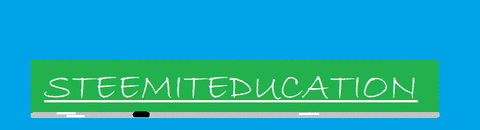
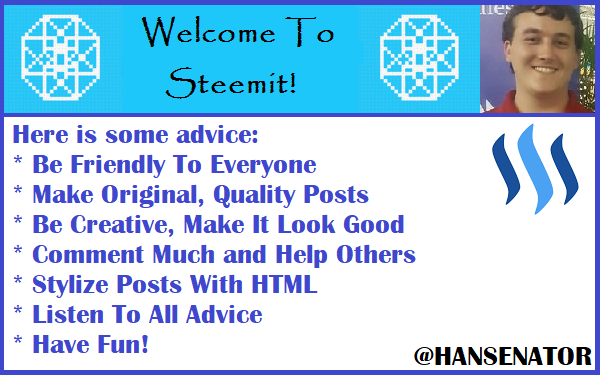
@hansenator,
Excellent java lesson. Bro add utopian-io tag. I think it might work well. Shall we built Steem app base on java?
Cheers~
I am currently working on a Steem Blockchain downloader in Java. My goal is to learn more about blockcahin and to have the data easily accessible for analysis. This already exists in Python but I'm more concerned with the learning effect.
Thanks Guru! I will definitely change the tag for the next java post! I would love to see a better Steem app! Haha
Hi, I took your great post as reason to implement this in python myself.
def getArmstongNumbers(start, end): armstongNumbers = [] for nr in range(start, end): sum = 0 for digit in str(nr): sum += pow(int(digit), len(str(nr))) if sum == nr: armstongNumbers += [nr] return armstongNumbers print(getArmstongNumbers(1, 100000))
Maybe we will find more users who post their own implementation here.
[v for p,v in enumerate([sum([int(d)**len(str(i))for d in str(i)])for i in range(7**6)])if p==v]
The readability is lost, but it is even shorter.
Awesome work guys! Glad I could inspire you to try it out!
This post has received 50% upvote from @minnowspower thanks to: @theguruasia
MinnowsPower is not a bot, I am a Crowdfunding Hybrid
no entendi muy bien me podrias exolicar. gracias.
Wow excellent tutorial & very helpful post.
I am new so i follow u
Thank you Raja! More to come soon!
Ok ... i really waiting for your post..
I'm not a programmer. But I like programmer.
By the way, thank you for your excellent advice...... :)
Thanks success! Hope to see you again!
@hanasenator...nice programming skils you have ..i am also a developer but i am working on a html flat form..i think their is no errors in this programme according to my knowledge..good work..thank you for sharing with us...
Thank you! I worked on it with my class the day before, so I hope it works! Hahaha. Best wishes to you!
@hansenator...very nice java programme ..you are useing constructors to execute the problem..you have a good knowledge in java programing bro. Thank you for sharing with us..
Thank you. The knowledge is only growing! Glad to hear from you durgaani!!!
Thank you! American Idol is a God awful show though. Haha.
i like your tutorial all time . your tutorial is so talented my dear friend @
@hansenator
Thank you khurshid! You are appreciated as well!
very helpful post i like it friend ...
& great work