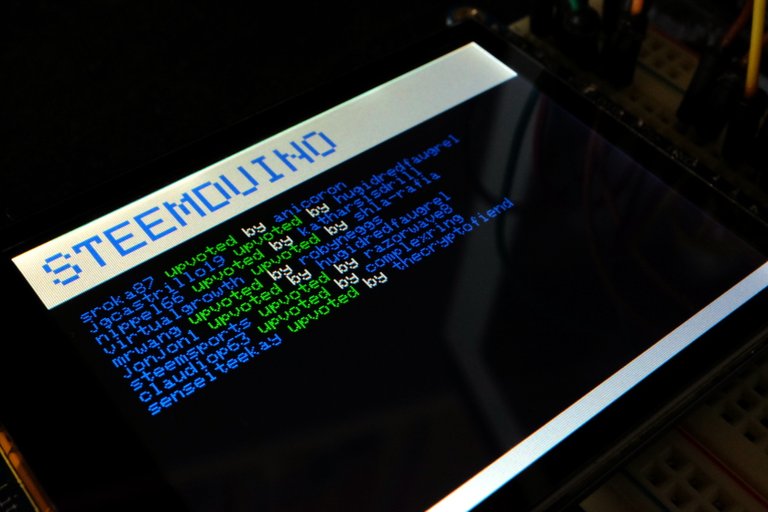
steemduino is an Arduino library for interacting with the steem websocket API. You can read more about it in [steemduino] - An Open Source Arduino Library for Steem.
The Adafruit 2.8 inch TFT LCD is a great little breakout board. It has a resolution of 320x240 pixels and comes in two flavours: one with a resistive touchscreen and the other with a capacitive touchscreen. It's a lot more flexible than basic two-row LCD screens, and with a touch screen, a lot more fun.
In this example, after connecting to the steem websocket, votes are listed on the screen. When the screen is full, it clears so more votes can be shown.
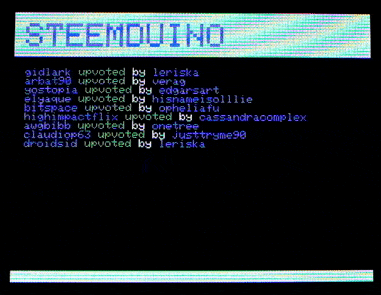
This is what that breakout board looks like when it's wired up:

Just follow the Wiring and Test instructions on the Adafruit website. It can be wired-up in either 8-bit or SPI mode. The example below is for 8-bit mode.
#include <Ethernet.h>
#include <SPI.h>
#include <Adafruit_GFX.h>
#include <Adafruit_TFTLCD.h>
#include <JsonStreamingParser.h>
#include <OpListener.h>
#include <SteemRpc.h>
#define LCD_CS A3 // Chip Select goes to Analog 3
#define LCD_CD A2 // Command/Data goes to Analog 2
#define LCD_WR A1 // LCD Write goes to Analog 1
#define LCD_RD A0 // LCD Read goes to Analog 0
#define LCD_RESET A4 // Can alternately just connect to Arduino's reset pin
#define BLACK 0x0000
#define BLUE 0x001F
#define RED 0xF800
#define GREEN 0x07E0
#define CYAN 0x07FF
#define MAGENTA 0xF81F
#define YELLOW 0xFFE0
#define WHITE 0xFFFF
Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET);
EthernetClient client = EthernetClient();
SteemRpc steem(client, "node.steem.ws", "/", 80);
unsigned long current_block = 0;
int lines = 21;
void op_handler(const String& op_type, const String& op_data) {
if (op_type != "vote")
return;
// Get the voter and author names. This is not even close to rhobust.
