Crypto Core has the ambitious target to provide a Swiss Army knife for Blockchain related projects.
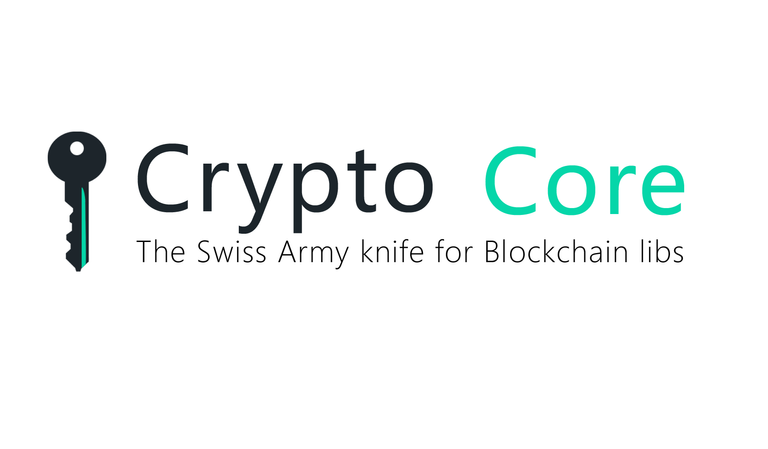
This project has the ambitious target to provide a Swiss Army knife for Blockchain related projects.
Crypto Core - A Swiss Army knife for Blockchain related Java projects
Hello Steemians!
I am really proud to share my latest project with you: It is called Crypto Core and I already had it in mind for a long long time, but never managed to push it until now.
Everything began with the development of version 0.2.0 of SteemJ. This version added 'Transaction Signing' to the project and the main part of this feature is based on some bitcoinj methods. At this point I was already disappointed of using the whole bitcoinj project with all its dependencies, while only being able to use 1% of their code.
This disappointed me even more when I've started to develop SteemJ-Image-Upload. This project now has a huge amount of dependencies due to bitcoinj and is not the small, additional lib it was supposed to be.
So because of that, I've asked the bitcoinj developer to separate the non-bitcoin related code to a separate package in Issue #1486. Their feedback was quite positive, but so far, I received no feedback in their forum and decided to start something on my own.
The upcoming chapters will explain the background behind the project and the problem it tries to solve in detail. The content below is based on the README of the project which has been updated in the following commit:
https://github.com/marvin-we/crypto-core/commit/8362b88fe2d71cfbaf9a9722e574e65f0830a44c
Background
It is well known that every Blockchain technology should have its own special features which makes it more or less unique. Because of this, every specific Blockchain requires its own specific library which implements and supports those features. When having a look at Java Api implementations, bitcoinj, etheriumj or steemj are good examples of those unique libraries.
However, a lot of Blockchain technologies use the same core mechanisms which every Blockchain related project has to solve. To make this more clear, here are some examples:
- SHA256 hashing
- Base58 encoding/decoding
- HEX encoding/decoding
- Handling of private and public keys
- Signing of messages
- Handling of common C types like
VarInt
that do not exist in Java - ...
The team around bitcoinj, which is the oldest and most proven Java Api implementation for a Blockchain, already solved all those issue. Sadly, the implementations are specific for Bitcoin and a big part can't be used out of the box for other Blockchain technologies. This fact forced other projects to:
- Implement a custom solution (e.g. the IOTA Java Api).
- Copy the source code of bitcoinj into their own project and adjust it (e.g. etheriumj).
- Use the full bitcoinj project and build the required changes around it (e.g. steemj).
The first approach is something you should only take into concideration if you have a really strong crypto and blockchain background as the those topics require a lot of knowledge.
The second approach is a better idea, as the well tested, documented and proven classes of the bitcoinj project are used. The disadvantage of this approach is that updates and fixes require a manual merge.
The third approach solves also the problem of manual merges, but requires to have add the whole bitcoinj project with all its dependencies and classes while only using ~2% of their code.
How crypto core solves this problem?
The idea behind crypto core is to provide a flexible and well documented Swiss Army knife for as much Blockchain technologies as possible. This is archieved by extracting non Bitcoin related classes from the great bitcoinj library and making those methods more flexible.
Example
The following snippets show the difference between bitcoinj and crypto core using the signMessage
as an example.
Using BitcoinJ
The ECKey
object of the bitcoinj project is used in a lot of other Blockchain projects like steemj, etheriumj or graphenej. It provides a signMessage
method which can be used to sign a message and, for sure, this is something every Blockchain related project has to do.
