Repository
https://github.com/pars11/AndroidQuizApp
What is the project about?
Android Quiz App is an quiz game for android. Dynamically as many categories and questions can be added, updated and deleted as desired. It currently uses Sqlite as the database.
When the game starts, the category selection screen appears. A new game is started with the questions in the category selected here. In each game questions are randomly brought.
When the game is over, a screen appears telling you how many questions you have made correctly and incorrectly and your score.
History
New Features
Play Quiz
- An XML layout
play_quiz_layout.xml
was designed with radio buttons to allow users select only one answer from multi-choice answers in a question. - An adapter class
PlayQuizAdapter.java
has the logic underlying the radio group. In this class, the option values of radio button are displayed with the help of the adapter class.
The following code snippet describes the whole logic of how the score was calculated.
holder.radioGroup.setOnCheckedChangeListener(new RadioGroup.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(RadioGroup group, int checkedId) {
radioButton = group.findViewById(checkedId);
String radioValue = radioButton.getText().toString().toLowerCase();
/* This sets each default value in the array e.g [0,0,0,0]
to 1 when a radiobutton is clicked
*/
answersQuestions.set(position, 1);
//This compares the answer from radiobutton with the answer in the database
if (radioValue.equals(data.getAnswerOoption())) {
if (selectedIds.get(position) != null){
if (!selectedIds.get(position).isCorrect){
correctScore += 1;
selectedIds.get(position).isCorrect = true;
}
} else {
AnswerModel model = new AnswerModel();
model.isCorrect = true;
model.id = group.getId();
selectedIds.set(position, model);
correctScore += 1;
}
} else {
if (selectedIds.get(position) != null){
if (selectedIds.get(position).isCorrect){
correctScore -= 1;
selectedIds.get(position).isCorrect = false;
}
} else {
AnswerModel model = new AnswerModel();
model.isCorrect = false;
model.id = group.getId();
selectedIds.set(position, model);
}
}
}
});
@Override
public int getItemCount() {
return questionList.size();
}
public int getCorrectScore() {
return correctScore;
}
public int getIncorrectScore() {
incorrectScore = questionList.size() - correctScore;
return incorrectScore;
}
void setValues(List<Question> values) {
questionList = values;
/**
* This gets the questions list size
* and generates a default array of integer
*/
int[] defaultValues = new int[questionList.size()];
for (int value : defaultValues) {
answersQuestions.add(value);
}
/**
* This generates a default array of AnswerModel with size() == questionList.size()
*/
for(int i = 0; i < questionList.size(); i++){
selectedIds.add(null);
}
notifyDataSetChanged();
}
- In the
PlayQuizFragment.java
class, this has aSubmit
button clicked to submit quiz and view score using a customized alert dialog. Also, the customized alert dialog has options of exiting the quiz or playing the game again.
The following code snippet shows the implementation:
@Override
public void onActivityCreated(@Nullable Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
category = getActivity().getIntent().getExtras().getString("category");
fab = getActivity().findViewById(R.id.fab);
submitButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//This checks the array of set integer if it contains 0
// e.g 0 means radiobutton(option) not selected
boolean isAllQuestionsAnswered = playQuizAdapter.answersQuestions.contains(0);
if (isAllQuestionsAnswered) {
Toast.makeText(getContext(), "You cannot leave any blank question", Toast.LENGTH_SHORT).show();
} else {
mPresenter.calculateScore();
}
}
});
}
@Override
public void showQuestions(List<Question> questions) {
if (questions.isEmpty()) {
showEmptyMessage();
fab.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent = new Intent(getContext(), AddQuestionActivity.class);
intent.putExtra("category", category);
startActivity(intent);
}
});
} else {
emptyTextView.setVisibility(View.GONE);
fab.setVisibility(View.GONE);
playQuizAdapter.setValues(questions);
}
}
@Override
public void showScore() {
//Create an alert dialog builder
AlertDialog.Builder builder = new AlertDialog.Builder(getContext());
//Set title value
builder.setPositiveButton("Play Again", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
startActivity(getActivity().getIntent());
}
})
.setNeutralButton("Exit", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
startActivity(new Intent(getContext(), CategoriesActivity.class));
}
});
//Get custom view
LayoutInflater inflater = getActivity().getLayoutInflater();
final View dialogView = inflater.inflate(R.layout.score_dialog, null);
builder.setView(dialogView);
final TextView correctView = dialogView.findViewById(R.id.correct);
final TextView incorrectView = dialogView.findViewById(R.id.incorrect);
correctView.setText(String.format(Locale.ENGLISH, "%s: %d", "Correct", playQuizAdapter.getCorrectScore()));
incorrectView.setText(String.format(Locale.ENGLISH, "%s: %d", "Incorrect", playQuizAdapter.getIncorrectScore()));
alertDialog = builder.create();
alertDialog.show();
}
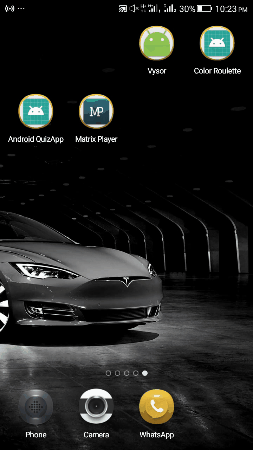
Important Resources
Roadmap
Play quiz per category and get resultShow questions per category- Update and delete category
- Update and delete question
Nice one.
Thanks
Thank you for your contribution. Instead of writing the whole code, try to summarize it little and also try to comment the code as much as possible.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Alright, thanks.
Hey @princessdharmy
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!