In order to allow Utopian contributors to design LHC analysis codes that could be helpful to particle physics, it is first necessary to explain what one detects in a collider experiment such as those ongoing at the Large Hadron Collider (the LHC) at CERN. In addition, it is also needed to be able to link those detectable physics objects to the manner to implement and use them in the MadAnalysis 5 platform that we plan to use (that is also mirrored on GitHub).
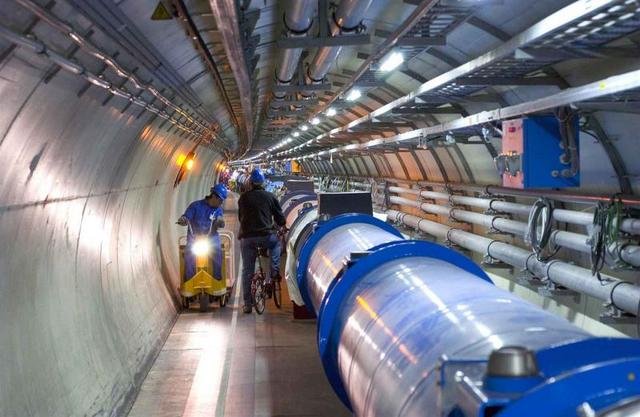
[image credits: CERN]
This post thus consists in the step 1a of the roadmap sketched here.
I decided to split the initial step 1 into several parts, as I prefer to move slowly and strongly instead of quickly and unreliably.
Each post will hence go straight to one specific point instead of plenty of them. I want to somehow make sure that everyone follows.
They are several classes of objects that are reconstructed in a typical LHC detector. They are classified through six containers in the MadAnalysis 5 framework (we will focus on only three of them today).
The handling of those objects is crucial for the rest of this project, and I will therefore propose a series of programming exercises accompanying my posts to facilitate their usage (in a way in which one understands what one is doing). I believe those exercises are important for a better handling of the next more complicated (at least from the physics standpoint) steps.
I invite all the participants to publish the pieces of code solving the exercises on Utopian. Please make sure to tag me either directly on the post or on discord. My discord username is lemouth#8260, and I can be found both on the steemstem and utopian discord servers. Without such a mention, I may just miss your post :/
I am obviously available for questions (both on discord and in the comment section of this article).
PARTICLE DETECTION AT THE LHC IN A NUTSHELL
In a particle collision such as those on-going at the LHC, many particles are produced.
Some of them can reach the material of the detectors unaltered, so that they can interact with it. This leaves signals that allow physicists to infer the presence of these particles (through an often complex electronic apparatus). This is what we call stable particles, as they are stable on detector time scales.
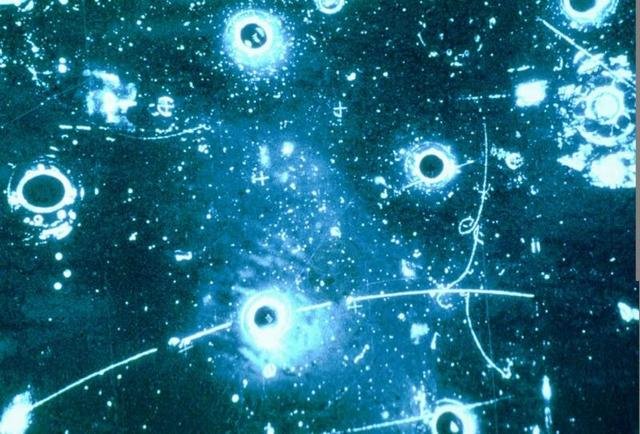
[image credits: CERN]
The second class of objects that can be reconstructed in a detector are what we call jets of strongly-interacting particles, or simply jets.
The strong interaction is one of the three fundamental interactions and it plays a key role at the LHC. It indeed governs the LHC collision processes from almost A to Z.
Of course, we are here more interested in the Z side, that tells us that any produced strongly-interacting particle always leads to a jet made of many of them.
