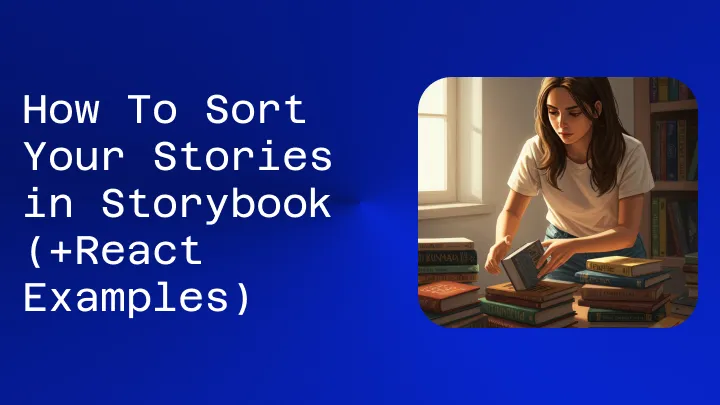
Storybook’s robust organization features let you categorize, search, and filter your stories, giving you the flexibility to tailor your workflow to your team’s specific needs.
Structure and hierarchy
You can organize your Storybook in two ways: implicitly or explicitly. Implicit organization uses the file system structure of your stories to determine their placement in the sidebar. Explicit organization uses the title parameter to define the story's location.
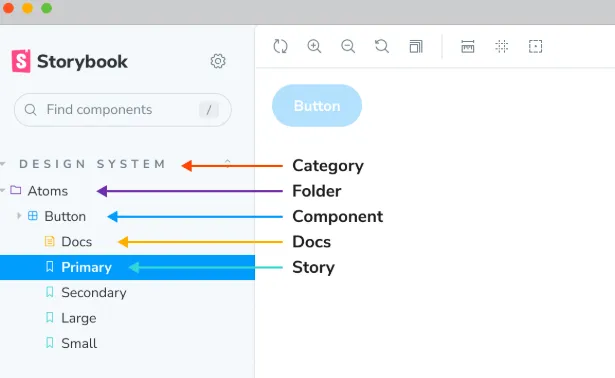
Storybook’s hierarchy is built from several parts, depending on how you organize your stories:
- Category: The highest level, grouping stories and documentation.
- Folder: A mid-level grouping within a category, often representing a feature or application section.
- Component: The lowest level, representing the component being tested.
- Docs: The auto-generated documentation for a component.
- Story: A specific test case for a component, showing a particular state.
Naming stories
The title parameter lets you explicitly control where a story appears in the Storybook sidebar. It also allows you to group related components within collapsible sections, making Storybook easier and more intuitive to navigate. For example:
// Replace your-framework with the name of your framework
import type { Meta } from '@storybook/your-framework';
import { Button } from './Button';
const meta: Meta<typeof Button> = {
/* 👇 The title prop is optional.
* See https://storybook.js.org/docs/configure/#configure-story-loading
* to learn how to generate automatic titles
*/
title: 'Button',
component: Button,
};
export default meta;
Yields this:
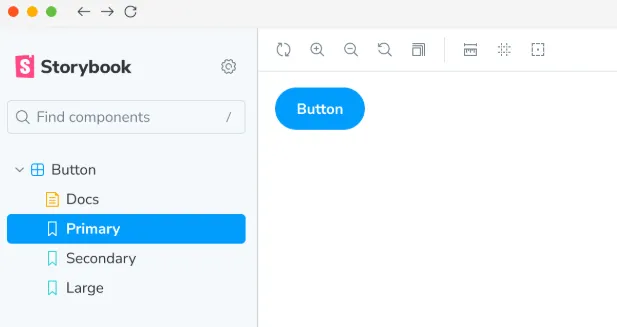
Grouping
You can also group related components in collapsible sections within Storybook by using the / character as a separator in your title.
// Replace your-framework with the name of your framework
import type { Meta } from '@storybook/your-framework';
import { Button } from './Button';
const meta: Meta<typeof Button> = {
/* 👇 The title prop is optional.
