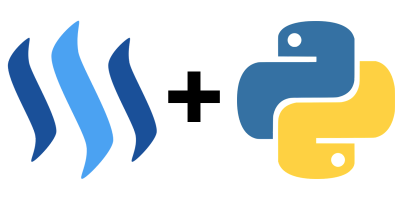
This tutorial is a continuation of Part 1: How to manage your wallet using steem-python - Automatically claim rewards, so make sure to read this before starting this tutorial. Today we will learn how to calculate how much STEEM we will get for selling our SBD and how to create an order on the market!
What will I learn?
- How to calculate how much STEEM our SBD is worth (at the lowest ask)
- How to create an order on the market
Requirements
- Python3.6
- steem-python
Difficulty
- Intermediate
Tutorial
Calculating our SBD's value in STEEM
When selling my SBD I usually go to the market via my browser and simply create an order on the market at the lowest ask. This is pretty tedious so let's see how we can do this automatically using steem-python
!
Our first step is finding out how to get our account's balance and simply show how much STEEM we would get if we sell all our SBD at the current lowest ask. To get the market ticker for the internal SBD:STEEM market we can simply use get_ticker()
function, which gives us the following information
{
"latest": "0.89493466976910685",
"lowest_ask": "0.89734386216798279",
"highest_bid": "0.89261804873694550",
"percent_change": "3.00698049042419946",
"steem_volume": "7890.950 STEEM",
"sbd_volume": "7124.069 SBD"
}
we can use this to get the lowest ask and calculate how much STEEM we can sell our SBD for as follows
sbd_balance = Amount(steem.get_account(USERNAME)["sbd_balance"]).amount
lowest_ask = float(steem.get_ticker()["lowest_ask"])
print("PRICE\t{:0.6f}\nAMOUNT\t{:0.3f}\nTOTAL\t{:0.3f}".format(
lowest_ask, sbd_balance / lowest_ask, sbd_balance))
which outputs the following
PRICE 0.897344
AMOUNT 9.750
TOTAL 8.749
This is indeed the same as the market shows (except for the fact we round the values in our program), as seen in the image below
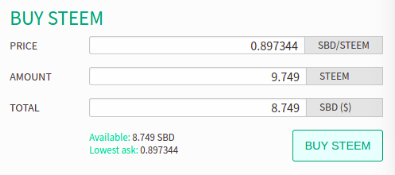
Creating an order on the market
Now we know what the lowest ask is, we can create an order on the market for that price. If you do this every hour and have a low amount relatively low amount of SBD like me, then you can probably simply create an order at the lowest ask and expect it to go through.
To do this we should've been able to use the sell()
function, but trying to do this gives the following error
AttributeError: 'Steemd' object has no attribute 'commit'
This can be fixed! You can either edit your python3.6/site-packages/steem/dex.py
file manually and replace it with this file where I have fixed the problem, or you can clone the repository where I have fixed this and install steem-python
like so
$ git clone https://github.com/amosbastian/steem-python/tree/dex-commit
$ cd steem-python
$ pip install -e .
Note: I recommend making a virtual environment for every project!
Once this has been fixed we can simply use the following code to sell our 0.1 SBD for STEEM! We set the order to expire an hour after making it in case it doesn't go through
from steem.dex import Dex
dex = Dex()
dex.sell(
amount=0.1,
quote_symbol="SBD",
rate=(1.0 / lowest_ask),
expiration=3600,
killfill=False,
account=USERNAME
)
and indeed, going to my account on steemd.com shows that the order went through.
If you want to sell all your SBD simply replace amount=0.1
with amount=sbd_balance
.
Congratulations! Your program can now claim your rewards automatically and then sell your SBD on the market for STEEM!
Note: if you have more SBD to sell than me it could happen that your orders don't go through. To prevent this you could add a small buffer (that you are comfortable with) to the lowest_ask
variable so that you sell your SBD for slightly less, but at least it does sell. Orders expire anyway, so even if it doesn't go through there's nothing to worry about!
Curriculum
The code for this tutorial can be found on my GitHub!
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks!
Thanks for sharing, very useful
Hey @amosbastian I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x