I will skip any extra detail for today; I hope to have been clear enough in a small amount of words. But please do not worry, I will come back to this very soon.
The last class of objects that can be reconstructed in a detector consists of unstable particles. As their name suggest it, those particles decay, almost instantaneously and through a sometimes complicated process, into stable particles and/or jets.
Therefore, one cannot detect them, and only the analysis of the properties of the observed final-state particles allows to infer their initial presence.
And of course, we are also capable to detect the invisible… This may be seen as a fourth class of observable objects (yes, really!) in the detector records of any LHC collision: the missing energy.
THE STRUCTURE OF A MADANALYSIS 5 ANALYSIS
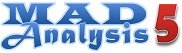
[image credits: MadAnalsysis 5]
It is now time to make a parallel with the MadAnalysis 5 framework.
Instead of providing a full manual that one may have forgotten before starting anything more concrete, I will instead be practical and proceed with a series of well defined exercises, closely linking physics to coding.
One given collision recorded in a detector is called an event. Correspondingly, the corresponding object in the MadAnalysis 5 framework is called event
.
The LHC analyses that will be implemented within this Utopian project hence consist in C++ codes allowing to process the information included in an entire sample of event
objects, representing what could really happen in thousands of LHC collisions.
In practice, the task that will be assigned to the contributors will be to compute quantities that will allow one to make decisions about how to treat an event. One indeed needs to decide if one needs to reject an event (because it is found boring) or to keep it for further investigations (as a source of a potentially interesting phenomenon).
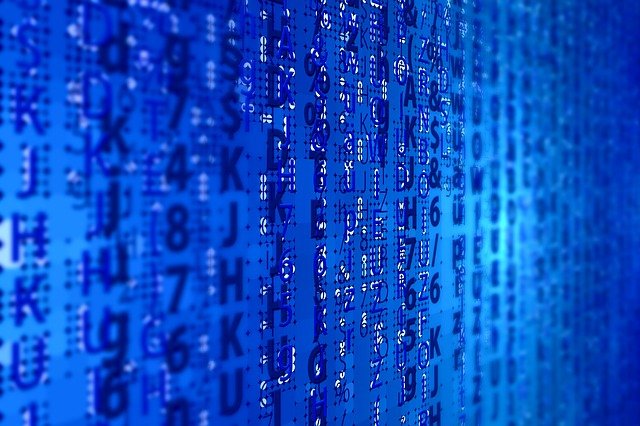
[image credits: Pixabay (CC0)]
In order to start, one should go to the directory in which MadAnalysis 5 has been installed (see here for the installation instructions) and then type, in a shell,
./bin/ma5 -E test_folder test_analysis
This leads to the creation of a folder named test_folder
in which we will code a toy analysis (i.e. the exercise to be done in this post).
The file to modify to this end is
test_folder/Build/SampleAnalyzer/User/Analyzer/test_analysis.cpp
The code inside it contains three methods named Initialize
, Execute
and Finalize
. In this post, we are only interested in modifying the Execute
method.
If one looks into that test_analysis.cpp
file and in the template for the Execute
method, one can observe that it takes an event
object in argument,
bool test_analysis::Execute(SampleFormat& sample, const EventFormat& event)
{
…
}
One will hence have to implement a code processing the attributes of this event
object.
STABLE PARTICLES
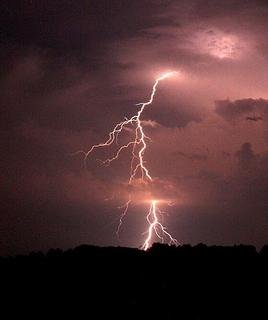
[image credits: Wikimedia]
The first category of particles that I want to focus on consists in the set of particles that are stable from the point of view of a typical LHC detector.
This means that once produced, these particles will just fly until they meet the material of the detector itself.
At that moment, they will interact with this material and leave tracks and/or energy deposits from which physicists will be able to deduce their presence and measure their properties.