* See https://storybook.js.org/docs/configure/#configure-story-loading
* to learn how to generate automatic titles
*/
title: 'Design System/Atoms/Button',
component: Button,
};
export default meta;
// Replace your-framework with the name of your framework
import type { Meta } from '@storybook/your-framework';
import { CheckBox } from './Checkbox';
const meta: Meta<typeof CheckBox> = {
/* 👇 The title prop is optional.
* See https://storybook.js.org/docs/configure/#configure-story-loading
* to learn how to generate automatic titles
*/
title: 'Design System/Atoms/Checkbox',
component: CheckBox,
};
export default meta;
Yields this:
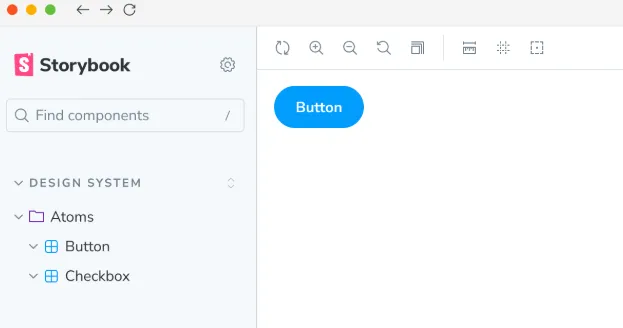
Roots
Storybook’s top-level groups are shown as non-expandable, uppercase items labeled “root” by default. You can disable this behavior in Storybook’s configuration. While this might create a cleaner look, for large Storybooks with many components, it’s generally better to name components according to your file structure for clear organization.
Single-story hoisting
If a component has only one story and its display name is identical to the component’s name (the last part of the title), that story will automatically replace the parent component's entry in the Storybook UI. For example:
// Replace your-framework with the name of your framework
import type { Meta, StoryObj } from '@storybook/your-framework';
import { Button as ButtonComponent } from './Button';
const meta: Meta<typeof ButtonComponent> = {
/* 👇 The title prop is optional.
* See https://storybook.js.org/docs/configure/#configure-story-loading
* to learn how to generate automatic titles
*/
title: 'Design System/Atoms/Button',
component: ButtonComponent,
};
export default meta;
type Story = StoryObj<typeof ButtonComponent>;
// This is the only named export in the file, and it matches the component name
export const Button: Story = {};
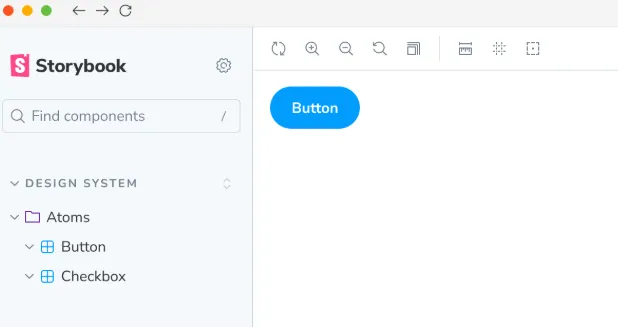
Story names are automatically converted to “start case” (e.g., myStory becomes "My Story"). For single-story components to be displayed correctly, either ensure your component name matches this start-cased story name, or explicitly set the story name using myStory.storyName = '...' to match the component name.
Controlling story display order
Improve Storybook navigation by customizing the order of your stories. Use the storySort option in preview.js to create a more user-friendly experience.
// Replace your-framework with the framework you are using (e.g., react, vue3)
import { Preview } from '@storybook/your-framework';
const preview: Preview = {
parameters: {
options: {
// The `a` and `b` arguments in this function have a type of `import('@storybook/types').IndexEntry`. Remember that the function is executed in a JavaScript environment, so use JSDoc for IntelliSense to introspect it.
storySort: (a, b) =>
a.id === b.id ? 0 : a.id.localeCompare(b.id, undefined, { numeric: true }),
},
},
};
export default preview;
Sort Storybook stories by title, name, or import path using the storySort function. Learn how to customize your story order beyond the default ID-based sorting.