Sadly, the bitcoinj implementation is doing this by creating an SHA256 Hash of the message as you can see in line 2:
public String signMessage(String message, @Nullable KeyParameter aesKey) throws KeyCrypterException {
byte[] data = Utils.formatMessageForSigning(message);
Sha256Hash hash = Sha256Hash.twiceOf(data);
ECDSASignature sig = sign(hash, aesKey);
// Now we have to work backwards to figure out the recId needed to recover the signature.
int recId = -1;
for (int i = 0; i < 4; i++) {
ECKey k = ECKey.recoverFromSignature(i, sig, hash, isCompressed());
if (k != null && k.pub.equals(pub)) {
recId = i;
break;
}
}
if (recId == -1)
throw new RuntimeException("Could not construct a recoverable key. This should never happen.");
int headerByte = recId + 27 + (isCompressed() ? 4 : 0);
byte[] sigData = new byte[65]; // 1 header + 32 bytes for R + 32 bytes for S
sigData[0] = (byte)headerByte;
System.arraycopy(Utils.bigIntegerToBytes(sig.r, 32), 0, sigData, 1, 32);
System.arraycopy(Utils.bigIntegerToBytes(sig.s, 32), 0, sigData, 33, 32);
return new String(Base64.encode(sigData), Charset.forName("UTF-8"));
}
So only because of the first two lines the whole rest of the method can't be used for other blockchain projects that do not generate the hash of a message in the exact same way. This forces developers to search for other solutions instead of being able to reuse the well tested bitcoinj code.
Using Crypto Core
Crypto Core solves this issue by removing Bitcoin related functionallities from those methods. In this concret example it allows to provide the hash itself as a parameter so that the remaining 90% of the method can be reused.
public String signMessage(Sha256Hash messageHash, @Nullable KeyParameter aesKey) {
ECDSASignature sig = sign(messageHash, aesKey);
// Now we have to work backwards to figure out the recId needed to
// recover the signature.
int recId = -1;
for (int i = 0; i < 4; i++) {
ECKey k = ECKey.recoverFromSignature(i, sig, messageHash, isCompressed());
if (k != null && k.pub.equals(pub)) {
recId = i;
break;
}
}
if (recId == -1)
throw new RuntimeException("Could not construct a recoverable key. This should never happen.");
int headerByte = recId + 27 + (isCompressed() ? 4 : 0);
byte[] sigData = new byte[65]; // 1 header + 32 bytes for R + 32 bytes
// for S
sigData[0] = (byte) headerByte;
System.arraycopy(CryptoUtils.bigIntegerToBytes(sig.r, 32), 0, sigData, 1, 32);
System.arraycopy(CryptoUtils.bigIntegerToBytes(sig.s, 32), 0, sigData, 33, 32);
return new String(Base64.encode(sigData), Charset.forName("UTF-8"));
}
This would, e.g., allow etheriumj to use this method with a keccak hash.
Technologies
- Java 7
- Maven 3+ - for building the project
- Google Protocol Buffers - for use with serialization and hardware communications
Full Documentation
As Crypto Core only adopts the method signatures in most cases, the bitcoinj documentation should still be the best way of searching for something. Beside that Crypto Core profits and extends the original JavaDoc developed by the bitcoinj team.
Communication
If you want to contact me you can:
- Use this thread in the bitcoinj google group where I've announced this idea.
- contact me directly at the Discord Java Channel.
- create an Issue here at GitHub.
Contribution
This project is really young and requires the help from every user to improve and become more usable. So every kind of feedback is more than welcome.
If you want to contribute simply clone the repository:
And submit a pull request :)
Binaries
Crypto Core binaries are pushed into the maven central repository and can be integrated with a bunch of build management tools like Maven.
Maven
File: pom.xml
<dependency>
<groupId>eu.bittrade.libs</groupId>
<artifactId>crypto-core</artifactId>
<version>0.1.0pre1</version>
</dependency>
How to build the project
The project requires Maven and Java to be installed on your machine. It can be build with the default maven command:
mvn clean package
The resulting JAR can be found in the target directory as usual.
Bugs and Feedback
For bugs or feature requests please create a GitHub Issue.
For general discussions or questions you can also:
- Post your questions in the Discord Java Channel
- Reply to one of the Crypto Core update posts on Steemit.com
- Contact me on steemit.chat
Get in touch!
Most of my projects are pretty time consuming and I always try to provide some useful stuff to the community. What keeps me going for that is your feedback and your support. For that reason I would love to get some Feedback from you <3. Just contact me here on Steemit or ping me on GitHub.
Thanks for reading and best regards,
@dez1337
Posted on Utopian.io - Rewarding Open Source Contributors
What are the use cases of this project ?
It allows people to start blockchain related project like api wrappers based on a lightweighted dependency instead of using the full bitcoinj project :)
Thank you for your contribution.
Your contribution cannot be approved yet, because it is in the wrong category.
Going through it, we noticed this more represents a documentation about the project, and is essentially a copy of the documentation you currently have at your github repo under your readme file.
Please edit your post, and adjust the category of your contribution to move to the documentation category. Also please include a link to the commit of your most recent documentation update along with the official github repo link within the post content.
See the Utopian Rules.
We would gladly then approve your post. And nice work btw on this project :)
You can contact us on Discord.
[utopian-moderator]
Thanks for the feedback @mcfarhat - Just let me know if the article is fine now :)
Thank you, your contribution has been accepted
Your contribution has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks a lot 😊👍
this post under review again
sorry for the mistake
[utopian-moderator]
No problem at all :) Just let me know if there is something I can do :)