There are three of these particles: electrons, muons and photons (as well as the associated antiparticles). In other words, these objects are observed as such and can be reconstructed (with a given efficiency) almost straightforwardly from all their hits in the detector.
All the physics objects that are reconstructed in an event can be accessed through the event
object attached to each collision event:
- electrons are stored in the
event.rec()->electrons()
container; - muons are stored in the
event.rec()->muons()
container; - photons are stored in the
event.rec()->photons()
container.
Kind of straightforward isn’t it? The three above C++ objects are vectors of special objects with given properties. The study of these properties will be the topic of the next post (this one is already way too long), as well as the investigation of any other type of objects recorded in a detector.
But in short, one can implement a loop over these vectors like for any c++ vector,
for(unsigned int ii=0; ii<event.rec()->electrons().size(); ii++)
{
…
}
THE EXERCISE
Let us first play a little bit with electrons, photons and muons. As I do no know the level of the participants to this project, I propose a super easy exercise. For more complicated things, please stay tuned! :)
Download a sample of 10 simulated LHC collisions from here. This file has been generated by means of the Delphes program that has been installed together with MadAnalysis 5, under the root format (also installed last week). I repeat that information on the installation procedure can be found here.
Edit the file
test_folder/Build/SampleAnalyzer/User/Analyzer/test_analysis.cpp
and implement a piece of code in theExecute
function allowing for the evaluation of the numbers of photons, electrons and muons present in each event. Following Utopian guidelines, I am expecting all codes to be stored on github.Compile the code and generate an executable by typing
cd test_folder/Build source setup.sh make
Create the (text) file
test_folder/Input/tth_aa.list
and indicate in it the absolute path to the downloaded event file (the location of thetth_aa.root
file on the local machine).Execute the code
cd test_folder/Build; ./MadAnalysis5job ../Input/tth_aa.list
Please provide the answer to the question raised in point 2.
Don’t hesitate to write a post presenting and detailing your code allowing to get to the answer. Please do not forget to mention me directly within your post, so that I could easily find it.
I also kindly ask to commit the c++ source file to this GitHub repository repository so that it would be easier for me to double check what has been done. Please follow the following syntax for the filename:
ex1a-<steemitID>.cpp
I fix the deadline to Wednesday May 30th, 16:00 UTC (I repeat, this exercise is easy). Then we will move on with the next step.
After this easy warm-up, things will get harder and more interesting next week! Remember, the goal is to implement a particle physics analysis such as those really performed at the LHC at CERN!
STEEMSTEM
SteemSTEM is a community-driven project that now runs on Steem for more than 1.5 year. We seek to build a community of science lovers and to make the Steem blockchain a better place for Science Technology Engineering and Mathematics (STEM).
More information can be found on the @steemstem blog, on our discord server and in our last project report. Please also have a look on this post for what concerns the building of our community.
Before anything else, thank you so much for contributing by sharing the series of posts and allowing developers to getting involved in such a great project. As I said before, I will take part in the process as long as I have time to work on.
Considering the post quality, the contribution is one of the best in the category, and it is staff picked because of the obvious reasons.
To assure the Utopian submission standards, I would like to see you edit the post for specifying the GitHub repository of the project. After creation of the mirror repository, I couldn't find the link to the repository in the post. If I am the one missing it, please do not mind this.
As my most important suggestion about the contribution, I think it would be really nice to see related posts within a section, containing series of links to the prior posts.
Your contribution has been evaluated according to Utopian rules and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post,Click here
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Hey @roj
Here's a tip for your valuable feedback! @Utopian-io loves and incentivises informative comments.
Contributing on Utopian
Learn how to contribute on our website.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thanks for the comment. I hope you will manage to free some time (at some point) :)
I added the two links roughly 6-7 hours ago. The mirror link is given in the first paragraph and the contributor link is given in the exercise section. You may just have missed them ;)
This is a good idea. I was in fact considering adding them, but then I forgot as several hours happened between the moment I started to write and the moment I finished the text... I will add all of this right now.