Configuring Storybook story sorting with storySort
// Replace your-framework with the framework you are using (e.g., react, vue3)
import { Preview } from '@storybook/your-framework';
const preview: Preview = {
parameters: {
options: {
storySort: {
method: '',
order: [],
locales: '',
},
},
},
};
export default preview;
- Alphabetical Story Sorting in Storybook with storySort
- Manually Ordering Storybook Stories with storySort
- Filtering Storybook Stories with storySort by Name
- Localizing Storybook Story Sorting with storySort
Control the order of your Storybook stories. Sort alphabetically using method: 'alphabetical' and locales, or create a custom order with the order array. Nested arrays allow for fine-grained control over multi-level story organization.
// Replace your-framework with the framework you are using (e.g., react, vue3)
import { Preview } from '@storybook/your-framework';
const preview: Preview = {
parameters: {
options: {
storySort: {
order: ['Intro', 'Pages', ['Home', 'Login', 'Admin'], 'Components'],
},
},
},
};
export default preview;
Which would result in this story ordering:
- Intro and then Intro/* stories
- Pages story
- Pages/Home and Pages/Home/* stories
- Pages/Login and Pages/Login/* stories
- Pages/Admin and Pages/Admin/* stories
- Pages/* stories
- Components and Components/* stories
- All other stories
Place specific Storybook story categories at the end of the list using the * wildcard in the order array. This allows you to prioritize certain stories while keeping others grouped at the end.
// Replace your-framework with the framework you are using (e.g., react, vue3)
import { Preview } from '@storybook/your-framework';
const preview: Preview = {
parameters: {
options: {
storySort: {
order: ['Intro', 'Pages', ['Home', 'Login', 'Admin'], 'Components', '*', 'WIP'],
},
},
},
};
export default preview;
Use the * wildcard in Storybook's order array to place specific categories, like "WIP" (Work in Progress), at the end of your story list. Note: order sorting happens before alphabetical or default import-based sorting.
If you liked this content I’d appreciate an upvote or a comment. That helps me improve the quality of my posts as well as getting to know more about you, my dear reader.
Muchas gracias!
Follow me for more content like this.
X | PeakD | Rumble | YouTube | Linked In | GitHub | PayPal.me | Medium
Down below you can find other ways to tip my work.
BankTransfer: "710969000019398639", // CLABE
BAT: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
ETH: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875",
BTC: "33xxUWU5kjcPk1Kr9ucn9tQXd2DbQ1b9tE",
ADA: "addr1q9l3y73e82hhwfr49eu0fkjw34w9s406wnln7rk9m4ky5fag8akgnwf3y4r2uzqf00rw0pvsucql0pqkzag5n450facq8vwr5e",
DOT: "1rRDzfMLPi88RixTeVc2beA5h2Q3z1K1Uk3kqqyej7nWPNf",
DOGE: "DRph8GEwGccvBWCe4wEQsWsTvQvsEH4QKH",
DAI: "0x33CD7770d3235F97e5A8a96D5F21766DbB08c875"
Hello.
Welcome to Hive.
To confirm your authorship of the content, could you please add the word "Hive" to your LinkedIn profile?
https://www.linkedin.com/in/edwardcasanova/
You can remove this mention once we confirm the authorship.
Thank you.
More Info: Introducing Identity/Content Verification Reporting & Lookup
I'm not generating any of this via AI, the reason of my it looks like it is because I'm migrating from Medium to Hive! I want my content to be freely available to those who want to read it, PeakD seemed promising
Hello.
The content has traces of AI. Many AI-generated coding prompts (that look similar to these posts) have been used by spammers in the past.
The suspicion arose since many posts have been suddenly published into Hive within a few hours.
I will edit the comments for authorship verification.
@hivewatchers daaaa, I'm migrating from Medium lol, I want to backup my posts and also at the same time extend my reach to free users.
Isn't that what Blockchain is about, freedom and censorship resistance?
@hivewatchers done, check it out!