This is the final 1.0.0 release of SteemJ-Image-Upload that heavily improves the code quality.
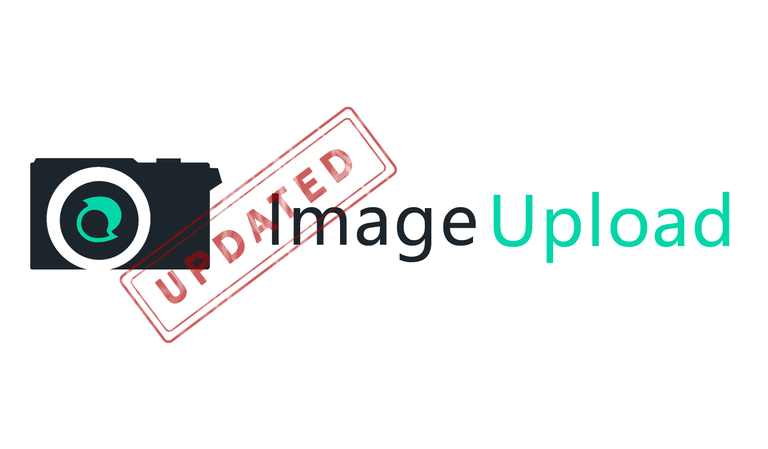
SteemJ-Image-Upload 1.0.0 has been released ~ Upload Images to steemitimages.com out of your Java Application
Hello Steemians!
about three weeks ago I've decided to make Steem-Image-Upload public and released a first pre-release to collect feedback from you guys (checkout the announcement post). So far, I've only received positive feedback and in addition testers like @paatrick mentioned that everything is working fine. Thanks for that! =)
While everything seems to work perfectly, I still had some code quality related tasks on my side. In example:
- The whole project had 0 tests and no documentation at all
- It supported no possibility to debug it as 0 logs were available
- It was pretty heavy as it was based on big libraries while only using a small part of them
This release solves all those issues so that I feel more than confident to give this version the number 1.0.0 which makes SteemJ-Image-Upload my first Steem related tool that I would call "finished".
Changelog for version 1.0.0
- Issue #01 - Use crypto-core instead of bitcoinj to reduce the size of the project
- Issue #02 - Add timeout configuration to the Config object
- Issue #04 - Add a logging framework and implement proper logging
- Issue #05 - Add, implement and use proper Exceptions
- Issue #06 - Update dependencies
- Issue #07 - Add a proper copyright header
- Issue #08 - Add a license
- Issue (PR) #09 - Create LICENSE
- Issue #10 - Do not depend on SteemJ
- Issue #11 - Secure the functionality with tests
Changes in Detail
The only change that will effect you, as a user, is the addition of a logging framework. Based on the experience made with SteemJ I only added the slf4j-api to the project. This gives you all the flexibility to choose the implementation on your own, so if you have an Android project, you can choose a logging framework that will actually work there, while you can still add common logging implementations like log4j2 if you develop a common desktop or server application.
If no slf4j-implementation is added at all, you will face the following WARNING messages at start up if no implementation has been provided:
SLF4J: Failed to load class "org.slf4j.impl.StaticLoggerBinder".
SLF4J: Defaulting to no-operation (NOP) logger implementation
SLF4J: See http://www.slf4j.org/codes.html#StaticLoggerBinder for further details.
Adding a slf4j-implementation to your project
One good example of an alternative slf4j implementation is log4j-slf4j-impl, which implements the slf4j-api and adds the powerfull configuration options of log4j2.
As a first step, the log4j-slf4j-impl jar needs to be added to your project. The most easiest way to achieve this is the usage of a Build-Management tool like Maven, because you only need to add the required dependencies the pom.xml.
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-slf4j-impl</artifactId>
<version>${log4j.version}</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>${log4j.version}</version>
</dependency>
To finalize the logging configuration of your project, create a log4j2.xml and add it to the src/main/resources folder. An example log4j2.xml configuration is shown below.