Thanks for the support in all cases :)
Thanks for the instructions @lemouth. I'll give it a shot shortly.
Just for clarity should we create our own individual project on GitHub for this exercise and future ones?
Also, I haven't used utopian before. Should we register this GitHub project on utopian?
Thanks.
I think this is the easiest thing to do. I could follow what contributors do on github, as well as on the blockchain through the associated posts that may be written.
On your personal side, you can create a github account and create one new project in there, that will contain your contribution to the MadAnalysis public analysis database. Since this corresponds to the only files you will modify (actually create) by yourself, it is fine (you will not have to touch madanalysis 5 itself, but only an external file that will be used by the platform for physics (if successfully coded at the end of the day).
I hope this clarifies :)
Hey @lemouth
Thanks for contributing on Utopian.
Congratulations! Your contribution was Staff Picked to receive a maximum vote for the blog category on Utopian for being of significant value to the project and the open source community.
We’re already looking forward to your next contribution!
Contributing on Utopian
Learn how to contribute on our website or by watching this tutorial on Youtube.
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Thanks for the support :)
I'm assuming you mean just the modifications to
test_analysis.cpp
and not the whole of Mad5, right?Exactly. There is no need to modify madanalysis 5 at all!
just now.....................................
Just now what?
Hi @lemouth! Loved your article of the step 1a about Particle physics @ Utopian - Detecting particles at colliders and implementing this on a computer.
Can you please explain me about > 'muons that are stored in event.rec()->muons() container'. I basically don't know about it and I will love to learn about it from you. :)
This container is a c++ vector. Each element of this vector is a muon. You can see this vector as the ensemble of the c++ representations of all the muons present in a simulated collision.
Does it clarify it?
Thanks for helping me out to know about this! :)
I got it now!! :)
You are welcome :)
Hello @lemouth
Many Commendations on this latest endeavor you are engineering to engender community interest in. You are such a great community builder. After months of dedicated work to popularize @steemstem and make it an undisputed destination for STEM enthusiasts, you are directing your attention to utopia to engineer a novel project for particle-physicists and programmers alike. This is worthy of commendation and community support to say the least.
I wish I were skilled in things of this nature, I would have been the first to partake in this educative exercise.
I pry you get massive support in this.
Thanks for being a true Steemian. The community appreciate you.
@eurogee of @euronation community
Thanks for this very nice message @eurogee! ;)
✌️
This sounds like electrons leaving their valence shell, not too much i learnt about LHC detector but i know the little knowledge i got from this article will play out well for me in the future. THANK YOU @lemouth
In this case, electrons are in fact produced not from atoms, but either from the annihilation or the decay of other particles. I hope this clarifies.
Une sacrée bonne idée et un bel exemple de démocratisation de la science rendu possible grâce à l'avancé des technologies. Le contre pied parfait à cette vague de désapprentissage des connaissances sévissant de nos jours en utilisant les mêmes armes, bravo!
Oui c'est aussi la conclusion a laquelle on est arrivee il y a 2 ans: afin de combattre les informations antiscience ou pseudoscience que l'on trouve partout (en particulier sur internet), il vaut mieux promouvoir et supporter le raisonnable afin de lui donner plus de visibilite. On est passe par le nombre dans tous les cas, donc ca ne peut etre que la seule chose a faire :)
This is just step 1a, and it's already filled with some much details and fun. Now my interest has been piqued to see more steps.
I believe this project will work out fine.
Big-up sire
Will you try it? :)
Sure I would like to try it.
I'm coupling the stages together. I've already bookmarked this post and the last one. So I can read them through again. To grasp more info.
First of all, I would start by running a linux emulator on my windows pc. Then I'll install the madanalysis.
Which Linux emulator would you recommend?
I don't know. I am not a windows user and I thus don't need any emulator. Please ask @effofex. He proposed something!
