https://github.com/facebook/react
https://github.com/steemit/steem-js
Building a web app with React, Redux, and the Steem.js API, Part 1
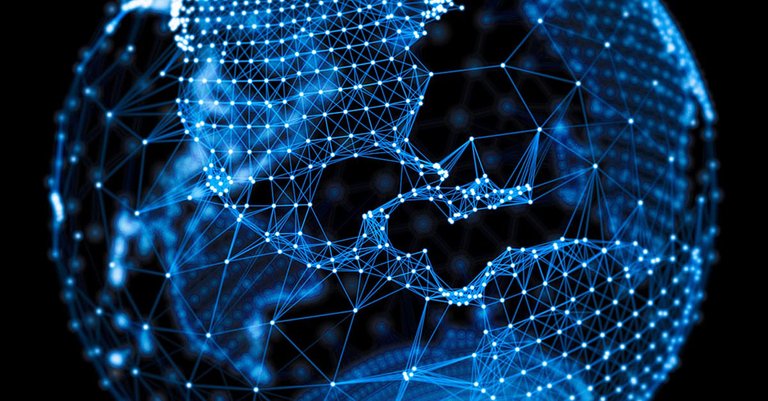
Image from mit.edu
Getting Started with the Steem.js API in a React project
I recently started poking around the Steem/Steemit documentation on Github and found several APIs made for interacting with the Steem blockchain. There are several APIs available in Javascript, Python, and Ruby and some very intensive documentation available on both Github and on the Steem website.
Over the course of my next few tutorials I will teach you how to programmatically communicate with the Steem blockchain via the Steem Javascript API in a React.js environment. Most of the Steem.js documentation is either outdated or works in a pure Node.js environment, and I have yet to find any documentation online that incorporates the Steem.js API in a React project. I hope to explore the API, integrate it into a React/Redux project, and build a cool little web app. This project will be a learning experience for both the reader and myself. Bear in mind that there isn't a whole lot of examples of apps working with the API in a React project and that I am learning as I go.
What you will learn in this tutorial series:
- Setting up a React project
- Using npm to install the Steem.js API
- Creating a basic React component with local state
- Making an API call to the Steem.js API
- Getting up and running with the Steem.js API in a React environment
Requirements:
- Understanding of Javascript/React basics
- Node.js
- Node Package Manager (npm)
- Some experience working with APIs
- Redux (later on in the series)
- And of course, the Steem.js API
Difficulty: Intermediate
Getting up and running with our React project:
In this first part of the tutorial we will get up and running with creating our React project using create-react-app, we will install the Steem.js dependency, and we will set up a basic React component that makes a call to the API and stores it in local state and can console.log the API data in the browser. Later on in this series we will incorporate Redux to the project and move away from using local state to manage the data, but for now let's start small and get comfortable making a call to the Steem.js API in a React environment.
Go over to the create-react-app Github page and follow the directions to get started. Install create-react-app by following the instructions on the Github page:
npx create-react-app my-app
cd my-app
npm start
Next navigate to the project folder and you can start the app by running npm start
. You should see the basic React project appear in the browser:
Basic React Project
Installing Steem.js in our project
Next, go to the Steem.js Github page and install the API.
npm install steem --save
This installs the Steem.js API and adds it to the package.json
file and allows us to work with it's functions in our project. Now we can get started with making API calls to the Steem blockchain, but first we need to do a few things.
First, import the Steem API into the project at the top of the app.js
file:
import steem from 'steem';
After that is done we can remove the logo imported at the top and all the boilerplate HTML that came with create-react-project. Our app.js
file should now look like this:
import React, { Component } from 'react';
import './App.css';
import steem from 'steem';
class App extends Component {
render() {
return (
<div className="App">
// Removed the boilerplate React HTML
</div>
);
}
}
export default App;
Adding state to app.js
Next let's add state to our component so we can store the data we are retrieving from the API. This is done by adding the following to our `app.js` component.
constructor(props) {
super(props);
this.state = {
userData: []
}
}
Using componentDidMount() to make the API request
Now that our component has state and has a place to store the data from the API we can get to the API request itself. To do this our project will use a React lifecycle method. In this case we will be using componentDidMount() to make our API call.
componentDidMount() {
// Retrieves and console.log data about the creators of Steemit
steem.api.getAccounts(['ned', 'dan'], (err, result) => {
console.log(err, result);
});
}
Inside the componentDidMount() function we call the Steem.js API and send out the request. In this example request from the documention we retrieve the Steemit profiles of the Steemit creators Ned Scott and Dan Larimer. I have taken the function and converted it to an ES6 arrow function.
The user data returned from the API request
If you run `npm start` and view the project in a browser and go to your browser's dev tools you should now see the data being retrieved in JSON format.

As you can see the request succcessfully returns the data and it is simple to request basic information about user accounts. Try playing with the request and changing the strings within the array in the steem.api.getAccounts
array to your own screen name and check out what it returns.
It should return a wealth of information within the JSON object like this:
Some of the properties in the returned user data
As you can see the API returns a lot of data pertaining to user profiles and there is a lot we could potentially do with this. Combine this with the hundreds of other functions in the API and it's not hard to see the potential for projects you could build using Steem.js. Functions from the API cover everything from trending categories, topic tags, retrieving user comments and followers, and even topics pertaining to the economics of Steemit such as escrow, votes, payout, etc...
Conclusion of Part 1
In the coming years and with the growth of Steemit.com and Busy.org and the Steem blockchain it wouldn't surprise me if social media sites all moved away from proprietary data and turned towards new solutions like blockchain based sites. APIs like Steem.js should lead to the growth in the number of front-ends for the Steem blockchain and hopefully developers will be creating new and exciting interfaces using these APIs.
I'm going to keep this first part of the series short and sweet. I hope this tutorial was concise and clear and that you got a taste of how to work with the Steem.js API in a React project.
In future tutorials I will be covering more advanced topics and we will be building a small app and incorporating Redux.
Nice introductory tutorial @nicknyr. Hope to see something more creative than simply querying the API in the next tutorials from you. There are already tutorials about React and SteemJs available, I would suggest you to keep each tutorial such that it produces some end product, for example you could have added some value to the tutorial by showing the response data in a react component on the web browser.
Keep contributing to the open source community!
Yeah I hear ya. Just wanted to start small. Some of my past tutorials were criticized for being too long. The next tutorial is going to show React components with the data displayed.
Hey @aneilpatel
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
I thank you for your contribution. Here are my thoughts on your post;
As @aneilpatel stated, it would be nice to see creative techniques. You achieved your curriculum's main goal as it was using steem.js in React. So, instead of showing every function of steem.js, you can show creative techniques which are special to React and can be used with steem.js.
Explaining the functions those special to React would be nice. For example, you could explain what componentDidMount does by 2 sentences. I don't want you to write down the whole documentation here as a filler content. But, quick explanations would be nice. Be consider them next time, please.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @yokunjon
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hey @nicknyr
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!