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="INFO">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n" />
</Console>
</Appenders>
<Loggers>
<Logger name="eu.bittrade.libs.steemj.image.upload" level="WARN">
<AppenderRef ref="Console" />
</Logger>
<Logger name="my.project" level="DEBUG">
<AppenderRef ref="Console" />
</Logger>
<Root level="ERROR" />
</Loggers>
</Configuration>
The configuration above would append all log messages of:
- SteemJ-Image-Upload, that have a severity of WARN or higher
- your project (package my.project), that have a severity of DEBUG or higher
to the console, while ignoring all other log messages, because no logger is defined for Root.
If you want to use log4j2 in your project too, you should have a look at the official documentation to find detailed configuration examples.
General Project information
What is SteemJ-Image-Upload?
SteemJ-Image-Upload is a sub-project of SteemJ - The Steem API for Java.
SteemJ Image Upload allows to upload images to (https://steemitimages.com) out of your Java application.
The project has been initialized by dez1337 on steemit.com.
Technologies
- Java 7
- Maven 3+ for building the project
- Crypto-Core to sign the image with your private posting key
- Google HTTP Client
- SLF4J
- WireMock to simulate a steemitimages.com response during test execution
Quick start guide
Using SteemJ-Image-Upload is really easy: Add it to your project and upload your first image as shown below.
Add SteemJ-Image-Upload to your project
SteemJ Image Upload binaries are pushed into the maven central repository and can be integrated with a bunch of build management tools like Maven.
File: pom.xml
<dependency>
<groupId>eu.bittrade.libs</groupId>
<artifactId>steemj-image-upload</artifactId>
<version>1.0.0</version>
</dependency>
Write a Java Class to upload an Image
SteemJ Image Upload is really easy to use. The following code shows a full sample:
import java.io.File;
import java.io.IOException;
import java.net.URL;
import eu.bittrade.libs.steemj.base.models.AccountName;
import eu.bittrade.libs.steemj.image.upload.SteemJImageUpload;
public class ExampleApplication {
public static void main(String args[]) {
AccountName myAccount = new AccountName("dez1337");
// Please be informed that this is not the correct private key of dez1337.
String myPrivatePostingKeyAsWif = "5KMamixsFoUkdlz7sNG4RsyaKQyJMBBqrdT6y54qr4cdVhU9rz7";
File imageToUpload = new File("C:/Users/dez1337/Desktop/SteemJImageBanner.png");
try {
URL myImageUrl = SteemJImageUpload.uploadImage(myAccount, myPrivatePostingKeyAsWif, imageToUpload);
System.out.println("The image has been uploaded and is reachable using the URL: " + myImageUrl);
} catch (IOException e) {
System.out.println("There was a problem uploading the image.");
e.printStackTrace();
}
}
}
An output of the code above will look like this:
The image has been uploaded and is reachable using the URL: https://steemitimages.com/DQmfWn1z6HwwxfaWhrpY28MYh43wEEyNsV25GnGSGZUDo2U/SteemJImageSteemFriendlyWithUpdatetBanner.png
As you can see in the sample above, your account name and your private posting are required to upload an image - If you do not provide those details, your upload request will not be accepted. For sure, SteemJ-Image-Upload will not send or store your private posting key!
If you have security doubts about it you can:
- Check the official imagehoster README file
- Review the SteemJ-Image-Upload source code at GitHub.
Bugs reports, feedback and discussions
For bugs or feature requests please create a GitHub Issue.
For general discussions or questions you can also:
- Post your questions in the Discord Java Channel
- Reply to one of the Crypto Core update posts on Steemit.com
- Contact me on steemit.chat
Contribute
Bug reports and feature requests already help a lot to improve the project. What helps even more is an active contribution, so if you see something to improve, I would love to see a pull request from you.
Get in touch!
Most of my projects are pretty time consuming and I always try to provide some useful stuff to the community. What keeps me going for that is your feedback and your support. For that reason I would love to get some Feedback from you <3. Just contact me here on Steemit or ping me on GitHub.
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thank you for your great job in contributing to the experience of steemit. It is people like you who can help steemit to keep progressing. I am new here and I appreciate every info and idea.
