Repository
https://github.com/dmlloyd/openjdk
What Will I Learn?
- You will learn how to conencting two computer.
- You will learn how to create peer to peer connection.
- You will learn how to implement java networking.
Requirements
- you must understand basic of java programming
- you must know about socket programming
- you must know defination about peer to peer networking.
Difficulty
- Basic
Tutorial Contents
okay, on this occasion I will share a tutorial how to make a connection between two computers using a peer to peer network, the first step we do is to create a program for the server computer, after that we will create a program for the client computer. we will determine the server and port number to match.
Okay, we just started the tutorial.
- the first step you have to do is provide two computers to connect to, if you don't have two computers, you can use VMware instead.
- then open the NetBeans application on one computer and create a new java file.
- then type the program below for the first computer or server computer.
package clientserver;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import javax.swing.JOptionPane;
public class Server {
public static void main(String[] args) throws IOException{
int Port =Integer.parseInt(JOptionPane.showInputDialog("Input Your Port : "));
String IP = JOptionPane.showInputDialog("Input Your IP Server : ");
ServerSocket serverSock=new ServerSocket(6066);
Socket Sock=serverSock .accept();
DataOutputStream out =new DataOutputStream(Sock.getOutputStream());
out.writeUTF("i am fine, thank you");
DataInputStream in= new DataInputStream(Sock.getInputStream());
System.out.println(in.readUTF());
Sock.close();
}
}
- okay, i will explain a little about the program above
import java.io.DataInputStream;
This program is a program that functions for input types with Stream typesimport java.io.DataOutputStream;
this is also Stream type, but this is for the output section.import java.io.IOException;
this is a class that functions to handle the two previous classes, namely input and output.import java.net.ServerSocket;
this is a class that works to run a server.import java.net.Socket;
this is a class that must exist on each socket programming.import javax.swing.JOptionPane;
this is the class we use if we implement the JOptionpane input method.public class Server {
this is a class name declarationpublic static void main(String[] args) throws IOException{
this is the main function that must be present in every java programming.int Port =Integer.parseInt(JOptionPane.showInputDialog("Input Your Port : "));
this is a declaration of port variables with integer data types.String IP = JOptionPane.showInputDialog("Input Your IP Server : ");
this is a declaration of IP variables with varchar data types.ServerSocket serverSock=new ServerSocket(6066);
this is the determination of the port number that is = 6066Socket Sock=serverSock .accept();
this is the creation of an accept action if the client computer with the same port number.out.writeUTF("connecting succes");
this is a notification if both computers are successfully connected.
- Okay, for the first program it's finished, then we will create a program for the second computer.
- create a new file for the second computer or client computer.
- then type the program below for client komputer
package clientserver;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.Socket;
import javax.swing.JOptionPane;
public class Client {
public static void main(String[] args)throws IOException {
int Port =Integer.parseInt(JOptionPane.showInputDialog("Input Your Port : "));
String IP = JOptionPane.showInputDialog("Input Your IP Server : ");
Socket sock=new Socket("localhost", 6066);
DataInputStream in= new DataInputStream(sock.getInputStream());
System.out.println(in.readUTF());
DataOutputStream out =new DataOutputStream(sock.getOutputStream());
out.writeUTF("waiting for connection");
sock.close();
}}
- actually the program is almost the same between the two computers, just different in a few parts.
- I don't need to explain about the second program because the syntax is the same as the first program.
- Okay, then we will run the program.
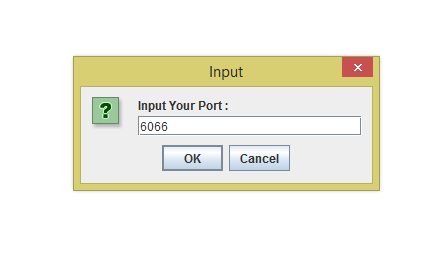
input port number
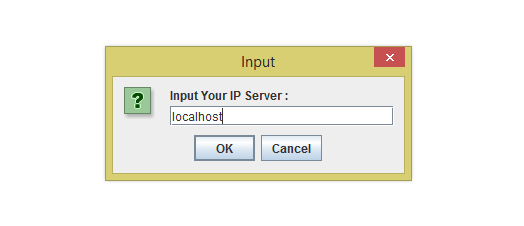
input your IP
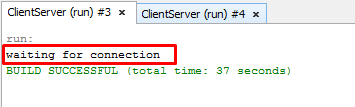
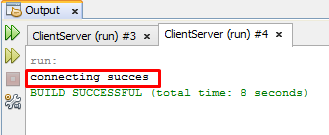
OK, the program is finished, the two computers can be connected to each other through the peer to peer network
Curriculum
- Java Tutorial: How To Create Auto Messaging (BOT MESSAGE) With Socket Programming On NetBeans
- Java Tutorial : How To Create java JFrame/GUI To Play Music On NetBeans
- Java Tutorial: How To Select And Send All File In One Folder Using "for" Looping Method On NetBeans
- HOW TO CREATE ACTION TO AUTO SELECT FILE USING "if" CONDITIONAL ON JAVA
- Java Tutorial: How To Create a Program To Send And Receive Files On NetBeans
Thank you for your contribution.
After reviewing your tutorial we suggest the following:
We recommend that you ident your code for better reading.
Nice work on the explanations of your code, although adding a bit more comments to the code can be helpful as well.
There are several tutorials that explain ServerSockets in java programming.
Try to come up with new and more innovative/useful ways to utilize Java.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 18 contributions. Keep up the good work!
Hi @lapulga!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @lapulga!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!