Interface in Java is similar to the abstract class where you can't instantiate the class i.e. create the objects of the class. The following are the features of the interface in java:
- All the methods within interface block are by default
public
andabstract
. - In order for the interface method to work, another class needs to implement that interface using
interface
keywords.
This is a simple program demonstrating the use of interface
in java.
interface A{
void output();
}
class B implements A{
public void output() {
System.out.println("Printing output for Interface Class");
}
}
public class InterfaceClass {
public static void main(String[] args) {
A a1=new B();
a1.output();
}
}
The output of this code is as follows:
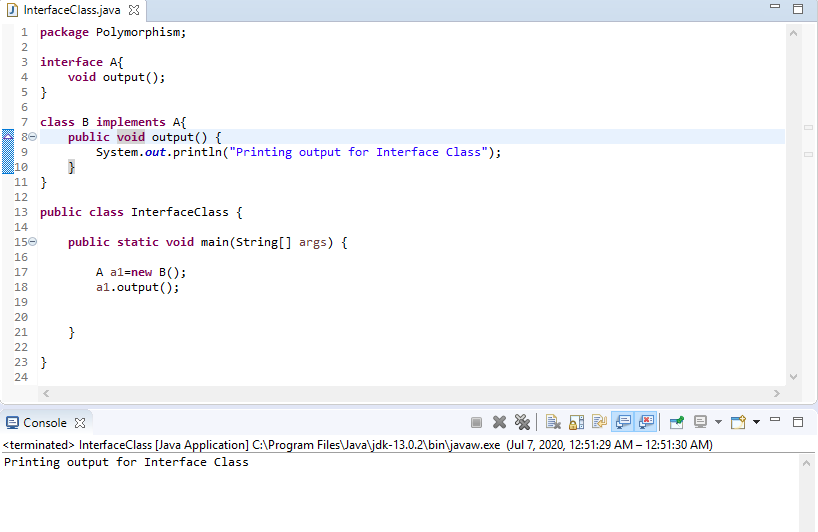