Repository
https://github.com/holgern/beem
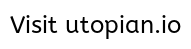
beem is a python library for steem. beem has now 481 unit tests and a coverage of 78 %. The current version is 0.19.33.
I created a discord channel for answering a question or discussing beem: https://discord.gg/4HM592V
Bug Fixes for Race condition in blockchain.blocks() when using threads
- The bug was described in detail here.
- Fix in commit 7c8b53
- The problem was the
auto_clean
function for the cache. - When using threads,
auto_clean
is now disabled withset_cache_auto_clean(False)
and the cache is manually cleaned withclear_cache_from_expired_items()
when all threads are finished.
Whenblocks()
finishes,set_cache_auto_clean(auto_clean)
is set to the old value.
SteemConnect integration
Inspired by steemconnect-python-client by @emrebeyler, SteemConnect is now integrated into beem.
I created @beem.app inside steemconnect for testing (https://v2.steemconnect.com/apps/).
The following steps were done to integrate steemconnect properly:
- A new Token class was added to beem/storage
- The following function were added to beem/wallet:
- setToken
- clear_local_token
- encrypt_token
- decrypt_token
- addToken
- getTokenForAccountName
- removeTokenFromPublicName
- getPublicNames
- a SteemConnect class was created (based on client.py from steemconnect-python-client)
- Integration into TransactionBuilder and Steem
You see a diff between both functions here (On the left side is client.py shown and on the right side steemconnect.py from beem). White ares indicate that both files are identically.
Usage
beem.steemconnect can be used to create steemconnect links for broadcasting transactions.
In the first example, nobroadcast=True
and unsigned=True
is used to disable signing and broadcasting. When then a broadcasting operation is called, the unsigned transaction is returned and entered into url_from_tx
. This function from SteemConnect
returns then a steemconnect v2 link which can be entered into the browser.
from beem import Steem
from beem.account import Account
from beem.steemconnect import SteemConnect
from pprint import pprint
steem = Steem(nobroadcast=True, unsigned=True)
sc2 = SteemConnect(steem_instance=steem)
acc = Account("test", steem_instance=steem)
pprint(sc2.url_from_tx(acc.transfer("test1", 1, "STEEM", "test")))
results in:
'https://v2.steemconnect.com/sign/transfer?from=test&to=test1&amount=1.000+STEEM&memo=test'
It is also possible to create a operation by hand:
from beem import Steem
from beem.transactionbuilder import TransactionBuilder
from beembase import operations
from beem.steemconnect import SteemConnect
from pprint import pprint
stm = Steem()
sc2 = SteemConnect(steem_instance=stm)
tx = TransactionBuilder(steem_instance=stm)
op = operations.Transfer(**{"from": 'test',
"to": 'test1',
"amount": '1.000 STEEM',
"memo": 'test'})
tx.appendOps(op)
pprint(sc2.url_from_tx(tx.json()))
results in:
'https://v2.steemconnect.com/sign/transfer?from=test&to=test1&amount=1.000+STEEM&memo=test'
Creating SteemConnect links with beempy
By adding -l
as option, all available broadcast operation can be converted into a link:
beempy -l claimreward holger80
results in
"https://v2.steemconnect.com/sign/claim_reward_balance?account=holger80&reward_steem=0.048+STEEM&reward_sbd=3.842+SBD&reward_vests=5093.882902+VESTS"
Fetching a steemconnect app token
In examples/login_app, an example app.py can be found. This example was adapted from steemconnect-python-client. Set the client_id
, the scope
and unlocking the wallet by entering the correct passphrase.
The flask app can be started by
flask run
Enter http://127.0.0.1:5000/
in a browser and click on the link.
After entering a username and password, the generated token is stored in the wallet.
Using the app token for broadcasting via SteemConnect
from beem import Steem
from beem.steemconnect import SteemConnect
from beem.comment import Comment
sc2 = SteemConnect(client_id="beem.app")
steem = Steem(steemconnect=sc2)
steem.wallet.unlock("supersecret-passphrase")
post = Comment("author/permlink", steem_instance=steem)
post.upvote(voter="test") # replace "test" with your account
Using the app token for broadcasting via SteemConnect in beempy
By adding -s
as option, all broadcast operation as upvote are broadcast via Steemconnect
beempy -s upvote -a test -w 100 @author/permlink
beempy listtoken
This command can be used to show all stored token:
Commit history
Include steemconnect v2 to beem
steem
- add steemconnect in init, when set, steemconnect is used for broadasting
steemconnect
- new class can be used to broadcast operation with steemconnect v2
storage
- Token class to store encrypted token
Transactionbuilder
- use steemconnect broadcast with set in steem
Wallet
- add token storage class
- add setToken, clear_local_token, encrypt_token, decrypt_token, addToken, getTokenForAccountName, removeTokenFromPublicName, getPublicNames
Example
- Add login app for testing steemconnect
Fix issue #16
Several improvements and fixes
Account
- Example added for transfer
Nodelist
- Doc added
Steem
- steemconnect integration improved and use_sc2 added
- Docu improved
SteemConnect
- compatible with py2.7
- several improvements
- Examles added
Transactionbuilder
- Integration of steemconnect improved
Wallet - fix bug
SteemNodeRPC
- fix old and removed parameter n_urls
Doc
- Fix bug in tutorial page
Creating links for signing with SC2
- commit 249bdb4
- url_from_tx function added, which can be used to create url for signing with steemconnect v2
- Authomatic url creation removed
Add option to create url from all broadcast operation to beempy
CLI
- Add -l option to beempy
- Add -s option for broadcast via steemconnect
- addtoken, listtoken and deltoken added
- Add doc - files for nodelist, steemconnect and node
Storage
- sc2_scope removed from config
- hot_sign_redirect_uri added to config
Some improvements for steemconnect
- commit e21be76
- Converts amounts from list to string (appbase)
- Unit test for steemconnect added
Transactionbuilder
- Remove log line
Hi there,
Great updates, again.
Oh, kudos to bug reporter. :)
Btw, did you consider implementing a semaphore here so only one thread runs clear_expired_items at once? Or does it sound like an overkill?
Thanks for the mention. My username is @emrebeyler. Would be happy if you fix it.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thanks for reviewing. I was confused by your github name. I corrected your username in the post.
I will take a look into semaphores, thanks :).
Exellent post
Hey @holger80
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!