Okay. Thanks a lot for the kind response
I don't know. I am not a windows user and I thus don't need any emulator. Please ask @effofex. He proposed something!
Early draft is up at hackmd, haven't put it up on the blockchain yet since I am seeing it it's appropriate as a utopian.io tutorial first.
Also sent you a pull request with my edited cpp file.
I noticed :)
This statement
if (0 != event.mc())
is not necessary. You are not using at all the
event.mc()
properties. You may however want to add a protection (in case of an empty event record). In this case, you should use:if (event.rec()==0) return true;
Hope this helps!
Nice catch, that's what I get for looking at some of the example code in the comments.
At one point, I was calling
event.mc()->processId()
, so I could've justified it. Which leads me to a question, I was getting all 0's for processId, do events have unique identifiers?I've gotten it working for for Ubuntu 16 under the WSL for Win 10, but there's a few installation issues I am in the process of documenting.
If you want to get into it right away, running Ubuntu within VirtualBox is probably the easiest thing to do.
The app doesn't seem to work on mine. The PC I'm using currently is windows 7, which I have left like that for some reasons, but I think I might get an OS upgrade.
Thanks for your help
Yep, it's Win 10 only.
You may need to upgrade your windows version (or move to linux) :p
Sure I'll stick to this kind advice sir. Thanks
Now you can check here. The post is online :)
Okay. Thanks a lot
@lemouth wounder full contribution you have contributed by @utopian-io .
In the core of my heart your work is appropriate.
Thanks a lot for your hearty comment :)
J’espère qu’il va y avoir une traduction en Français pour bientôt. C’est trop technique pour moi et mon petit niveau d’anglais.
Malheureusement non, car ce projet est integralement en anglais. Mais je pourrai traduire le cours d'introduction a la physique des particules que j'ai ecrit pour Steem ici. Cela fait d'ailleurs longtepms que je n'ai pas poste en francais :)
Well written and explained @lemouth, more grease to your elbow for trying to educate people, really hope the Utopian community will appreciate your effort and contribute to make the community better
I really hope this project will work. This is step 1 (a). Let's see whether this will work. More from me in a week from now ;)
Thank you for the explanation, we often see the things physicist do on the particle colider, and what are their findings but we fail to realize the theory behind it
Physics consists in what lies behind the key principle of particle accelerators and searches at the LHC. A bunch of key ideas put all together and we can try to understand better how our universe works :)
This is becoming more Interesting, been following the article relating to this LHC. This was really quite explanatory and muxh easier to comprehend . I hope to understand better as times go on with your next post.
Great work @lemouth
Thanks! The next post will address the points mentioned here and still obscure. And of course include one more exercise ;)
Well, this is the beginning of a fantastic project. I'm happy I was here when it all started.
Let's see now how it will evolve :)
Well done @lemouth . This is a great project. I think it will go a long way in the history of particle physics. Meanwhile, I think I got something interesting in the initial part of the post. That is the different class of particles
I. Stable particles
II.Jets of strongly-interacting particles
III.Unstable particles.
IV.Detect the invisible
More strength.
Thanks! I will come back to the second, third and fourth category in the next post. Please stay tuned :)
Alright
Exercise done! Can't wait for more :)
I will have a look. This was an easy warmup :)
Particle Physics is a very interesting area where numerous studies are currently carried out. Excellent post @lemouth. Regards.
Yep, thousands of studies a year (I don't read all of them... :) )
Fabulous post @lemouth
I like the way you broke it down: i look forward to reading your next post
Thanks! :)
Exellent post
Source
Generic comments could be mistaken for spam.
More information:
The Art of Commenting
Comment Classifications
Excellent comment I would say...
love to read it about this.
I guess I should say "Thanks!". I am not sure to fully get the comment, sorry ;)
i support you man for your activity.
Thanks for your support!
Up lemouth!
Thank you!
Very nice ;-)
Indeed ^^