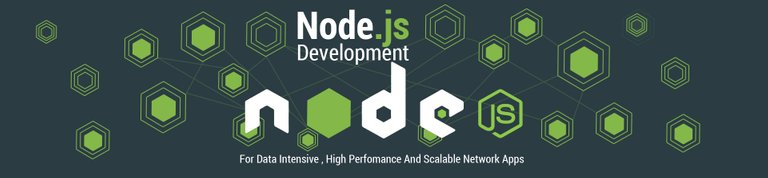
Repository
https://github.com/feekayo/nodejs_tutorials
What will I learn
- How to set up NodeJS on your local device
- How to install ExpressJS and other modules
- How to handle HTTP Requests
- Modularizing your code
Requirements
- A Computer System running on Winodws, Linux or MacOS
- A Basic knowledge of Command Prompt/Terminal
- A Good Internet Connection
Difficulty
- Intermediate
Tutorial Content
Today, we're going to be kicking off our NodeJS journey from the scratch. In the previous tutorial posted; general feedback was that we tried to pass too much information at once. This led to the tutorial being too confusing for a novel learner.
So in trying to avoid making this mistake again, we would be taking you on a carefully crafted journey through the stages from setting up nodejs on your computer system to deploying your first application
Let's get started.
Installing NodeJS
Let us start our NodeJS journey with NodeJS installation. Now if you already have NodeJS installed on your Computer, you can skip this stage of the Tutorial, otherwise proceed.
Now Open up your Command Prompt/ Terminal. If you're running a Ubuntu distribution of Linux. You can download NodeJS on your device by running the following line of code
apt-get install nodejs
Otherwise if you are running on a Windows or MacOS you can download the installation file from https://nodejs.org/download
step 1
step 2
step 3
You can proceed to follow the installation wizard. With the installer comes the NodeJS runtime, the npm package manager
and the option to add both of them to the Operating System PATH
After a succesful installation, you should have node set as a PATH variable. You can test whether node was successfully installed and set using was successful by running the following line
node ---version
Ubuntu users who perform the installation via package will need to install the NPM package manager seperately. Using the following line on terminal
apt-get npm install
To set npm as a path variable on your Ubuntu device, run the following command
export PATH=$PATH:/path/to/npm
For the above you would need to find the path to npm installed on your local device.
Installing the ExpressJS module
NodeJS is heavily modular, and its core capabilities are often stretched and extended using certain packages and modules.
One of such modules is ExpressJS. Which is one of the most commonly used and most powerful frameworks built on top of NodeJS. It is a flexible web application framework that provides a rich array of methods and interfaces for developing single or multipaged applications for the web. Read more here (https://expressjs.com).
To install ExpressJS. Run the following command
npm install express
How to Handle HTTP Requests
Most of the work we would be doing with RESTful APIs would be handling and playing with HTTP requests and their parameters. Thankfully this can be done easily in NodeJS using simple request handlers and NodeJS's http module.
To perform the task below, create a file in a directory of your choosing and name it http-request-handler.js then paste the code below
var http = require('http');//require NodeJS's http module
var port = 8000; //http port
function handle_requests(req, res) { //req=>request, res=>response
res.writeHead(200, { //only text content type would be returned
'Content-Type' : 'text/plain'
});
res.end('Hello World'); //send hello world response to the client
console.log('requested');
}
http.createServer(handle_requests).listen(port, '127.0.0.1');
console.log('Node.js http server started at http://127.0.0.1');
Inspecting the code, you should be easily able to decipher the functions and their meanings
- handle_requests:- Function for handling requests
- req:- Contains the request object
- res:- Contains the response object
- http.createServer:- Creates a server that handles requests
To add more functionality of the handle_requests function to be able to know what sort of request is being made, you can make a slight adjustment to the handle_requests function using the req.method object
function handle_requests(req, res) { //req=>request, res=>response
res.writeHead(200, { //only text content type would be returned
'Content-Type' : 'text/plain'
});
res.end(req.method+' request made'); //send hello world response to the client
}
req.method allows us to know what http verb was used to send the request to the server. For more on http verbs check the previous tutorial
To run the above file with NodeJS run the following on terminal/command prompt at the directory of the folder where your file is saved
node http-request-handler.js
To check whether the above works we would be using the postman http client which you should look to download.
The Results of the tests on postman client show the following
GET Request
POST Request
PUT Request
DELETE Request
Modularizing Your Code
NodeJS allows the seperation of code into a seperation of concerns. The above code snippets can be simplified further by splitting it into modules rather than just one module.
Looking at the above code we've written so far. There are two major parts of the code
- The part that creates the http server
- The part that handles the http requests
Now these two parts are intertwined. But that doesn't stop us from modularizing our code a little bit.
To do this should seperate the request handler from the http-module so we can then use the request handler to extend the functionality of the http-module.
To do this. Rename your current file server.js, and create a new file named request-handler.js; and save if in a folder named modules.
In this file. Save the following snippet
module.exports = {
handle_requests: function (req, res) { //req=>request, res=>response
res.writeHead(200, { //only text content type would be returned
'Content-Type' : 'text/plain'
});
res.end('Hello World'); //send hello world response to the client
console.log(req.method+' request made');
}
}
This file creates a user defined module that exports the handle_requests function to the outer components. The server.js file now looks like this
var requestHandlerModule = require('./modules/request-handler');
var http = require('http');
var port = 8000;
http.createServer(requestHandlerModule.handle_requests).listen(port, '127.0.0.1');
//pass handle_requests
By doing this, you can see how much simpler, gorgeous and explainable our program is. That is one of the major strengths of NodeJS. You would be seeing the power of this in later tutorials.
Running
node server.js
On your terminal gives the same results as when we ran
node http-request-handler.js
Earlier in the tutorial.
Conclusion
This is a soft introduction to Using NodeJS for writing RESTful APIs. We covered the basics of NodeJS.
From setting up your NodeJS environment to modularizing your code. We kept the tutorial short so as to avoid information overload and hope you enjoyed this tutorial. Thank you for following
Any questions would be entertained in the comment section.
Thank you for your contribution.
This moderation might not be considered due to the below:
Chat with us on Discord.
[utopian-moderator]Need help? Write a ticket on https://support.utopian.io/.