int voter_beg_pos = op_data.indexOf("voter") + 9;
int voter_end_pos = op_data.indexOf(',') - 1;
String voter = op_data.substring(voter_beg_pos, voter_end_pos);
int author_beg_pos = op_data.indexOf("author") + 10;
int author_end_pos = op_data.indexOf(',', author_beg_pos) - 1;
String author = op_data.substring(author_beg_pos, author_end_pos);
int permlink_beg_pos = op_data.indexOf("permlink") + 12;
int permlink_end_pos = op_data.indexOf(',', permlink_beg_pos) - 1;
String permlink = op_data.substring(permlink_beg_pos, permlink_end_pos);
int weight_beg_pos = op_data.indexOf("weight") + 10;
int weight_end_pos = op_data.indexOf(',', weight_beg_pos) - 1;
int weight = atoi(op_data.substring(weight_beg_pos, weight_end_pos).c_str());
if (lines == 21) {
tft.fillRect(0, 40, 320, 190, BLACK);
tft.setCursor(10, 50);
tft.setTextSize(1);
lines = 0;
} else {
tft.setCursor(10, tft.getCursorY());
lines += 1;
}
tft.setTextColor(BLUE);
tft.print(author);
if (weight > 0) {
tft.setTextColor(GREEN);
tft.print(" upvoted ");
} else if (weight < 0 ) {
tft.setTextColor(RED);
tft.print(" flagged ");
} else {
tft.setTextColor(CYAN);
tft.print(" unvoted ");
}
tft.setTextColor(WHITE);
tft.print("by ");
tft.setTextColor(BLUE);
tft.println(voter);
}
void setup(void) {
Serial.begin(9600);
Serial.println(F("TFT LCD test"));
Serial.print("TFT size is ");
Serial.print(tft.width());
Serial.print("x");
Serial.println(tft.height());
tft.reset();
uint16_t identifier = tft.readID();
if(identifier == 0x9325) {
Serial.println(F("Found ILI9325 LCD driver"));
} else if(identifier == 0x9328) {
Serial.println(F("Found ILI9328 LCD driver"));
} else if(identifier == 0x7575) {
Serial.println(F("Found HX8347G LCD driver"));
} else if(identifier == 0x9341) {
Serial.println(F("Found ILI9341 LCD driver"));
} else if(identifier == 0x8357) {
Serial.println(F("Found HX8357D LCD driver"));
} else {
Serial.print(F("Unknown LCD driver chip: "));
Serial.println(identifier, HEX);
Serial.println(F("If using the Adafruit 2.8\" TFT Arduino shield, the line:"));
Serial.println(F(" #define USE_ADAFRUIT_SHIELD_PINOUT"));
Serial.println(F("should appear in the library header (Adafruit_TFT.h)."));
Serial.println(F("If using the breakout board, it should NOT be #defined!"));
Serial.println(F("Also if using the breakout, double-check that all wiring"));
Serial.println(F("matches the tutorial."));
return;
}
tft.begin(identifier);
tft.fillScreen(WHITE);
tft.setRotation(1);
tft.setTextColor(BLUE);
tft.setTextSize(3);
tft.setCursor(10, 10);
tft.println("STEEMDUINO");
tft.fillRect(0, 40, 320, 190, BLACK);
tft.setCursor(10, 50);
tft.setTextSize(1);
tft.setTextColor(WHITE);
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
Ethernet.begin(mac);
tft.setTextColor(WHITE);
tft.setCursor(10, tft.getCursorY());
tft.println("Connecting to steem...");
if (steem.connect()) {
tft.setTextColor(GREEN);
tft.setCursor(10, tft.getCursorY());
tft.println("Connected to steem");
delay(2000);
} else {
tft.setTextColor(RED);
tft.setCursor(10, tft.getCursorY());
tft.println("Failed to connect to steem");
while (1) {};
}
tft.reset();
}
void loop() {
// Hang if the client disconnects
if (!steem.is_connected()) {
Serial.println("Client disconnected");
while (1) {}
}
// Get the last block number
unsigned long last_block = steem.get_last_block_num();
if (current_block == 0) current_block = last_block;
while (current_block <= last_block) {
// Get the block
String block = "";
if (!steem.get_block(current_block, block)) {
Serial.print("Failed to get block ");
Serial.println(current_block);
continue;
}
// Parse the block
JsonStreamingParser parser;
OpListener listener(&op_handler);
parser.setListener(&listener);
const char* block_c = block.c_str();
for (size_t i = 0; i < strlen(block_c); ++i) {
parser.parse(block_c[i]);
}
current_block += 1;
}
delay(5000);
}
The example above and the rest of the library code can be found on GitHub. As the library develops I'll post more examples and as it becomes more stable, some tutorials.
This looks awesome, but just to be sure its clear - Is this your code/github and are the photos taken by yourself ?
All this post needs is image sourcing or a clarification you made this and I'll submit it to #steemtrail under the programming tag :)
Edit: Dug through your past posts, looks legit - awesome work :D
Glad you discovered that this is legitimate. For others reading this, the steemduino project is my own and the example was written for it.
Great that you've submitted the post to #steemtrail. Thanks! :)
Do you have any cool robots to share?
I'm afraid not. I'd be more interested in making a drone, but I'd need a 3d printer if I were to do it all myself. Would be fun.
For now I'm focusing on Steem applications. I have something nice in the works.
Sweet. Have you tried shapeways.com ? It's a great place for getting high quality 3d prints.
I've not done anything with 3d printing yet. I'm waiting for it to be a little cheaper.
good job
love arduino
what about rasb PI?
This library is only for the Arduino. Since the rPi runs a variant of linux, you can use the python-based libraries like piston and steemtools. I've not tried it, but I don't see why it wouldn't work.
very nice :)
Nice post! I voted for you.
Please follow me, I will follow you back! :)