Thank you for your nice words :)
Your contribution cannot be approved yet because it does not have proof of work because the development category rules state that if your username on Github does not correspond to the Utopian username you must provide proof that you are the owner by providing a screenshot of the logged in session in Github. Also you need to remove the resteem part from the post.
See the Utopian Rules. Please edit your contribution and add proof (links, screenshots, commits, etc) of your work, to reapply for approval.
You may edit your post here, as shown below:
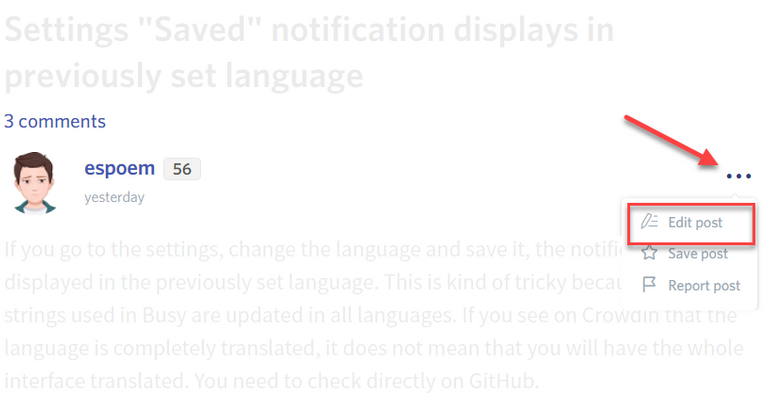
You can contact us on Discord.
[utopian-moderator]
Hay @codingdefined and thank you for your accurate report.
Here is a screenshot where I am logged in to GitHub:
marvin-we/dez1337:
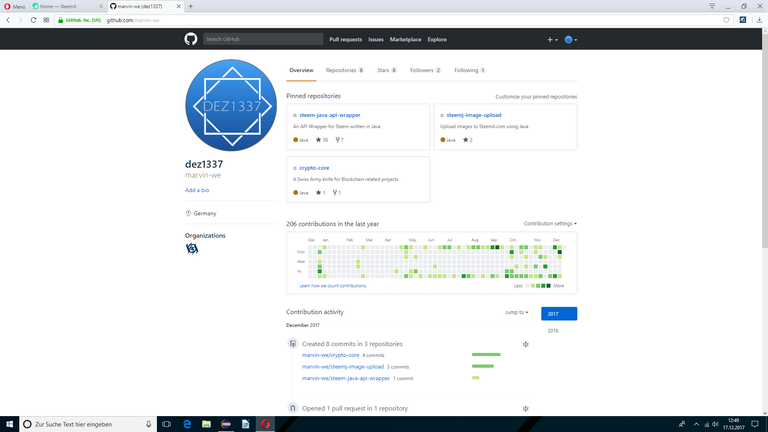
neutralleiter:
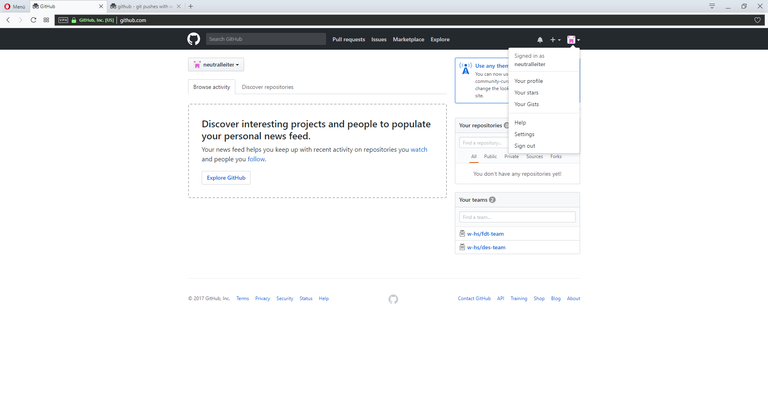
Beside that the projects are already linked with Utopian :) Please let me know if this is sufficient now.
Best regards!
Hey @dez1337 I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Good post.
Follow and upvote me https://steemit.com/@devart/
Thanks buddy.